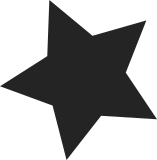
resurrect an old patch from Pablo Neira to remove the untracked objects. Currently, there are four possible states of an skb wrt. conntrack. 1. No conntrack attached, ct is NULL. 2. Normal (kmem cache allocated) ct attached. 3. a template (kmalloc'd), not in any hash tables at any point in time 4. the 'untracked' conntrack, a percpu nf_conn object, tagged via IPS_UNTRACKED_BIT in ct->status. Untracked is supposed to be identical to case 1. It exists only so users can check -m conntrack --ctstate UNTRACKED vs. -m conntrack --ctstate INVALID e.g. attempts to set connmark on INVALID or UNTRACKED conntracks is supposed to be a no-op. Thus currently we need to check ct == NULL || nf_ct_is_untracked(ct) in a lot of places in order to avoid altering untracked objects. The other consequence of the percpu untracked object is that all -j NOTRACK (and, later, kfree_skb of such skbs) result in an atomic op (inc/dec the untracked conntracks refcount). This adds a new kernel-private ctinfo state, IP_CT_UNTRACKED, to make the distinction instead. The (few) places that care about packet invalid (ct is NULL) vs. packet untracked now need to test ct == NULL vs. ctinfo == IP_CT_UNTRACKED, but all other places can omit the nf_ct_is_untracked() check. Signed-off-by: Florian Westphal <fw@strlen.de> Signed-off-by: Pablo Neira Ayuso <pablo@netfilter.org>
82 lines
2.2 KiB
C
82 lines
2.2 KiB
C
/*
|
|
* (C) 2007 by Sebastian Claßen <sebastian.classen@freenet.ag>
|
|
* (C) 2007-2010 by Jan Engelhardt <jengelh@medozas.de>
|
|
*
|
|
* Extracted from xt_TEE.c
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License version 2 or later, as
|
|
* published by the Free Software Foundation.
|
|
*/
|
|
#include <linux/module.h>
|
|
#include <linux/percpu.h>
|
|
#include <linux/skbuff.h>
|
|
#include <linux/netfilter.h>
|
|
#include <net/ipv6.h>
|
|
#include <net/ip6_route.h>
|
|
#include <net/netfilter/ipv6/nf_dup_ipv6.h>
|
|
#if IS_ENABLED(CONFIG_NF_CONNTRACK)
|
|
#include <net/netfilter/nf_conntrack.h>
|
|
#endif
|
|
|
|
static bool nf_dup_ipv6_route(struct net *net, struct sk_buff *skb,
|
|
const struct in6_addr *gw, int oif)
|
|
{
|
|
const struct ipv6hdr *iph = ipv6_hdr(skb);
|
|
struct dst_entry *dst;
|
|
struct flowi6 fl6;
|
|
|
|
memset(&fl6, 0, sizeof(fl6));
|
|
if (oif != -1)
|
|
fl6.flowi6_oif = oif;
|
|
|
|
fl6.daddr = *gw;
|
|
fl6.flowlabel = (__force __be32)(((iph->flow_lbl[0] & 0xF) << 16) |
|
|
(iph->flow_lbl[1] << 8) | iph->flow_lbl[2]);
|
|
fl6.flowi6_flags = FLOWI_FLAG_KNOWN_NH;
|
|
dst = ip6_route_output(net, NULL, &fl6);
|
|
if (dst->error) {
|
|
dst_release(dst);
|
|
return false;
|
|
}
|
|
skb_dst_drop(skb);
|
|
skb_dst_set(skb, dst);
|
|
skb->dev = dst->dev;
|
|
skb->protocol = htons(ETH_P_IPV6);
|
|
|
|
return true;
|
|
}
|
|
|
|
void nf_dup_ipv6(struct net *net, struct sk_buff *skb, unsigned int hooknum,
|
|
const struct in6_addr *gw, int oif)
|
|
{
|
|
if (this_cpu_read(nf_skb_duplicated))
|
|
return;
|
|
skb = pskb_copy(skb, GFP_ATOMIC);
|
|
if (skb == NULL)
|
|
return;
|
|
|
|
#if IS_ENABLED(CONFIG_NF_CONNTRACK)
|
|
nf_reset(skb);
|
|
nf_ct_set(skb, NULL, IP_CT_UNTRACKED);
|
|
#endif
|
|
if (hooknum == NF_INET_PRE_ROUTING ||
|
|
hooknum == NF_INET_LOCAL_IN) {
|
|
struct ipv6hdr *iph = ipv6_hdr(skb);
|
|
--iph->hop_limit;
|
|
}
|
|
if (nf_dup_ipv6_route(net, skb, gw, oif)) {
|
|
__this_cpu_write(nf_skb_duplicated, true);
|
|
ip6_local_out(net, skb->sk, skb);
|
|
__this_cpu_write(nf_skb_duplicated, false);
|
|
} else {
|
|
kfree_skb(skb);
|
|
}
|
|
}
|
|
EXPORT_SYMBOL_GPL(nf_dup_ipv6);
|
|
|
|
MODULE_AUTHOR("Sebastian Claßen <sebastian.classen@freenet.ag>");
|
|
MODULE_AUTHOR("Jan Engelhardt <jengelh@medozas.de>");
|
|
MODULE_DESCRIPTION("nf_dup_ipv6: IPv6 packet duplication");
|
|
MODULE_LICENSE("GPL");
|