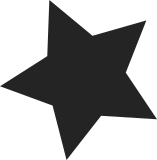
DMA routers are transparent devices used to mux DMA requests from peripherals to DMA controllers. They are used when the SoC integrates more devices with DMA requests then their controller can handle. DRA7x is one example of such SoC, where the sDMA can hanlde 128 DMA request lines, but in SoC level it has 205 DMA requests. The of_dma_router will be registered as of_dma_controller with special xlate function and additional parameters. The driver for the router is responsible to craft the dma_spec (in the of_dma_route_allocate callback) which can be used to requests a DMA channel from the real DMA controller. This way the router can be transparent for the system while remaining generic enough to be used in different environments. Signed-off-by: Peter Ujfalusi <peter.ujfalusi@ti.com> Signed-off-by: Vinod Koul <vinod.koul@intel.com>
97 lines
2.4 KiB
C
97 lines
2.4 KiB
C
/*
|
|
* OF helpers for DMA request / controller
|
|
*
|
|
* Based on of_gpio.h
|
|
*
|
|
* Copyright (C) 2012 Texas Instruments Incorporated - http://www.ti.com/
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*/
|
|
|
|
#ifndef __LINUX_OF_DMA_H
|
|
#define __LINUX_OF_DMA_H
|
|
|
|
#include <linux/of.h>
|
|
#include <linux/dmaengine.h>
|
|
|
|
struct device_node;
|
|
|
|
struct of_dma {
|
|
struct list_head of_dma_controllers;
|
|
struct device_node *of_node;
|
|
struct dma_chan *(*of_dma_xlate)
|
|
(struct of_phandle_args *, struct of_dma *);
|
|
void *(*of_dma_route_allocate)
|
|
(struct of_phandle_args *, struct of_dma *);
|
|
struct dma_router *dma_router;
|
|
void *of_dma_data;
|
|
};
|
|
|
|
struct of_dma_filter_info {
|
|
dma_cap_mask_t dma_cap;
|
|
dma_filter_fn filter_fn;
|
|
};
|
|
|
|
#ifdef CONFIG_OF
|
|
extern int of_dma_controller_register(struct device_node *np,
|
|
struct dma_chan *(*of_dma_xlate)
|
|
(struct of_phandle_args *, struct of_dma *),
|
|
void *data);
|
|
extern void of_dma_controller_free(struct device_node *np);
|
|
|
|
extern int of_dma_router_register(struct device_node *np,
|
|
void *(*of_dma_route_allocate)
|
|
(struct of_phandle_args *, struct of_dma *),
|
|
struct dma_router *dma_router);
|
|
#define of_dma_router_free of_dma_controller_free
|
|
|
|
extern struct dma_chan *of_dma_request_slave_channel(struct device_node *np,
|
|
const char *name);
|
|
extern struct dma_chan *of_dma_simple_xlate(struct of_phandle_args *dma_spec,
|
|
struct of_dma *ofdma);
|
|
extern struct dma_chan *of_dma_xlate_by_chan_id(struct of_phandle_args *dma_spec,
|
|
struct of_dma *ofdma);
|
|
|
|
#else
|
|
static inline int of_dma_controller_register(struct device_node *np,
|
|
struct dma_chan *(*of_dma_xlate)
|
|
(struct of_phandle_args *, struct of_dma *),
|
|
void *data)
|
|
{
|
|
return -ENODEV;
|
|
}
|
|
|
|
static inline void of_dma_controller_free(struct device_node *np)
|
|
{
|
|
}
|
|
|
|
static inline int of_dma_router_register(struct device_node *np,
|
|
void *(*of_dma_route_allocate)
|
|
(struct of_phandle_args *, struct of_dma *),
|
|
struct dma_router *dma_router)
|
|
{
|
|
return -ENODEV;
|
|
}
|
|
|
|
#define of_dma_router_free of_dma_controller_free
|
|
|
|
static inline struct dma_chan *of_dma_request_slave_channel(struct device_node *np,
|
|
const char *name)
|
|
{
|
|
return NULL;
|
|
}
|
|
|
|
static inline struct dma_chan *of_dma_simple_xlate(struct of_phandle_args *dma_spec,
|
|
struct of_dma *ofdma)
|
|
{
|
|
return NULL;
|
|
}
|
|
|
|
#define of_dma_xlate_by_chan_id NULL
|
|
|
|
#endif
|
|
|
|
#endif /* __LINUX_OF_DMA_H */
|