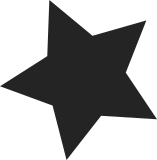
This patch (as1564c) converts the EHCI platform drivers to use the central ehci_setup() routine for generic controller initialization rather than each having its own idiosyncratic approach. The major point of difficulty lies in ehci-pci's many vendor- and device-specific workarounds. Some of them have to be applied before calling ehci_setup() and some after, which necessitates a fair amount of code motion. The other platform drivers require much smaller changes. One point not addressed by the patch is whether ports should be powered on or off following initialization. The different drivers appear to handle this pretty much at random. In fact it shouldn't matter, because the hub driver turns on power to all ports when it binds to the root hub. Straightening that out will be left for another day. Signed-off-by: Alan Stern <stern@rowland.harvard.edu> Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
153 lines
3.5 KiB
C
153 lines
3.5 KiB
C
/*
|
|
* IXP4XX EHCI Host Controller Driver
|
|
*
|
|
* Author: Vladimir Barinov <vbarinov@embeddedalley.com>
|
|
*
|
|
* Based on "ehci-fsl.c" by Randy Vinson <rvinson@mvista.com>
|
|
*
|
|
* 2007 (c) MontaVista Software, Inc. This file is licensed under
|
|
* the terms of the GNU General Public License version 2. This program
|
|
* is licensed "as is" without any warranty of any kind, whether express
|
|
* or implied.
|
|
*/
|
|
|
|
#include <linux/platform_device.h>
|
|
|
|
static int ixp4xx_ehci_init(struct usb_hcd *hcd)
|
|
{
|
|
struct ehci_hcd *ehci = hcd_to_ehci(hcd);
|
|
int retval = 0;
|
|
|
|
ehci->big_endian_desc = 1;
|
|
ehci->big_endian_mmio = 1;
|
|
|
|
ehci->caps = hcd->regs + 0x100;
|
|
|
|
hcd->has_tt = 1;
|
|
|
|
retval = ehci_setup(hcd);
|
|
if (retval)
|
|
return retval;
|
|
|
|
ehci_port_power(ehci, 0);
|
|
|
|
return retval;
|
|
}
|
|
|
|
static const struct hc_driver ixp4xx_ehci_hc_driver = {
|
|
.description = hcd_name,
|
|
.product_desc = "IXP4XX EHCI Host Controller",
|
|
.hcd_priv_size = sizeof(struct ehci_hcd),
|
|
.irq = ehci_irq,
|
|
.flags = HCD_MEMORY | HCD_USB2,
|
|
.reset = ixp4xx_ehci_init,
|
|
.start = ehci_run,
|
|
.stop = ehci_stop,
|
|
.shutdown = ehci_shutdown,
|
|
.urb_enqueue = ehci_urb_enqueue,
|
|
.urb_dequeue = ehci_urb_dequeue,
|
|
.endpoint_disable = ehci_endpoint_disable,
|
|
.endpoint_reset = ehci_endpoint_reset,
|
|
.get_frame_number = ehci_get_frame,
|
|
.hub_status_data = ehci_hub_status_data,
|
|
.hub_control = ehci_hub_control,
|
|
#if defined(CONFIG_PM)
|
|
.bus_suspend = ehci_bus_suspend,
|
|
.bus_resume = ehci_bus_resume,
|
|
#endif
|
|
.relinquish_port = ehci_relinquish_port,
|
|
.port_handed_over = ehci_port_handed_over,
|
|
|
|
.clear_tt_buffer_complete = ehci_clear_tt_buffer_complete,
|
|
};
|
|
|
|
static int ixp4xx_ehci_probe(struct platform_device *pdev)
|
|
{
|
|
struct usb_hcd *hcd;
|
|
const struct hc_driver *driver = &ixp4xx_ehci_hc_driver;
|
|
struct resource *res;
|
|
int irq;
|
|
int retval;
|
|
|
|
if (usb_disabled())
|
|
return -ENODEV;
|
|
|
|
res = platform_get_resource(pdev, IORESOURCE_IRQ, 0);
|
|
if (!res) {
|
|
dev_err(&pdev->dev,
|
|
"Found HC with no IRQ. Check %s setup!\n",
|
|
dev_name(&pdev->dev));
|
|
return -ENODEV;
|
|
}
|
|
irq = res->start;
|
|
|
|
hcd = usb_create_hcd(driver, &pdev->dev, dev_name(&pdev->dev));
|
|
if (!hcd) {
|
|
retval = -ENOMEM;
|
|
goto fail_create_hcd;
|
|
}
|
|
|
|
res = platform_get_resource(pdev, IORESOURCE_MEM, 0);
|
|
if (!res) {
|
|
dev_err(&pdev->dev,
|
|
"Found HC with no register addr. Check %s setup!\n",
|
|
dev_name(&pdev->dev));
|
|
retval = -ENODEV;
|
|
goto fail_request_resource;
|
|
}
|
|
hcd->rsrc_start = res->start;
|
|
hcd->rsrc_len = resource_size(res);
|
|
|
|
if (!request_mem_region(hcd->rsrc_start, hcd->rsrc_len,
|
|
driver->description)) {
|
|
dev_dbg(&pdev->dev, "controller already in use\n");
|
|
retval = -EBUSY;
|
|
goto fail_request_resource;
|
|
}
|
|
|
|
hcd->regs = ioremap_nocache(hcd->rsrc_start, hcd->rsrc_len);
|
|
if (hcd->regs == NULL) {
|
|
dev_dbg(&pdev->dev, "error mapping memory\n");
|
|
retval = -EFAULT;
|
|
goto fail_ioremap;
|
|
}
|
|
|
|
retval = usb_add_hcd(hcd, irq, IRQF_SHARED);
|
|
if (retval)
|
|
goto fail_add_hcd;
|
|
|
|
return retval;
|
|
|
|
fail_add_hcd:
|
|
iounmap(hcd->regs);
|
|
fail_ioremap:
|
|
release_mem_region(hcd->rsrc_start, hcd->rsrc_len);
|
|
fail_request_resource:
|
|
usb_put_hcd(hcd);
|
|
fail_create_hcd:
|
|
dev_err(&pdev->dev, "init %s fail, %d\n", dev_name(&pdev->dev), retval);
|
|
return retval;
|
|
}
|
|
|
|
static int ixp4xx_ehci_remove(struct platform_device *pdev)
|
|
{
|
|
struct usb_hcd *hcd = platform_get_drvdata(pdev);
|
|
|
|
usb_remove_hcd(hcd);
|
|
iounmap(hcd->regs);
|
|
release_mem_region(hcd->rsrc_start, hcd->rsrc_len);
|
|
usb_put_hcd(hcd);
|
|
|
|
return 0;
|
|
}
|
|
|
|
MODULE_ALIAS("platform:ixp4xx-ehci");
|
|
|
|
static struct platform_driver ixp4xx_ehci_driver = {
|
|
.probe = ixp4xx_ehci_probe,
|
|
.remove = ixp4xx_ehci_remove,
|
|
.driver = {
|
|
.name = "ixp4xx-ehci",
|
|
},
|
|
};
|