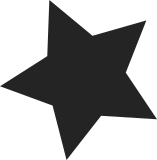
Checksums were only verified by btrfs_read_tree_block, which meant the functions to probe the page cache for blocks were not validating checksums. Normally this is fine because the buffers will only be in cache if they have already been validated. But, there is a window while the buffer is being read from disk where it could be up to date in the cache but not yet verified. This patch makes sure all buffers go through checksum verification before they are used. This is safer, and it prevents modification of buffers before they go through the csum code. Signed-off-by: Chris Mason <chris.mason@oracle.com>
75 lines
3.3 KiB
C
75 lines
3.3 KiB
C
/*
|
|
* Copyright (C) 2007 Oracle. All rights reserved.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public
|
|
* License v2 as published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public
|
|
* License along with this program; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 021110-1307, USA.
|
|
*/
|
|
|
|
#ifndef __DISKIO__
|
|
#define __DISKIO__
|
|
|
|
#define BTRFS_SUPER_INFO_OFFSET (16 * 1024)
|
|
struct btrfs_device;
|
|
struct btrfs_fs_devices;
|
|
|
|
struct extent_buffer *read_tree_block(struct btrfs_root *root, u64 bytenr,
|
|
u32 blocksize);
|
|
int readahead_tree_block(struct btrfs_root *root, u64 bytenr, u32 blocksize);
|
|
struct extent_buffer *btrfs_find_create_tree_block(struct btrfs_root *root,
|
|
u64 bytenr, u32 blocksize);
|
|
int clean_tree_block(struct btrfs_trans_handle *trans,
|
|
struct btrfs_root *root, struct extent_buffer *buf);
|
|
struct btrfs_root *open_ctree(struct super_block *sb,
|
|
struct btrfs_fs_devices *fs_devices);
|
|
int close_ctree(struct btrfs_root *root);
|
|
int write_ctree_super(struct btrfs_trans_handle *trans,
|
|
struct btrfs_root *root);
|
|
struct extent_buffer *btrfs_find_tree_block(struct btrfs_root *root,
|
|
u64 bytenr, u32 blocksize);
|
|
struct btrfs_root *btrfs_lookup_fs_root(struct btrfs_fs_info *fs_info,
|
|
u64 root_objectid);
|
|
struct btrfs_root *btrfs_read_fs_root(struct btrfs_fs_info *fs_info,
|
|
struct btrfs_key *location,
|
|
const char *name, int namelen);
|
|
struct btrfs_root *btrfs_read_fs_root_no_radix(struct btrfs_fs_info *fs_info,
|
|
struct btrfs_key *location);
|
|
struct btrfs_root *btrfs_read_fs_root_no_name(struct btrfs_fs_info *fs_info,
|
|
struct btrfs_key *location);
|
|
int btrfs_insert_dev_radix(struct btrfs_root *root,
|
|
struct block_device *bdev,
|
|
u64 device_id,
|
|
u64 block_start,
|
|
u64 num_blocks);
|
|
void btrfs_btree_balance_dirty(struct btrfs_root *root, unsigned long nr);
|
|
int btrfs_free_fs_root(struct btrfs_fs_info *fs_info, struct btrfs_root *root);
|
|
void btrfs_mark_buffer_dirty(struct extent_buffer *buf);
|
|
int btrfs_buffer_uptodate(struct extent_buffer *buf);
|
|
int btrfs_set_buffer_uptodate(struct extent_buffer *buf);
|
|
int wait_on_tree_block_writeback(struct btrfs_root *root,
|
|
struct extent_buffer *buf);
|
|
void btrfs_set_buffer_defrag(struct extent_buffer *buf);
|
|
void btrfs_set_buffer_defrag_done(struct extent_buffer *buf);
|
|
int btrfs_buffer_defrag(struct extent_buffer *buf);
|
|
int btrfs_buffer_defrag_done(struct extent_buffer *buf);
|
|
int btrfs_clear_buffer_defrag(struct extent_buffer *buf);
|
|
int btrfs_clear_buffer_defrag_done(struct extent_buffer *buf);
|
|
int btrfs_read_buffer(struct extent_buffer *buf);
|
|
u32 btrfs_csum_data(struct btrfs_root *root, char *data, u32 seed, size_t len);
|
|
void btrfs_csum_final(u32 crc, char *result);
|
|
void btrfs_throttle(struct btrfs_root *root);
|
|
int btrfs_open_device(struct btrfs_device *dev);
|
|
int btrfs_verify_block_csum(struct btrfs_root *root,
|
|
struct extent_buffer *buf);
|
|
#endif
|