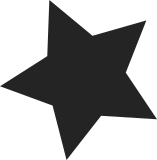
put_cpu_no_resched() is an optimization of put_cpu() which unfortunately can cause high latencies. The nfs iostats code uses put_cpu_no_resched() in a code sequence where a reschedule request caused by an interrupt between the get_cpu() and the put_cpu_no_resched() can delay the reschedule for at least HZ. The other users of put_cpu_no_resched() optimize correctly in interrupt code, but there is no real harm in using the put_cpu() function which is an alias for preempt_enable(). The extra check of the preemmpt count is not as critical as the potential source of missing a reschedule. Debugged in the preempt-rt tree and verified in mainline. Impact: remove a high latency source [akpm@linux-foundation.org: build fix] Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Acked-by: Ingo Molnar <mingo@elte.hu> Cc: Tony Luck <tony.luck@intel.com> Cc: Trond Myklebust <Trond.Myklebust@netapp.com> Cc: "J. Bruce Fields" <bfields@fieldses.org> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
90 lines
1.9 KiB
C
90 lines
1.9 KiB
C
/*
|
|
* linux/fs/nfs/iostat.h
|
|
*
|
|
* Declarations for NFS client per-mount statistics
|
|
*
|
|
* Copyright (C) 2005, 2006 Chuck Lever <cel@netapp.com>
|
|
*
|
|
*/
|
|
|
|
#ifndef _NFS_IOSTAT
|
|
#define _NFS_IOSTAT
|
|
|
|
#include <linux/percpu.h>
|
|
#include <linux/cache.h>
|
|
#include <linux/nfs_iostat.h>
|
|
|
|
struct nfs_iostats {
|
|
unsigned long long bytes[__NFSIOS_BYTESMAX];
|
|
#ifdef CONFIG_NFS_FSCACHE
|
|
unsigned long long fscache[__NFSIOS_FSCACHEMAX];
|
|
#endif
|
|
unsigned long events[__NFSIOS_COUNTSMAX];
|
|
} ____cacheline_aligned;
|
|
|
|
static inline void nfs_inc_server_stats(const struct nfs_server *server,
|
|
enum nfs_stat_eventcounters stat)
|
|
{
|
|
struct nfs_iostats *iostats;
|
|
int cpu;
|
|
|
|
cpu = get_cpu();
|
|
iostats = per_cpu_ptr(server->io_stats, cpu);
|
|
iostats->events[stat]++;
|
|
put_cpu();
|
|
}
|
|
|
|
static inline void nfs_inc_stats(const struct inode *inode,
|
|
enum nfs_stat_eventcounters stat)
|
|
{
|
|
nfs_inc_server_stats(NFS_SERVER(inode), stat);
|
|
}
|
|
|
|
static inline void nfs_add_server_stats(const struct nfs_server *server,
|
|
enum nfs_stat_bytecounters stat,
|
|
unsigned long addend)
|
|
{
|
|
struct nfs_iostats *iostats;
|
|
int cpu;
|
|
|
|
cpu = get_cpu();
|
|
iostats = per_cpu_ptr(server->io_stats, cpu);
|
|
iostats->bytes[stat] += addend;
|
|
put_cpu();
|
|
}
|
|
|
|
static inline void nfs_add_stats(const struct inode *inode,
|
|
enum nfs_stat_bytecounters stat,
|
|
unsigned long addend)
|
|
{
|
|
nfs_add_server_stats(NFS_SERVER(inode), stat, addend);
|
|
}
|
|
|
|
#ifdef CONFIG_NFS_FSCACHE
|
|
static inline void nfs_add_fscache_stats(struct inode *inode,
|
|
enum nfs_stat_fscachecounters stat,
|
|
unsigned long addend)
|
|
{
|
|
struct nfs_iostats *iostats;
|
|
int cpu;
|
|
|
|
cpu = get_cpu();
|
|
iostats = per_cpu_ptr(NFS_SERVER(inode)->io_stats, cpu);
|
|
iostats->fscache[stat] += addend;
|
|
put_cpu();
|
|
}
|
|
#endif
|
|
|
|
static inline struct nfs_iostats *nfs_alloc_iostats(void)
|
|
{
|
|
return alloc_percpu(struct nfs_iostats);
|
|
}
|
|
|
|
static inline void nfs_free_iostats(struct nfs_iostats *stats)
|
|
{
|
|
if (stats != NULL)
|
|
free_percpu(stats);
|
|
}
|
|
|
|
#endif /* _NFS_IOSTAT */
|