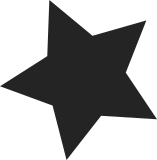
We currently install the default database by passing --user=mysql to the install script. With the upcoming bump to the 10.4 series, this does not work as intended. An error occurs because of missing PAM modules. We work around this now by creating the default db as root and calling chown to change the files to user mysql. Signed-off-by: Ryan Coe <bluemrp9@gmail.com> Signed-off-by: Peter Korsgaard <peter@korsgaard.com>
86 lines
1.6 KiB
Bash
86 lines
1.6 KiB
Bash
#!/bin/sh
|
|
#
|
|
# mysql
|
|
#
|
|
|
|
MYSQL_LIB="/var/lib/mysql"
|
|
MYSQL_RUN="/run/mysql"
|
|
MYSQL_PIDFILE="$MYSQL_RUN/mysqld.pid"
|
|
MYSQL_LOG="/var/log/mysql"
|
|
MYSQL_LOGFILE="$MYSQL_LOG/mysqld.log"
|
|
MYSQL_BIN="/usr/bin"
|
|
|
|
wait_for_ready() {
|
|
WAIT_DELAY=5
|
|
while [ $WAIT_DELAY -gt 0 ]; do
|
|
if $MYSQL_BIN/mysqladmin ping > /dev/null 2>&1; then
|
|
return 0
|
|
fi
|
|
sleep 1
|
|
: $((WAIT_DELAY -= 1))
|
|
done
|
|
return 1
|
|
}
|
|
|
|
start() {
|
|
# stderr is redirected to prevent a warning
|
|
# if mysql lib directory doesn't exist
|
|
if [ `ls -1 $MYSQL_LIB 2> /dev/null | wc -l` = 0 ] ; then
|
|
printf "Creating mysql system tables ... "
|
|
$MYSQL_BIN/mysql_install_db --basedir=/usr \
|
|
--datadir=$MYSQL_LIB > /dev/null 2>&1
|
|
if [ $? != 0 ]; then
|
|
echo "FAIL"
|
|
exit 1
|
|
fi
|
|
chown -R mysql:mysql $MYSQL_LIB
|
|
echo "OK"
|
|
fi
|
|
|
|
# mysqld runs as user mysql, but /run is only writable by root
|
|
# so create a subdirectory for mysql.
|
|
install -d -o mysql -g root -m 0755 $MYSQL_RUN
|
|
|
|
# Also create logging directory as user mysql.
|
|
install -d -o mysql -g root -m 0755 $MYSQL_LOG
|
|
|
|
# We don't use start-stop-daemon because mysqld has its own
|
|
# wrapper script.
|
|
printf "Starting mysql ... "
|
|
$MYSQL_BIN/mysqld_safe --pid-file=$MYSQL_PIDFILE --user=mysql \
|
|
--log-error=$MYSQL_LOGFILE > /dev/null 2>&1 &
|
|
wait_for_ready
|
|
[ $? = 0 ] && echo "OK" || echo "FAIL"
|
|
}
|
|
|
|
stop() {
|
|
printf "Stopping mysql ... "
|
|
if [ -f $MYSQL_PIDFILE ]; then
|
|
kill `cat $MYSQL_PIDFILE` > /dev/null 2>&1
|
|
[ $? = 0 ] && echo "OK" || echo "FAIL"
|
|
else
|
|
echo "FAIL"
|
|
fi
|
|
}
|
|
|
|
restart() {
|
|
stop
|
|
sleep 1
|
|
start
|
|
}
|
|
|
|
case "$1" in
|
|
start)
|
|
start
|
|
;;
|
|
stop)
|
|
stop
|
|
;;
|
|
restart)
|
|
restart
|
|
;;
|
|
*)
|
|
echo "Usage: $0 {start|stop|restart}"
|
|
;;
|
|
esac
|