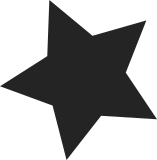
There are two reasons for this fix: - the result of choose_orig() and vis_choose() is an index and therefore it can't be negative. Hence it is correct to make the return type unsigned too. - sizeof(int) may not be the same on ALL the architectures. Since we plan to use choose_orig() as DHT hash function, we need to guarantee that, given the same argument, the result is the same. Then it is correct to explicitly express the size of the return type (and the second argument). Since the expected length is currently 4, uint32_t is the most convenient choice. Signed-off-by: Antonio Quartulli <ordex@autistici.org> Signed-off-by: Sven Eckelmann <sven@narfation.org>
72 lines
1.7 KiB
C
72 lines
1.7 KiB
C
/*
|
|
* Copyright (C) 2006-2011 B.A.T.M.A.N. contributors:
|
|
*
|
|
* Simon Wunderlich, Marek Lindner
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of version 2 of the GNU General Public
|
|
* License as published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but
|
|
* WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
|
|
* 02110-1301, USA
|
|
*
|
|
*/
|
|
|
|
#include "main.h"
|
|
#include "hash.h"
|
|
|
|
/* clears the hash */
|
|
static void hash_init(struct hashtable_t *hash)
|
|
{
|
|
uint32_t i;
|
|
|
|
for (i = 0 ; i < hash->size; i++) {
|
|
INIT_HLIST_HEAD(&hash->table[i]);
|
|
spin_lock_init(&hash->list_locks[i]);
|
|
}
|
|
}
|
|
|
|
/* free only the hashtable and the hash itself. */
|
|
void hash_destroy(struct hashtable_t *hash)
|
|
{
|
|
kfree(hash->list_locks);
|
|
kfree(hash->table);
|
|
kfree(hash);
|
|
}
|
|
|
|
/* allocates and clears the hash */
|
|
struct hashtable_t *hash_new(uint32_t size)
|
|
{
|
|
struct hashtable_t *hash;
|
|
|
|
hash = kmalloc(sizeof(*hash), GFP_ATOMIC);
|
|
if (!hash)
|
|
return NULL;
|
|
|
|
hash->table = kmalloc(sizeof(*hash->table) * size, GFP_ATOMIC);
|
|
if (!hash->table)
|
|
goto free_hash;
|
|
|
|
hash->list_locks = kmalloc(sizeof(*hash->list_locks) * size,
|
|
GFP_ATOMIC);
|
|
if (!hash->list_locks)
|
|
goto free_table;
|
|
|
|
hash->size = size;
|
|
hash_init(hash);
|
|
return hash;
|
|
|
|
free_table:
|
|
kfree(hash->table);
|
|
free_hash:
|
|
kfree(hash);
|
|
return NULL;
|
|
}
|