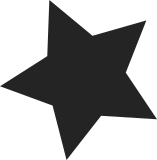
Adds support for hardware i2c to the zephyr port. Similar to other ports such as stm32 and nrf, we only implement the i2c protocol functions (readfrom and writeto) and defer memory operations (readfrom_mem, readfrom_mem_into, and writeto_mem) to the software i2c implementation. This may need to change in the future because zephyr is considering deprecating its i2c_transfer function in favor of i2c_write_read; in this case we would probably want to implement the memory operations directly using i2c_write_read. Tested with the accelerometer on frdm_k64f and bbc_microbit boards.
31 lines
1,009 B
C
31 lines
1,009 B
C
#include <zephyr.h>
|
|
#include "lib/utils/interrupt_char.h"
|
|
|
|
static inline mp_uint_t mp_hal_ticks_us(void) {
|
|
return SYS_CLOCK_HW_CYCLES_TO_NS(k_cycle_get_32()) / 1000;
|
|
}
|
|
|
|
static inline mp_uint_t mp_hal_ticks_ms(void) {
|
|
return k_uptime_get();
|
|
}
|
|
|
|
static inline mp_uint_t mp_hal_ticks_cpu(void) {
|
|
// ticks_cpu() is defined as using the highest-resolution timing source
|
|
// in the system. This is usually a CPU clock, but doesn't have to be,
|
|
// here we just use Zephyr hi-res timer.
|
|
return k_cycle_get_32();
|
|
}
|
|
|
|
static inline void mp_hal_delay_us(mp_uint_t delay) {
|
|
k_busy_wait(delay);
|
|
}
|
|
|
|
static inline void mp_hal_delay_ms(mp_uint_t delay) {
|
|
k_sleep(delay);
|
|
}
|
|
|
|
#define mp_hal_delay_us_fast(us) (mp_hal_delay_us(us))
|
|
#define mp_hal_pin_od_low(p) (mp_raise_NotImplementedError("mp_hal_pin_od_low"))
|
|
#define mp_hal_pin_od_high(p) (mp_raise_NotImplementedError("mp_hal_pin_od_high"))
|
|
#define mp_hal_pin_open_drain(p) (mp_raise_NotImplementedError("mp_hal_pin_open_drain"))
|