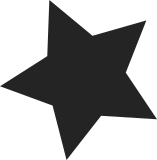
structures. Always use the generic xfs_btree_block type instead of the short / long structures. Add XFS_BTREE_SBLOCK_LEN / XFS_BTREE_LBLOCK_LEN defines for the length of a short / long form block. The rationale for this is that we will grow more btree block header variants to support CRCs and other RAS information, and always accessing them through the same datatype with unions for the short / long form pointers makes implementing this much easier. SGI-PV: 988146 SGI-Modid: xfs-linux-melb:xfs-kern:32300a Signed-off-by: Christoph Hellwig <hch@infradead.org> Signed-off-by: Donald Douwsma <donaldd@sgi.com> Signed-off-by: David Chinner <david@fromorbit.com> Signed-off-by: Lachlan McIlroy <lachlan@sgi.com>
111 lines
3.5 KiB
C
111 lines
3.5 KiB
C
/*
|
|
* Copyright (c) 2000,2005 Silicon Graphics, Inc.
|
|
* All Rights Reserved.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it would be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write the Free Software Foundation,
|
|
* Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
|
|
*/
|
|
#ifndef __XFS_ALLOC_BTREE_H__
|
|
#define __XFS_ALLOC_BTREE_H__
|
|
|
|
/*
|
|
* Freespace on-disk structures
|
|
*/
|
|
|
|
struct xfs_buf;
|
|
struct xfs_btree_cur;
|
|
struct xfs_mount;
|
|
|
|
/*
|
|
* There are two on-disk btrees, one sorted by blockno and one sorted
|
|
* by blockcount and blockno. All blocks look the same to make the code
|
|
* simpler; if we have time later, we'll make the optimizations.
|
|
*/
|
|
#define XFS_ABTB_MAGIC 0x41425442 /* 'ABTB' for bno tree */
|
|
#define XFS_ABTC_MAGIC 0x41425443 /* 'ABTC' for cnt tree */
|
|
|
|
/*
|
|
* Data record/key structure
|
|
*/
|
|
typedef struct xfs_alloc_rec {
|
|
__be32 ar_startblock; /* starting block number */
|
|
__be32 ar_blockcount; /* count of free blocks */
|
|
} xfs_alloc_rec_t, xfs_alloc_key_t;
|
|
|
|
typedef struct xfs_alloc_rec_incore {
|
|
xfs_agblock_t ar_startblock; /* starting block number */
|
|
xfs_extlen_t ar_blockcount; /* count of free blocks */
|
|
} xfs_alloc_rec_incore_t;
|
|
|
|
/* btree pointer type */
|
|
typedef __be32 xfs_alloc_ptr_t;
|
|
|
|
/*
|
|
* Minimum and maximum blocksize and sectorsize.
|
|
* The blocksize upper limit is pretty much arbitrary.
|
|
* The sectorsize upper limit is due to sizeof(sb_sectsize).
|
|
*/
|
|
#define XFS_MIN_BLOCKSIZE_LOG 9 /* i.e. 512 bytes */
|
|
#define XFS_MAX_BLOCKSIZE_LOG 16 /* i.e. 65536 bytes */
|
|
#define XFS_MIN_BLOCKSIZE (1 << XFS_MIN_BLOCKSIZE_LOG)
|
|
#define XFS_MAX_BLOCKSIZE (1 << XFS_MAX_BLOCKSIZE_LOG)
|
|
#define XFS_MIN_SECTORSIZE_LOG 9 /* i.e. 512 bytes */
|
|
#define XFS_MAX_SECTORSIZE_LOG 15 /* i.e. 32768 bytes */
|
|
#define XFS_MIN_SECTORSIZE (1 << XFS_MIN_SECTORSIZE_LOG)
|
|
#define XFS_MAX_SECTORSIZE (1 << XFS_MAX_SECTORSIZE_LOG)
|
|
|
|
/*
|
|
* Block numbers in the AG:
|
|
* SB is sector 0, AGF is sector 1, AGI is sector 2, AGFL is sector 3.
|
|
*/
|
|
#define XFS_BNO_BLOCK(mp) ((xfs_agblock_t)(XFS_AGFL_BLOCK(mp) + 1))
|
|
#define XFS_CNT_BLOCK(mp) ((xfs_agblock_t)(XFS_BNO_BLOCK(mp) + 1))
|
|
|
|
/*
|
|
* Btree block header size depends on a superblock flag.
|
|
*
|
|
* (not quite yet, but soon)
|
|
*/
|
|
#define XFS_ALLOC_BLOCK_LEN(mp) XFS_BTREE_SBLOCK_LEN
|
|
|
|
/*
|
|
* Record, key, and pointer address macros for btree blocks.
|
|
*
|
|
* (note that some of these may appear unused, but they are used in userspace)
|
|
*/
|
|
#define XFS_ALLOC_REC_ADDR(mp, block, index) \
|
|
((xfs_alloc_rec_t *) \
|
|
((char *)(block) + \
|
|
XFS_ALLOC_BLOCK_LEN(mp) + \
|
|
(((index) - 1) * sizeof(xfs_alloc_rec_t))))
|
|
|
|
#define XFS_ALLOC_KEY_ADDR(mp, block, index) \
|
|
((xfs_alloc_key_t *) \
|
|
((char *)(block) + \
|
|
XFS_ALLOC_BLOCK_LEN(mp) + \
|
|
((index) - 1) * sizeof(xfs_alloc_key_t)))
|
|
|
|
#define XFS_ALLOC_PTR_ADDR(mp, block, index, maxrecs) \
|
|
((xfs_alloc_ptr_t *) \
|
|
((char *)(block) + \
|
|
XFS_ALLOC_BLOCK_LEN(mp) + \
|
|
(maxrecs) * sizeof(xfs_alloc_key_t) + \
|
|
((index) - 1) * sizeof(xfs_alloc_ptr_t)))
|
|
|
|
extern struct xfs_btree_cur *xfs_allocbt_init_cursor(struct xfs_mount *,
|
|
struct xfs_trans *, struct xfs_buf *,
|
|
xfs_agnumber_t, xfs_btnum_t);
|
|
extern int xfs_allocbt_maxrecs(struct xfs_mount *, int, int);
|
|
|
|
#endif /* __XFS_ALLOC_BTREE_H__ */
|