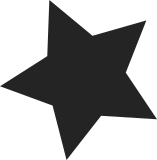
For a channel to make use of SVM features, it requires a different GPU MMU configuration than we would normally use, which is not desirable to switch to unless a client is actively going to use SVM. In order to supporting SVM without more extensive changes to the userspace interfaces, the SVM_INIT ioctl needs to replace the previous configuration safely. The only way we can currently do this safely, accounting for some unlikely failure conditions, is to allocate the new VMM without destroying the last one, and prioritising the SVM-enabled configuration in the code that cares. This will get cleaned up again further down the track. Signed-off-by: Ben Skeggs <bskeggs@redhat.com>
62 lines
1.1 KiB
C
62 lines
1.1 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef __NOUVEAU_CHAN_H__
|
|
#define __NOUVEAU_CHAN_H__
|
|
#include <nvif/object.h>
|
|
#include <nvif/notify.h>
|
|
struct nvif_device;
|
|
|
|
struct nouveau_channel {
|
|
struct nvif_device *device;
|
|
struct nouveau_drm *drm;
|
|
struct nouveau_vmm *vmm;
|
|
|
|
int chid;
|
|
u64 inst;
|
|
u32 token;
|
|
|
|
struct nvif_object vram;
|
|
struct nvif_object gart;
|
|
struct nvif_object nvsw;
|
|
|
|
struct {
|
|
struct nouveau_bo *buffer;
|
|
struct nouveau_vma *vma;
|
|
struct nvif_object ctxdma;
|
|
u64 addr;
|
|
} push;
|
|
|
|
/* TODO: this will be reworked in the near future */
|
|
bool accel_done;
|
|
void *fence;
|
|
struct {
|
|
int max;
|
|
int free;
|
|
int cur;
|
|
int put;
|
|
int ib_base;
|
|
int ib_max;
|
|
int ib_free;
|
|
int ib_put;
|
|
} dma;
|
|
u32 user_get_hi;
|
|
u32 user_get;
|
|
u32 user_put;
|
|
|
|
struct nvif_object user;
|
|
|
|
struct nvif_notify kill;
|
|
atomic_t killed;
|
|
};
|
|
|
|
int nouveau_channels_init(struct nouveau_drm *);
|
|
|
|
int nouveau_channel_new(struct nouveau_drm *, struct nvif_device *,
|
|
u32 arg0, u32 arg1, bool priv,
|
|
struct nouveau_channel **);
|
|
void nouveau_channel_del(struct nouveau_channel **);
|
|
int nouveau_channel_idle(struct nouveau_channel *);
|
|
|
|
extern int nouveau_vram_pushbuf;
|
|
|
|
#endif
|