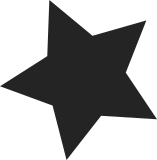
Currently the reclaim code for the case where we don't reclaim the final reclaim is overly complicated. We know that the inode is clean but instead of just directly reclaiming the clean inode we go through the whole process of marking the inode reclaimable just to directly reclaim it from the calling context. Besides being overly complicated this introduces a race where iget could recycle an inode between marked reclaimable and actually being reclaimed leading to panics. This patch gets rid of the existing reclaim path, and replaces it with a simple call to xfs_ireclaim if the inode was clean. While we're at it we also use the slightly more lax xfs_inode_clean check we'd use later to determine if we need to flush the inode here. Finally get rid of xfs_reclaim function and place the remaining small bits of reclaim code directly into xfs_fs_destroy_inode. Signed-off-by: Christoph Hellwig <hch@lst.de> Reported-by: Patrick Schreurs <patrick@news-service.com> Reported-by: Tommy van Leeuwen <tommy@news-service.com> Tested-by: Patrick Schreurs <patrick@news-service.com> Reviewed-by: Alex Elder <aelder@sgi.com> Signed-off-by: Alex Elder <aelder@sgi.com>
76 lines
3.2 KiB
C
76 lines
3.2 KiB
C
#ifndef _XFS_VNODEOPS_H
|
|
#define _XFS_VNODEOPS_H 1
|
|
|
|
struct attrlist_cursor_kern;
|
|
struct cred;
|
|
struct file;
|
|
struct iattr;
|
|
struct inode;
|
|
struct iovec;
|
|
struct kiocb;
|
|
struct pipe_inode_info;
|
|
struct uio;
|
|
struct xfs_inode;
|
|
struct xfs_iomap;
|
|
|
|
|
|
int xfs_setattr(struct xfs_inode *ip, struct iattr *vap, int flags);
|
|
#define XFS_ATTR_DMI 0x01 /* invocation from a DMI function */
|
|
#define XFS_ATTR_NONBLOCK 0x02 /* return EAGAIN if operation would block */
|
|
#define XFS_ATTR_NOLOCK 0x04 /* Don't grab any conflicting locks */
|
|
#define XFS_ATTR_NOACL 0x08 /* Don't call xfs_acl_chmod */
|
|
|
|
int xfs_readlink(struct xfs_inode *ip, char *link);
|
|
int xfs_fsync(struct xfs_inode *ip);
|
|
int xfs_release(struct xfs_inode *ip);
|
|
int xfs_inactive(struct xfs_inode *ip);
|
|
int xfs_lookup(struct xfs_inode *dp, struct xfs_name *name,
|
|
struct xfs_inode **ipp, struct xfs_name *ci_name);
|
|
int xfs_create(struct xfs_inode *dp, struct xfs_name *name, mode_t mode,
|
|
xfs_dev_t rdev, struct xfs_inode **ipp, cred_t *credp);
|
|
int xfs_remove(struct xfs_inode *dp, struct xfs_name *name,
|
|
struct xfs_inode *ip);
|
|
int xfs_link(struct xfs_inode *tdp, struct xfs_inode *sip,
|
|
struct xfs_name *target_name);
|
|
int xfs_readdir(struct xfs_inode *dp, void *dirent, size_t bufsize,
|
|
xfs_off_t *offset, filldir_t filldir);
|
|
int xfs_symlink(struct xfs_inode *dp, struct xfs_name *link_name,
|
|
const char *target_path, mode_t mode, struct xfs_inode **ipp,
|
|
cred_t *credp);
|
|
int xfs_set_dmattrs(struct xfs_inode *ip, u_int evmask, u_int16_t state);
|
|
int xfs_change_file_space(struct xfs_inode *ip, int cmd,
|
|
xfs_flock64_t *bf, xfs_off_t offset, int attr_flags);
|
|
int xfs_rename(struct xfs_inode *src_dp, struct xfs_name *src_name,
|
|
struct xfs_inode *src_ip, struct xfs_inode *target_dp,
|
|
struct xfs_name *target_name, struct xfs_inode *target_ip);
|
|
int xfs_attr_get(struct xfs_inode *ip, const char *name, char *value,
|
|
int *valuelenp, int flags);
|
|
int xfs_attr_set(struct xfs_inode *dp, const char *name, char *value,
|
|
int valuelen, int flags);
|
|
int xfs_attr_remove(struct xfs_inode *dp, const char *name, int flags);
|
|
int xfs_attr_list(struct xfs_inode *dp, char *buffer, int bufsize,
|
|
int flags, struct attrlist_cursor_kern *cursor);
|
|
ssize_t xfs_read(struct xfs_inode *ip, struct kiocb *iocb,
|
|
const struct iovec *iovp, unsigned int segs,
|
|
loff_t *offset, int ioflags);
|
|
ssize_t xfs_splice_read(struct xfs_inode *ip, struct file *infilp,
|
|
loff_t *ppos, struct pipe_inode_info *pipe, size_t count,
|
|
int flags, int ioflags);
|
|
ssize_t xfs_splice_write(struct xfs_inode *ip,
|
|
struct pipe_inode_info *pipe, struct file *outfilp,
|
|
loff_t *ppos, size_t count, int flags, int ioflags);
|
|
ssize_t xfs_write(struct xfs_inode *xip, struct kiocb *iocb,
|
|
const struct iovec *iovp, unsigned int nsegs,
|
|
loff_t *offset, int ioflags);
|
|
int xfs_bmap(struct xfs_inode *ip, xfs_off_t offset, ssize_t count,
|
|
int flags, struct xfs_iomap *iomapp, int *niomaps);
|
|
void xfs_tosspages(struct xfs_inode *inode, xfs_off_t first,
|
|
xfs_off_t last, int fiopt);
|
|
int xfs_flushinval_pages(struct xfs_inode *ip, xfs_off_t first,
|
|
xfs_off_t last, int fiopt);
|
|
int xfs_flush_pages(struct xfs_inode *ip, xfs_off_t first,
|
|
xfs_off_t last, uint64_t flags, int fiopt);
|
|
int xfs_wait_on_pages(struct xfs_inode *ip, xfs_off_t first, xfs_off_t last);
|
|
|
|
#endif /* _XFS_VNODEOPS_H */
|