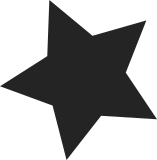
Some versions of MCFW do not support the MC_CMD_VADAPTOR_SET_MAC command, and ENOSYS will be returned. If the PF created its own vport, the function's datapath must be stopped and the vport can be reconfigured to reflect the new MAC address. If the MCFW created the vport for the PF (which is the case when the nic_data->vport_mac is blank), nothing further needs to be done as the vport is not under the control of the PF. This only applies to PFs because the MCFW in question does not support VFs. Signed-off-by: Shradha Shah <sshah@solarflare.com> Signed-off-by: David S. Miller <davem@davemloft.net>
76 lines
2.6 KiB
C
76 lines
2.6 KiB
C
/****************************************************************************
|
|
* Driver for Solarflare network controllers and boards
|
|
* Copyright 2015 Solarflare Communications Inc.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License version 2 as published
|
|
* by the Free Software Foundation, incorporated herein by reference.
|
|
*/
|
|
|
|
#ifndef EF10_SRIOV_H
|
|
#define EF10_SRIOV_H
|
|
|
|
#include "net_driver.h"
|
|
|
|
/**
|
|
* struct ef10_vf - PF's store of VF data
|
|
* @efx: efx_nic struct for the current VF
|
|
* @pci_dev: the pci_dev struct for the VF, retained while the VF is assigned
|
|
* @vport_id: vport ID for the VF
|
|
* @vport_assigned: record whether the vport is currently assigned to the VF
|
|
* @mac: MAC address for the VF, zero when address is removed from the vport
|
|
* @vlan: Default VLAN for the VF or #EFX_EF10_NO_VLAN
|
|
*/
|
|
struct ef10_vf {
|
|
struct efx_nic *efx;
|
|
struct pci_dev *pci_dev;
|
|
unsigned int vport_id;
|
|
unsigned int vport_assigned;
|
|
u8 mac[ETH_ALEN];
|
|
u16 vlan;
|
|
#define EFX_EF10_NO_VLAN 0
|
|
};
|
|
|
|
static inline bool efx_ef10_sriov_wanted(struct efx_nic *efx)
|
|
{
|
|
return false;
|
|
}
|
|
|
|
int efx_ef10_sriov_configure(struct efx_nic *efx, int num_vfs);
|
|
int efx_ef10_sriov_init(struct efx_nic *efx);
|
|
static inline void efx_ef10_sriov_reset(struct efx_nic *efx) {}
|
|
void efx_ef10_sriov_fini(struct efx_nic *efx);
|
|
static inline void efx_ef10_sriov_flr(struct efx_nic *efx, unsigned vf_i) {}
|
|
|
|
int efx_ef10_sriov_set_vf_mac(struct efx_nic *efx, int vf, u8 *mac);
|
|
|
|
int efx_ef10_sriov_set_vf_vlan(struct efx_nic *efx, int vf_i,
|
|
u16 vlan, u8 qos);
|
|
|
|
int efx_ef10_sriov_set_vf_spoofchk(struct efx_nic *efx, int vf,
|
|
bool spoofchk);
|
|
|
|
int efx_ef10_sriov_get_vf_config(struct efx_nic *efx, int vf_i,
|
|
struct ifla_vf_info *ivf);
|
|
|
|
int efx_ef10_sriov_set_vf_link_state(struct efx_nic *efx, int vf_i,
|
|
int link_state);
|
|
|
|
int efx_ef10_sriov_get_phys_port_id(struct efx_nic *efx,
|
|
struct netdev_phys_item_id *ppid);
|
|
|
|
int efx_ef10_vswitching_probe_pf(struct efx_nic *efx);
|
|
int efx_ef10_vswitching_probe_vf(struct efx_nic *efx);
|
|
int efx_ef10_vswitching_restore_pf(struct efx_nic *efx);
|
|
int efx_ef10_vswitching_restore_vf(struct efx_nic *efx);
|
|
void efx_ef10_vswitching_remove_pf(struct efx_nic *efx);
|
|
void efx_ef10_vswitching_remove_vf(struct efx_nic *efx);
|
|
int efx_ef10_vport_add_mac(struct efx_nic *efx,
|
|
unsigned int port_id, u8 *mac);
|
|
int efx_ef10_vport_del_mac(struct efx_nic *efx,
|
|
unsigned int port_id, u8 *mac);
|
|
int efx_ef10_vadaptor_alloc(struct efx_nic *efx, unsigned int port_id);
|
|
int efx_ef10_vadaptor_free(struct efx_nic *efx, unsigned int port_id);
|
|
|
|
#endif /* EF10_SRIOV_H */
|