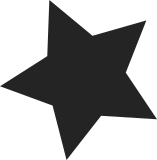
This reverts commit 5163fb6254
("ahci: added support for Freescale AHCI sata")
The reverted patch added Freescale QorIQ AHCI sata support to
ahci_platform driver though, but it left SoC specific settings to uboot.
It leads to QorIQ sata heavily depending on uboot. In order to removing
the dependency we first revert the old patch and then will add a new driver
for QorIQ SATA.
Since there are no LS* platforms that have been upstreamed, So
the revert would not break anything exists.
Signed-off-by: Tang Yuantian <Yuantian.Tang@freescale.com>
Reviewed-by: Hans de Goede <hdegoede@redhat.com>
Signed-off-by: Tejun Heo <tj@kernel.org>
105 lines
2.7 KiB
C
105 lines
2.7 KiB
C
/*
|
|
* AHCI SATA platform driver
|
|
*
|
|
* Copyright 2004-2005 Red Hat, Inc.
|
|
* Jeff Garzik <jgarzik@pobox.com>
|
|
* Copyright 2010 MontaVista Software, LLC.
|
|
* Anton Vorontsov <avorontsov@ru.mvista.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2, or (at your option)
|
|
* any later version.
|
|
*/
|
|
|
|
#include <linux/kernel.h>
|
|
#include <linux/module.h>
|
|
#include <linux/pm.h>
|
|
#include <linux/device.h>
|
|
#include <linux/of_device.h>
|
|
#include <linux/platform_device.h>
|
|
#include <linux/libata.h>
|
|
#include <linux/ahci_platform.h>
|
|
#include <linux/acpi.h>
|
|
#include <linux/pci_ids.h>
|
|
#include "ahci.h"
|
|
|
|
#define DRV_NAME "ahci"
|
|
|
|
static const struct ata_port_info ahci_port_info = {
|
|
.flags = AHCI_FLAG_COMMON,
|
|
.pio_mask = ATA_PIO4,
|
|
.udma_mask = ATA_UDMA6,
|
|
.port_ops = &ahci_platform_ops,
|
|
};
|
|
|
|
static struct scsi_host_template ahci_platform_sht = {
|
|
AHCI_SHT(DRV_NAME),
|
|
};
|
|
|
|
static int ahci_probe(struct platform_device *pdev)
|
|
{
|
|
struct device *dev = &pdev->dev;
|
|
struct ahci_host_priv *hpriv;
|
|
int rc;
|
|
|
|
hpriv = ahci_platform_get_resources(pdev);
|
|
if (IS_ERR(hpriv))
|
|
return PTR_ERR(hpriv);
|
|
|
|
rc = ahci_platform_enable_resources(hpriv);
|
|
if (rc)
|
|
return rc;
|
|
|
|
if (of_device_is_compatible(dev->of_node, "hisilicon,hisi-ahci"))
|
|
hpriv->flags |= AHCI_HFLAG_NO_FBS | AHCI_HFLAG_NO_NCQ;
|
|
|
|
rc = ahci_platform_init_host(pdev, hpriv, &ahci_port_info,
|
|
&ahci_platform_sht);
|
|
if (rc)
|
|
goto disable_resources;
|
|
|
|
return 0;
|
|
disable_resources:
|
|
ahci_platform_disable_resources(hpriv);
|
|
return rc;
|
|
}
|
|
|
|
static SIMPLE_DEV_PM_OPS(ahci_pm_ops, ahci_platform_suspend,
|
|
ahci_platform_resume);
|
|
|
|
static const struct of_device_id ahci_of_match[] = {
|
|
{ .compatible = "generic-ahci", },
|
|
/* Keep the following compatibles for device tree compatibility */
|
|
{ .compatible = "snps,spear-ahci", },
|
|
{ .compatible = "snps,exynos5440-ahci", },
|
|
{ .compatible = "ibm,476gtr-ahci", },
|
|
{ .compatible = "snps,dwc-ahci", },
|
|
{ .compatible = "hisilicon,hisi-ahci", },
|
|
{},
|
|
};
|
|
MODULE_DEVICE_TABLE(of, ahci_of_match);
|
|
|
|
static const struct acpi_device_id ahci_acpi_match[] = {
|
|
{ ACPI_DEVICE_CLASS(PCI_CLASS_STORAGE_SATA_AHCI, 0xffffff) },
|
|
{},
|
|
};
|
|
MODULE_DEVICE_TABLE(acpi, ahci_acpi_match);
|
|
|
|
static struct platform_driver ahci_driver = {
|
|
.probe = ahci_probe,
|
|
.remove = ata_platform_remove_one,
|
|
.driver = {
|
|
.name = DRV_NAME,
|
|
.of_match_table = ahci_of_match,
|
|
.acpi_match_table = ahci_acpi_match,
|
|
.pm = &ahci_pm_ops,
|
|
},
|
|
};
|
|
module_platform_driver(ahci_driver);
|
|
|
|
MODULE_DESCRIPTION("AHCI SATA platform driver");
|
|
MODULE_AUTHOR("Anton Vorontsov <avorontsov@ru.mvista.com>");
|
|
MODULE_LICENSE("GPL");
|
|
MODULE_ALIAS("platform:ahci");
|