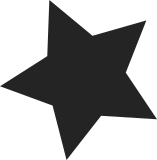
The changes introduced to allow rxrpc calls to be retried creates an issue
when it comes to refcounting afs_call structs. The problem is that when
rxrpc_send_data() queues the last packet for an asynchronous call, the
following sequence can occur:
(1) The notify_end_tx callback is invoked which causes the state in the
afs_call to be changed from AFS_CALL_CL_REQUESTING or
AFS_CALL_SV_REPLYING.
(2) afs_deliver_to_call() can then process event notifications from rxrpc
on the async_work queue.
(3) Delivery of events, such as an abort from the server, can cause the
afs_call state to be changed to AFS_CALL_COMPLETE on async_work.
(4) For an asynchronous call, afs_process_async_call() notes that the call
is complete and tried to clean up all the refs on async_work.
(5) rxrpc_send_data() might return the amount of data transferred
(success) or an error - which could in turn reflect a local error or a
received error.
Synchronising the clean up after rxrpc_kernel_send_data() returns an error
with the asynchronous cleanup is then tricky to get right.
Mostly revert commit c038a58ccf
. The two API
functions the original commit added aren't currently used. This makes
rxrpc_kernel_send_data() always return successfully if it queued the data
it was given.
Note that this doesn't affect synchronous calls since their Rx notification
function merely pokes a wait queue and does not refcounting. The
asynchronous call notification function *has* to do refcounting and pass a
ref over the work item to avoid the need to sync the workqueue in call
cleanup.
Signed-off-by: David Howells <dhowells@redhat.com>
Signed-off-by: David S. Miller <davem@davemloft.net>
71 lines
2.5 KiB
C
71 lines
2.5 KiB
C
/* RxRPC kernel service interface definitions
|
|
*
|
|
* Copyright (C) 2007 Red Hat, Inc. All Rights Reserved.
|
|
* Written by David Howells (dhowells@redhat.com)
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version
|
|
* 2 of the License, or (at your option) any later version.
|
|
*/
|
|
|
|
#ifndef _NET_RXRPC_H
|
|
#define _NET_RXRPC_H
|
|
|
|
#include <linux/rxrpc.h>
|
|
#include <linux/ktime.h>
|
|
|
|
struct key;
|
|
struct sock;
|
|
struct socket;
|
|
struct rxrpc_call;
|
|
|
|
/*
|
|
* Debug ID counter for tracing.
|
|
*/
|
|
extern atomic_t rxrpc_debug_id;
|
|
|
|
typedef void (*rxrpc_notify_rx_t)(struct sock *, struct rxrpc_call *,
|
|
unsigned long);
|
|
typedef void (*rxrpc_notify_end_tx_t)(struct sock *, struct rxrpc_call *,
|
|
unsigned long);
|
|
typedef void (*rxrpc_notify_new_call_t)(struct sock *, struct rxrpc_call *,
|
|
unsigned long);
|
|
typedef void (*rxrpc_discard_new_call_t)(struct rxrpc_call *, unsigned long);
|
|
typedef void (*rxrpc_user_attach_call_t)(struct rxrpc_call *, unsigned long);
|
|
|
|
void rxrpc_kernel_new_call_notification(struct socket *,
|
|
rxrpc_notify_new_call_t,
|
|
rxrpc_discard_new_call_t);
|
|
struct rxrpc_call *rxrpc_kernel_begin_call(struct socket *,
|
|
struct sockaddr_rxrpc *,
|
|
struct key *,
|
|
unsigned long,
|
|
s64,
|
|
gfp_t,
|
|
rxrpc_notify_rx_t,
|
|
bool,
|
|
unsigned int);
|
|
int rxrpc_kernel_send_data(struct socket *, struct rxrpc_call *,
|
|
struct msghdr *, size_t,
|
|
rxrpc_notify_end_tx_t);
|
|
int rxrpc_kernel_recv_data(struct socket *, struct rxrpc_call *,
|
|
struct iov_iter *, bool, u32 *, u16 *);
|
|
bool rxrpc_kernel_abort_call(struct socket *, struct rxrpc_call *,
|
|
u32, int, const char *);
|
|
void rxrpc_kernel_end_call(struct socket *, struct rxrpc_call *);
|
|
void rxrpc_kernel_get_peer(struct socket *, struct rxrpc_call *,
|
|
struct sockaddr_rxrpc *);
|
|
u64 rxrpc_kernel_get_rtt(struct socket *, struct rxrpc_call *);
|
|
int rxrpc_kernel_charge_accept(struct socket *, rxrpc_notify_rx_t,
|
|
rxrpc_user_attach_call_t, unsigned long, gfp_t,
|
|
unsigned int);
|
|
void rxrpc_kernel_set_tx_length(struct socket *, struct rxrpc_call *, s64);
|
|
u32 rxrpc_kernel_check_life(const struct socket *, const struct rxrpc_call *);
|
|
void rxrpc_kernel_probe_life(struct socket *, struct rxrpc_call *);
|
|
u32 rxrpc_kernel_get_epoch(struct socket *, struct rxrpc_call *);
|
|
bool rxrpc_kernel_get_reply_time(struct socket *, struct rxrpc_call *,
|
|
ktime_t *);
|
|
|
|
#endif /* _NET_RXRPC_H */
|