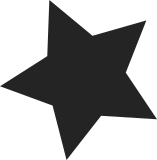
setlocalversion will use 'hg id' to determine whether or not the current revision is tagged. If there is no tag, the Mercurial revision is printed, otherwise nothing is printed. The problem is that the user may have custom configuration settings (in their ~/.hgrc file or similar) that changes the output of 'hg id' in a way that the script does not expect. In such cases, the Mercurial revision may not be printed or printed incorrectly. It is good practice to ignore the user environment when calling Mercurial commands from a well-defined script, by setting the environment variable HGRCPATH to the empty string. See also 'hg help environment'. In the particular case of Nokia, a custom extension adds dynamic tags in the repository, i.e. tags that are stored in a file external to the repository and only visible when the extension is active. These tags should not influence the behavior of setlocalversion as they are not official Buildroot tags, i.e. even if a revision is tagged, the Mercurial revision should still be printed. Note that this still does not solve the problem where an organization adds _real_ tags in their Buildroot repository. For example, there might be a moving tag 'last-validated' or tags indicating in which product release that Buildroot revision was used. In these cases, setlocalversion will still not behave as expected, i.e. show the Mercurial revision. Signed-off-by: Thomas De Schampheleire <thomas.de_schampheleire@nokia.com> Signed-off-by: Thomas Petazzoni <thomas.petazzoni@bootlin.com>
83 lines
2 KiB
Bash
Executable file
83 lines
2 KiB
Bash
Executable file
#!/bin/sh
|
|
#
|
|
# This scripts adds local version information from the version
|
|
# control systems git, mercurial (hg) and subversion (svn).
|
|
#
|
|
# If something goes wrong, send a mail the kernel build mailinglist
|
|
# (see MAINTAINERS) and CC Nico Schottelius
|
|
# <nico-linuxsetlocalversion -at- schottelius.org>.
|
|
#
|
|
#
|
|
|
|
usage() {
|
|
echo "Usage: $0 [srctree]" >&2
|
|
exit 1
|
|
}
|
|
|
|
cd "${1:-.}" || usage
|
|
|
|
# Check for git and a git repo.
|
|
if head=`git rev-parse --verify --short HEAD 2>/dev/null`; then
|
|
|
|
# If we are at a tagged commit (like "v2.6.30-rc6"), we ignore it,
|
|
# because this version is defined in the top level Makefile.
|
|
if [ -z "`git describe --exact-match 2>/dev/null`" ]; then
|
|
|
|
# If we are past a tagged commit (like "v2.6.30-rc5-302-g72357d5"),
|
|
# we pretty print it.
|
|
if atag="`git describe 2>/dev/null`"; then
|
|
echo "$atag" | awk -F- '{printf("-%05d-%s", $(NF-1),$(NF))}'
|
|
|
|
# If we don't have a tag at all we print -g{commitish}.
|
|
else
|
|
printf '%s%s' -g $head
|
|
fi
|
|
fi
|
|
|
|
# Is this git on svn?
|
|
if git config --get svn-remote.svn.url >/dev/null; then
|
|
printf -- '-svn%s' "`git svn find-rev $head`"
|
|
fi
|
|
|
|
# Update index only on r/w media
|
|
[ -w . ] && git update-index --refresh --unmerged > /dev/null
|
|
|
|
# Check for uncommitted changes
|
|
if git diff-index --name-only HEAD | grep -v "^scripts/package" \
|
|
| read dummy; then
|
|
printf '%s' -dirty
|
|
fi
|
|
|
|
# All done with git
|
|
exit
|
|
fi
|
|
|
|
# Check for mercurial and a mercurial repo.
|
|
if hgid=`HGRCPATH= hg id --id --tags 2>/dev/null`; then
|
|
tag=`printf '%s' "$hgid" | cut -d' ' -f2 --only-delimited`
|
|
|
|
# Do we have an untagged version?
|
|
if [ -z "$tag" -o "$tag" = tip ]; then
|
|
id=`printf '%s' "$hgid" | sed 's/[+ ].*//'`
|
|
printf '%s%s' -hg "$id"
|
|
fi
|
|
|
|
# Are there uncommitted changes?
|
|
# These are represented by + after the changeset id.
|
|
case "$hgid" in
|
|
*+|*+\ *) printf '%s' -dirty ;;
|
|
esac
|
|
|
|
# All done with mercurial
|
|
exit
|
|
fi
|
|
|
|
# Check for svn and a svn repo.
|
|
if rev=`LC_ALL=C svn info 2>/dev/null | grep '^Last Changed Rev'`; then
|
|
rev=`echo $rev | awk '{print $NF}'`
|
|
printf -- '-svn%s' "$rev"
|
|
|
|
# All done with svn
|
|
exit
|
|
fi
|