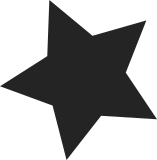
This patch adds the kernel module ib_srpt SCSI RDMA Protocol (SRP) target implementation conforming to the SRP r16a specification for the mainline drivers/target infrastructure. This driver was originally developed by Vu Pham and has been optimized by Bart Van Assche and merged into upstream LIO based on his srpt-lio-4.1 branch here: https://github.com/bvanassche/srpt-lio/commits/srpt-lio-4.1/ This updated patch also contains the following two changes from lio-core-2.6.git/master. One is to fix a bug with 1 >= task->task_sg[] chained mappings in ib_srpt, and the other to convert the configfs control plane to reference IB Port GUID and struct srpt_port directly following mainline v4.x target_core_fabric_configfs.c convertion for ib_srpt to work with rtslib/rtsadmin v2 code. These seperate patches can be found here: ib_srpt: Fix bug with chainged SGLs in srpt_map_sg_to_ib_sge http://www.risingtidesystems.com/git/?p=lio-core-2.6.git;a=commitdiff;h=ea485147563b6555a97dbf811825fbb586519252 ib_srpt: Convert se_wwn endpoint reference to struct srpt_port->port_wwn http://www.risingtidesystems.com/git/?p=lio-core-2.6.git;a=commitdiff;h=4e544a210acb227df1bb4ca5086e65bdf4e648ea This also includes the following recent v1 -> v2 review changes: ib_srpt: Fix potential out-of-bounds array access ib_srpt: Avoid failed multipart RDMA transfers ib_srpt: Fix srpt_alloc_fabric_acl failure case return value ib_srpt: Update comments to reference $driver/$port layout ib_srpt: Fix sport->port_guid formatting code ib_srpt: Remove legacy use_port_guid_in_session_name module parameter ib_srpt: Convert srp_max_rdma_size into per port configfs attribute ib_srpt: Convert srp_max_rsp_size into per port configfs attribute ib_srpt: Convert srpt_sq_size into per port configfs attribute and v2 -> v3 review changes: ib_srpt: Fix possible race with srp_sq_size in srpt_create_ch_ib ib_srpt: Fix possible race with srp_max_rsp_size in srpt_release_channel_work ib_srpt: Fix up MAX_SRPT_RDMA_SIZE define ib_srpt: Make srpt_map_sg_to_ib_sge() failure case return -EAGAIN ib_srpt: Convert port_guid to use subnet_prefix + interface_id formatting ib_srpt: Make srpt_check_stop_free return kref_put status ib_srpt: Make compilation with BUG=n proceed` ib_srpt: Use new target_core_fabric.h include ib_srpt: Check hex2bin() return code to silence build warning Cc: Bart Van Assche <bvanassche@acm.org> Cc: Roland Dreier <roland@purestorage.com> Cc: Christoph Hellwig <hch@lst.de> Cc: Vu Pham <vu@mellanox.com> Cc: David Dillow <dillowda@ornl.gov> Signed-off-by: Nicholas A. Bellinger <nab@risingtidesystems.com>
140 lines
4 KiB
C
140 lines
4 KiB
C
/*
|
|
* Copyright (c) 2006 - 2009 Mellanox Technology Inc. All rights reserved.
|
|
*
|
|
* This software is available to you under a choice of one of two
|
|
* licenses. You may choose to be licensed under the terms of the GNU
|
|
* General Public License (GPL) Version 2, available from the file
|
|
* COPYING in the main directory of this source tree, or the
|
|
* OpenIB.org BSD license below:
|
|
*
|
|
* Redistribution and use in source and binary forms, with or
|
|
* without modification, are permitted provided that the following
|
|
* conditions are met:
|
|
*
|
|
* - Redistributions of source code must retain the above
|
|
* copyright notice, this list of conditions and the following
|
|
* disclaimer.
|
|
*
|
|
* - Redistributions in binary form must reproduce the above
|
|
* copyright notice, this list of conditions and the following
|
|
* disclaimer in the documentation and/or other materials
|
|
* provided with the distribution.
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
|
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
|
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
|
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS
|
|
* BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN
|
|
* ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
|
|
* CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
|
* SOFTWARE.
|
|
*
|
|
*/
|
|
|
|
#ifndef IB_DM_MAD_H
|
|
#define IB_DM_MAD_H
|
|
|
|
#include <linux/types.h>
|
|
|
|
#include <rdma/ib_mad.h>
|
|
|
|
enum {
|
|
/*
|
|
* See also section 13.4.7 Status Field, table 115 MAD Common Status
|
|
* Field Bit Values and also section 16.3.1.1 Status Field in the
|
|
* InfiniBand Architecture Specification.
|
|
*/
|
|
DM_MAD_STATUS_UNSUP_METHOD = 0x0008,
|
|
DM_MAD_STATUS_UNSUP_METHOD_ATTR = 0x000c,
|
|
DM_MAD_STATUS_INVALID_FIELD = 0x001c,
|
|
DM_MAD_STATUS_NO_IOC = 0x0100,
|
|
|
|
/*
|
|
* See also the Device Management chapter, section 16.3.3 Attributes,
|
|
* table 279 Device Management Attributes in the InfiniBand
|
|
* Architecture Specification.
|
|
*/
|
|
DM_ATTR_CLASS_PORT_INFO = 0x01,
|
|
DM_ATTR_IOU_INFO = 0x10,
|
|
DM_ATTR_IOC_PROFILE = 0x11,
|
|
DM_ATTR_SVC_ENTRIES = 0x12
|
|
};
|
|
|
|
struct ib_dm_hdr {
|
|
u8 reserved[28];
|
|
};
|
|
|
|
/*
|
|
* Structure of management datagram sent by the SRP target implementation.
|
|
* Contains a management datagram header, reliable multi-packet transaction
|
|
* protocol (RMPP) header and ib_dm_hdr. Notes:
|
|
* - The SRP target implementation does not use RMPP or ib_dm_hdr when sending
|
|
* management datagrams.
|
|
* - The header size must be exactly 64 bytes (IB_MGMT_DEVICE_HDR), since this
|
|
* is the header size that is passed to ib_create_send_mad() in ib_srpt.c.
|
|
* - The maximum supported size for a management datagram when not using RMPP
|
|
* is 256 bytes -- 64 bytes header and 192 (IB_MGMT_DEVICE_DATA) bytes data.
|
|
*/
|
|
struct ib_dm_mad {
|
|
struct ib_mad_hdr mad_hdr;
|
|
struct ib_rmpp_hdr rmpp_hdr;
|
|
struct ib_dm_hdr dm_hdr;
|
|
u8 data[IB_MGMT_DEVICE_DATA];
|
|
};
|
|
|
|
/*
|
|
* IOUnitInfo as defined in section 16.3.3.3 IOUnitInfo of the InfiniBand
|
|
* Architecture Specification.
|
|
*/
|
|
struct ib_dm_iou_info {
|
|
__be16 change_id;
|
|
u8 max_controllers;
|
|
u8 op_rom;
|
|
u8 controller_list[128];
|
|
};
|
|
|
|
/*
|
|
* IOControllerprofile as defined in section 16.3.3.4 IOControllerProfile of
|
|
* the InfiniBand Architecture Specification.
|
|
*/
|
|
struct ib_dm_ioc_profile {
|
|
__be64 guid;
|
|
__be32 vendor_id;
|
|
__be32 device_id;
|
|
__be16 device_version;
|
|
__be16 reserved1;
|
|
__be32 subsys_vendor_id;
|
|
__be32 subsys_device_id;
|
|
__be16 io_class;
|
|
__be16 io_subclass;
|
|
__be16 protocol;
|
|
__be16 protocol_version;
|
|
__be16 service_conn;
|
|
__be16 initiators_supported;
|
|
__be16 send_queue_depth;
|
|
u8 reserved2;
|
|
u8 rdma_read_depth;
|
|
__be32 send_size;
|
|
__be32 rdma_size;
|
|
u8 op_cap_mask;
|
|
u8 svc_cap_mask;
|
|
u8 num_svc_entries;
|
|
u8 reserved3[9];
|
|
u8 id_string[64];
|
|
};
|
|
|
|
struct ib_dm_svc_entry {
|
|
u8 name[40];
|
|
__be64 id;
|
|
};
|
|
|
|
/*
|
|
* See also section 16.3.3.5 ServiceEntries in the InfiniBand Architecture
|
|
* Specification. See also section B.7, table B.8 in the T10 SRP r16a document.
|
|
*/
|
|
struct ib_dm_svc_entries {
|
|
struct ib_dm_svc_entry service_entries[4];
|
|
};
|
|
|
|
#endif
|