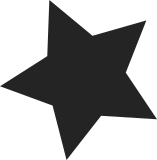
Many of the core ARM kernel files are not modules, but just including module.h for exporting symbols. Now these files can use the lighter footprint export.h for this role. There are probably lots more, but ARM files of mach-* and plat-* don't get coverage via a simple yesconfig build. They will have to be cleaned up and tested via using their respective configs. Signed-off-by: Paul Gortmaker <paul.gortmaker@windriver.com>
51 lines
905 B
C
51 lines
905 B
C
#include <linux/export.h>
|
|
#include <linux/types.h>
|
|
#include <linux/io.h>
|
|
|
|
/*
|
|
* Copy data from IO memory space to "real" memory space.
|
|
* This needs to be optimized.
|
|
*/
|
|
void _memcpy_fromio(void *to, const volatile void __iomem *from, size_t count)
|
|
{
|
|
unsigned char *t = to;
|
|
while (count) {
|
|
count--;
|
|
*t = readb(from);
|
|
t++;
|
|
from++;
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Copy data from "real" memory space to IO memory space.
|
|
* This needs to be optimized.
|
|
*/
|
|
void _memcpy_toio(volatile void __iomem *to, const void *from, size_t count)
|
|
{
|
|
const unsigned char *f = from;
|
|
while (count) {
|
|
count--;
|
|
writeb(*f, to);
|
|
f++;
|
|
to++;
|
|
}
|
|
}
|
|
|
|
/*
|
|
* "memset" on IO memory space.
|
|
* This needs to be optimized.
|
|
*/
|
|
void _memset_io(volatile void __iomem *dst, int c, size_t count)
|
|
{
|
|
while (count) {
|
|
count--;
|
|
writeb(c, dst);
|
|
dst++;
|
|
}
|
|
}
|
|
|
|
EXPORT_SYMBOL(_memcpy_fromio);
|
|
EXPORT_SYMBOL(_memcpy_toio);
|
|
EXPORT_SYMBOL(_memset_io);
|