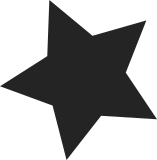
This patch proposes to remove the IRQF_DISABLED flag from CRIS architecture code. It's a NOOP since 2.6.35 and it will be removed one day. Comments mentioning IRQF_DISABLED are also updated, knowing that all interrupts are now "fast interrupts", their handlers running with interrupts disabled. Don't hesitate to let me know if you have other ways of rephrasing the comments! This is an update for 3.11 of a patch already sent for 3.10 Signed-off-by: Michael Opdenacker <michael.opdenacker@free-electrons.com> Signed-off-by: Jesper Nilsson <jespern@axis.com>
67 lines
1.6 KiB
C
67 lines
1.6 KiB
C
/*
|
|
*
|
|
* linux/arch/cris/kernel/irq.c
|
|
*
|
|
* Copyright (c) 2000,2007 Axis Communications AB
|
|
*
|
|
* Authors: Bjorn Wesen (bjornw@axis.com)
|
|
*
|
|
* This file contains the code used by various IRQ handling routines:
|
|
* asking for different IRQs should be done through these routines
|
|
* instead of just grabbing them. Thus setups with different IRQ numbers
|
|
* shouldn't result in any weird surprises, and installing new handlers
|
|
* should be easier.
|
|
*
|
|
*/
|
|
|
|
/*
|
|
* IRQs are in fact implemented a bit like signal handlers for the kernel.
|
|
* Naturally it's not a 1:1 relation, but there are similarities.
|
|
*/
|
|
|
|
#include <linux/module.h>
|
|
#include <linux/ptrace.h>
|
|
#include <linux/irq.h>
|
|
|
|
#include <linux/kernel_stat.h>
|
|
#include <linux/signal.h>
|
|
#include <linux/sched.h>
|
|
#include <linux/ioport.h>
|
|
#include <linux/interrupt.h>
|
|
#include <linux/timex.h>
|
|
#include <linux/random.h>
|
|
#include <linux/init.h>
|
|
#include <linux/seq_file.h>
|
|
#include <linux/errno.h>
|
|
#include <linux/spinlock.h>
|
|
|
|
#include <asm/io.h>
|
|
#include <arch/system.h>
|
|
|
|
/* called by the assembler IRQ entry functions defined in irq.h
|
|
* to dispatch the interrupts to registered handlers
|
|
*/
|
|
|
|
asmlinkage void do_IRQ(int irq, struct pt_regs * regs)
|
|
{
|
|
unsigned long sp;
|
|
struct pt_regs *old_regs = set_irq_regs(regs);
|
|
irq_enter();
|
|
sp = rdsp();
|
|
if (unlikely((sp & (PAGE_SIZE - 1)) < (PAGE_SIZE/8))) {
|
|
printk("do_IRQ: stack overflow: %lX\n", sp);
|
|
show_stack(NULL, (unsigned long *)sp);
|
|
}
|
|
generic_handle_irq(irq);
|
|
irq_exit();
|
|
set_irq_regs(old_regs);
|
|
}
|
|
|
|
void weird_irq(void)
|
|
{
|
|
local_irq_disable();
|
|
printk("weird irq\n");
|
|
while(1);
|
|
}
|
|
|