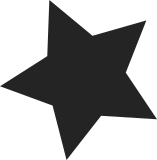
Due to the cross dependencies between hwmod for automanaged device information for OMAP and dts node definitions, we can run into scenarios where the dts node is defined, however it's hwmod entry is yet to be added. In these cases: a) omap_device does not register a pm_domain (since it cannot find hwmod entry). b) driver does not know about (a), does a pm_runtime_get_sync which never fails c) It then tries to do some operation on the device (such as read the revision register (as part of probe) without clock or adequate OMAP generic PM operation performed for enabling the module. This causes a crash such as that reported in: https://bugzilla.kernel.org/show_bug.cgi?id=66441 When 'ti,hwmod' is provided in dt node, it is expected that the device will not function without the OMAP's power automanagement. Hence, when we hit a fail condition (due to hwmod entries not present or other similar scenario), fail at pm_domain level due to lack of data, provide enough information for it to be fixed, however, it allows for the driver to take appropriate measures to prevent crash. Reported-by: Tobias Jakobi <tjakobi@math.uni-bielefeld.de> Signed-off-by: Nishanth Menon <nm@ti.com> Acked-by: Kevin Hilman <khilman@linaro.org> Acked-by: Tony Lindgren <tony@atomide.com> Signed-off-by: Kevin Hilman <khilman@linaro.org>
105 lines
3.2 KiB
C
105 lines
3.2 KiB
C
/*
|
|
* omap_device headers
|
|
*
|
|
* Copyright (C) 2009 Nokia Corporation
|
|
* Paul Walmsley
|
|
*
|
|
* Developed in collaboration with (alphabetical order): Benoit
|
|
* Cousson, Kevin Hilman, Tony Lindgren, Rajendra Nayak, Vikram
|
|
* Pandita, Sakari Poussa, Anand Sawant, Santosh Shilimkar, Richard
|
|
* Woodruff
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*
|
|
* This type of functionality should be implemented as a proper
|
|
* omap_bus/omap_device in Linux.
|
|
*
|
|
* omap_device differs from omap_hwmod in that it includes external
|
|
* (e.g., board- and system-level) integration details. omap_hwmod
|
|
* stores hardware data that is invariant for a given OMAP chip.
|
|
*/
|
|
#ifndef __ARCH_ARM_PLAT_OMAP_INCLUDE_MACH_OMAP_DEVICE_H
|
|
#define __ARCH_ARM_PLAT_OMAP_INCLUDE_MACH_OMAP_DEVICE_H
|
|
|
|
#include <linux/kernel.h>
|
|
#include <linux/platform_device.h>
|
|
|
|
#include "omap_hwmod.h"
|
|
|
|
extern struct dev_pm_domain omap_device_pm_domain;
|
|
extern struct dev_pm_domain omap_device_fail_pm_domain;
|
|
|
|
/* omap_device._state values */
|
|
#define OMAP_DEVICE_STATE_UNKNOWN 0
|
|
#define OMAP_DEVICE_STATE_ENABLED 1
|
|
#define OMAP_DEVICE_STATE_IDLE 2
|
|
#define OMAP_DEVICE_STATE_SHUTDOWN 3
|
|
|
|
/* omap_device.flags values */
|
|
#define OMAP_DEVICE_SUSPENDED BIT(0)
|
|
|
|
/**
|
|
* struct omap_device - omap_device wrapper for platform_devices
|
|
* @pdev: platform_device
|
|
* @hwmods: (one .. many per omap_device)
|
|
* @hwmods_cnt: ARRAY_SIZE() of @hwmods
|
|
* @_state: one of OMAP_DEVICE_STATE_* (see above)
|
|
* @flags: device flags
|
|
* @_driver_status: one of BUS_NOTIFY_*_DRIVER from <linux/device.h>
|
|
*
|
|
* Integrates omap_hwmod data into Linux platform_device.
|
|
*
|
|
* Field names beginning with underscores are for the internal use of
|
|
* the omap_device code.
|
|
*
|
|
*/
|
|
struct omap_device {
|
|
struct platform_device *pdev;
|
|
struct omap_hwmod **hwmods;
|
|
unsigned long _driver_status;
|
|
u8 hwmods_cnt;
|
|
u8 _state;
|
|
u8 flags;
|
|
};
|
|
|
|
/* Device driver interface (call via platform_data fn ptrs) */
|
|
|
|
int omap_device_enable(struct platform_device *pdev);
|
|
int omap_device_idle(struct platform_device *pdev);
|
|
|
|
/* Core code interface */
|
|
|
|
struct platform_device *omap_device_build(const char *pdev_name, int pdev_id,
|
|
struct omap_hwmod *oh, void *pdata,
|
|
int pdata_len);
|
|
|
|
struct platform_device *omap_device_build_ss(const char *pdev_name, int pdev_id,
|
|
struct omap_hwmod **oh, int oh_cnt,
|
|
void *pdata, int pdata_len);
|
|
|
|
struct omap_device *omap_device_alloc(struct platform_device *pdev,
|
|
struct omap_hwmod **ohs, int oh_cnt);
|
|
void omap_device_delete(struct omap_device *od);
|
|
int omap_device_register(struct platform_device *pdev);
|
|
|
|
struct device *omap_device_get_by_hwmod_name(const char *oh_name);
|
|
|
|
/* OMAP PM interface */
|
|
int omap_device_get_context_loss_count(struct platform_device *pdev);
|
|
|
|
/* Other */
|
|
|
|
int omap_device_assert_hardreset(struct platform_device *pdev,
|
|
const char *name);
|
|
int omap_device_deassert_hardreset(struct platform_device *pdev,
|
|
const char *name);
|
|
|
|
/* Get omap_device pointer from platform_device pointer */
|
|
static inline struct omap_device *to_omap_device(struct platform_device *pdev)
|
|
{
|
|
return pdev ? pdev->archdata.od : NULL;
|
|
}
|
|
#endif
|