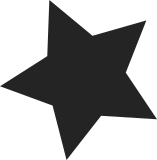
-----BEGIN PGP SIGNATURE----- Version: GnuPG v1.4.11 (GNU/Linux) iQIcBAABAgAGBQJPD2aFAAoJENkgDmzRrbjxNzsQAIeYbbrXYLjr6kQzUSngj/eC FzjaTEfYTQIeuQCFJHcHthyc5lXV4sQbo3jOezW+Bp5yuDJL2aWIHesSfWZe7imu zQdM4VshOYdAmUR9Q0AW5zhB8Smbs7/AyABiF2jm4p0ZPOuyMDSlei9sjvE9Vjvt B7g5ht7L6kz0JbDnwwy0u5gs+tEitwpXYId9Y4ysZIBzIbL0qkPX8veOddGTMy0N 8xhWXaKtufpjvxFD2ORLDsw3AkoF1xXSNuFd/5nzCNpbeE7TW931jfkPoqJumuAO 7GLxcU9kKYl+IICobC6wBtsj/RrB7w+cBXMvPGwdBliam1qaRhUcJZi5FLM/Ha5d 2A9QDYNUpoXiO8JbPXrV9Z+Y0+Co8RilsQj7R/rjZh6AbbYCWt9nxzx2Svl/RfTr xfiimHuB2P3rHjOvpCXULwOOuE5c8MzPuWncpdjiD3uGXOY/aY+X1m+if/quJw9D grPlKL0+YiRakEYUeGG4M77KCqyKFZaF7L7UQPbqfZcj8V/9AW3/7U5I/B9RlAjs idsr4fcf5s0N+oKUyTCW1ncpUDQNiwbU2NyJQqeu1ZxaRGj72AgyvsaNeyIPDyK+ f6x95Bi7i8KLjXc9Z1KvJwh2Nxt25gNUiTYVha/9H2NpJGd1cfI15kTOGXrgddVv 1pvuGcJDZwYiwfiXr3FL =HHrh -----END PGP SIGNATURE----- Merge tag 'for-linus' of git://github.com/rustyrussell/linux Autogenerated GPG tag for Rusty D1ADB8F1: 15EE 8D6C AB0E 7F0C F999 BFCB D920 0E6C D1AD B8F1 * tag 'for-linus' of git://github.com/rustyrussell/linux: module_param: check that bool parameters really are bool. intelfbdrv.c: bailearly is an int module_param paride/pcd: fix bool verbose module parameter. module_param: make bool parameters really bool (drivers & misc) module_param: make bool parameters really bool (arch) module_param: make bool parameters really bool (core code) kernel/async: remove redundant declaration. printk: fix unnecessary module_param_name. lirc_parallel: fix module parameter description. module_param: avoid bool abuse, add bint for special cases. module_param: check type correctness for module_param_array modpost: use linker section to generate table. modpost: use a table rather than a giant if/else statement. modules: sysfs - export: taint, coresize, initsize kernel/params: replace DEBUGP with pr_debug module: replace DEBUGP with pr_debug module: struct module_ref should contains long fields module: Fix performance regression on modules with large symbol tables module: Add comments describing how the "strmap" logic works Fix up conflicts in scripts/mod/file2alias.c due to the new linker- generated table approach to adding __mod_*_device_table entries. The ARM sa11x0 mcp bus needed to be converted to that too.
81 lines
2.4 KiB
C
81 lines
2.4 KiB
C
/*
|
|
* linux/drivers/mmc/core/core.h
|
|
*
|
|
* Copyright (C) 2003 Russell King, All Rights Reserved.
|
|
* Copyright 2007 Pierre Ossman
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*/
|
|
#ifndef _MMC_CORE_CORE_H
|
|
#define _MMC_CORE_CORE_H
|
|
|
|
#include <linux/delay.h>
|
|
|
|
#define MMC_CMD_RETRIES 3
|
|
|
|
struct mmc_bus_ops {
|
|
int (*awake)(struct mmc_host *);
|
|
int (*sleep)(struct mmc_host *);
|
|
void (*remove)(struct mmc_host *);
|
|
void (*detect)(struct mmc_host *);
|
|
int (*suspend)(struct mmc_host *);
|
|
int (*resume)(struct mmc_host *);
|
|
int (*power_save)(struct mmc_host *);
|
|
int (*power_restore)(struct mmc_host *);
|
|
int (*alive)(struct mmc_host *);
|
|
};
|
|
|
|
void mmc_attach_bus(struct mmc_host *host, const struct mmc_bus_ops *ops);
|
|
void mmc_detach_bus(struct mmc_host *host);
|
|
|
|
void mmc_init_erase(struct mmc_card *card);
|
|
|
|
void mmc_set_chip_select(struct mmc_host *host, int mode);
|
|
void mmc_set_clock(struct mmc_host *host, unsigned int hz);
|
|
void mmc_gate_clock(struct mmc_host *host);
|
|
void mmc_ungate_clock(struct mmc_host *host);
|
|
void mmc_set_ungated(struct mmc_host *host);
|
|
void mmc_set_bus_mode(struct mmc_host *host, unsigned int mode);
|
|
void mmc_set_bus_width(struct mmc_host *host, unsigned int width);
|
|
u32 mmc_select_voltage(struct mmc_host *host, u32 ocr);
|
|
int mmc_set_signal_voltage(struct mmc_host *host, int signal_voltage,
|
|
bool cmd11);
|
|
void mmc_set_timing(struct mmc_host *host, unsigned int timing);
|
|
void mmc_set_driver_type(struct mmc_host *host, unsigned int drv_type);
|
|
void mmc_power_off(struct mmc_host *host);
|
|
|
|
static inline void mmc_delay(unsigned int ms)
|
|
{
|
|
if (ms < 1000 / HZ) {
|
|
cond_resched();
|
|
mdelay(ms);
|
|
} else {
|
|
msleep(ms);
|
|
}
|
|
}
|
|
|
|
void mmc_rescan(struct work_struct *work);
|
|
void mmc_start_host(struct mmc_host *host);
|
|
void mmc_stop_host(struct mmc_host *host);
|
|
|
|
int _mmc_detect_card_removed(struct mmc_host *host);
|
|
|
|
int mmc_attach_mmc(struct mmc_host *host);
|
|
int mmc_attach_sd(struct mmc_host *host);
|
|
int mmc_attach_sdio(struct mmc_host *host);
|
|
|
|
/* Module parameters */
|
|
extern bool use_spi_crc;
|
|
|
|
/* Debugfs information for hosts and cards */
|
|
void mmc_add_host_debugfs(struct mmc_host *host);
|
|
void mmc_remove_host_debugfs(struct mmc_host *host);
|
|
|
|
void mmc_add_card_debugfs(struct mmc_card *card);
|
|
void mmc_remove_card_debugfs(struct mmc_card *card);
|
|
|
|
#endif
|
|
|