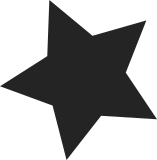
Instead of __raw_*, define imx_* to *_relaxed and use those. Using imx_* was requested by Arnd because *_relaxed tends to indicate that the code was carefully reviewed to not require any synchronisation and otherwise be safe, which isn't the case here with the automatic conversion. The conversion itself was done using the following spatch (since that automatically adjusts the coding style unlike a simple search&replace). @@ expression E1, E2; @@ -__raw_writel(E1, E2) +imx_writel(E1, E2) @@ expression E1, E2; @@ -__raw_writew(E1, E2) +imx_writew(E1, E2) @@ expression E1; @@ -__raw_readl(E1) +imx_readl(E1) @@ expression E1; @@ -__raw_readw(E1) +imx_readw(E1) Signed-off-by: Johannes Berg <johannes@sipsolutions.net> Signed-off-by: Shawn Guo <shawnguo@kernel.org>
44 lines
925 B
C
44 lines
925 B
C
/*
|
|
* MX35 CPU type detection
|
|
*
|
|
* Copyright (c) 2009 Daniel Mack <daniel@caiaq.de>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*/
|
|
#include <linux/module.h>
|
|
#include <linux/io.h>
|
|
|
|
#include "hardware.h"
|
|
#include "iim.h"
|
|
|
|
static int mx35_cpu_rev = -1;
|
|
|
|
static int mx35_read_cpu_rev(void)
|
|
{
|
|
u32 rev;
|
|
|
|
rev = imx_readl(MX35_IO_ADDRESS(MX35_IIM_BASE_ADDR + MXC_IIMSREV));
|
|
switch (rev) {
|
|
case 0x00:
|
|
return IMX_CHIP_REVISION_1_0;
|
|
case 0x10:
|
|
return IMX_CHIP_REVISION_2_0;
|
|
case 0x11:
|
|
return IMX_CHIP_REVISION_2_1;
|
|
default:
|
|
return IMX_CHIP_REVISION_UNKNOWN;
|
|
}
|
|
}
|
|
|
|
int mx35_revision(void)
|
|
{
|
|
if (mx35_cpu_rev == -1)
|
|
mx35_cpu_rev = mx35_read_cpu_rev();
|
|
|
|
return mx35_cpu_rev;
|
|
}
|
|
EXPORT_SYMBOL(mx35_revision);
|