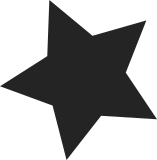
This patch (as1573) adds hrtimer support for managing ehci-hcd's periodic schedule. There are two issues to deal with. First, the schedule's state (on or off) must not be changed until the hardware status has caught up with the current command. This is handled by an hrtimer event that polls at 1-ms intervals to see when the Periodic Schedule Status (PSS) flag matches the Periodic Schedule Enable (PSE) value. Second, the schedule should not be turned off as soon as it becomes empty. Turning the schedule on and off takes time, so we want to wait until the schedule has been empty for a suitable period before turning it off. This is handled by an hrtimer event that gets set to expire 10 ms after the periodic schedule becomes empty. The existing code polls (for up to 1125 us and with interrupts disabled!) to check the status, and doesn't implement a delay before turning off the schedule. Furthermore, if the polling fails then the driver decides that the controller has died. This has caused problems for several people; some controllers can take 10 ms or more to turn off their periodic schedules. This patch fixes these issues. It also makes the "broken_periodic" workaround unnecessary; there is no longer any danger of turning off the periodic schedule after it has been on for less than 1 ms. Signed-off-by: Alan Stern <stern@rowland.harvard.edu> Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
483 lines
14 KiB
C
483 lines
14 KiB
C
/*
|
|
* EHCI HCD (Host Controller Driver) PCI Bus Glue.
|
|
*
|
|
* Copyright (c) 2000-2004 by David Brownell
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License as published by the
|
|
* Free Software Foundation; either version 2 of the License, or (at your
|
|
* option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but
|
|
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
|
|
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
|
|
* for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software Foundation,
|
|
* Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
|
|
*/
|
|
|
|
#ifndef CONFIG_PCI
|
|
#error "This file is PCI bus glue. CONFIG_PCI must be defined."
|
|
#endif
|
|
|
|
/* defined here to avoid adding to pci_ids.h for single instance use */
|
|
#define PCI_DEVICE_ID_INTEL_CE4100_USB 0x2e70
|
|
|
|
/*-------------------------------------------------------------------------*/
|
|
|
|
/* called after powerup, by probe or system-pm "wakeup" */
|
|
static int ehci_pci_reinit(struct ehci_hcd *ehci, struct pci_dev *pdev)
|
|
{
|
|
int retval;
|
|
|
|
/* we expect static quirk code to handle the "extended capabilities"
|
|
* (currently just BIOS handoff) allowed starting with EHCI 0.96
|
|
*/
|
|
|
|
/* PCI Memory-Write-Invalidate cycle support is optional (uncommon) */
|
|
retval = pci_set_mwi(pdev);
|
|
if (!retval)
|
|
ehci_dbg(ehci, "MWI active\n");
|
|
|
|
return 0;
|
|
}
|
|
|
|
/* called during probe() after chip reset completes */
|
|
static int ehci_pci_setup(struct usb_hcd *hcd)
|
|
{
|
|
struct ehci_hcd *ehci = hcd_to_ehci(hcd);
|
|
struct pci_dev *pdev = to_pci_dev(hcd->self.controller);
|
|
struct pci_dev *p_smbus;
|
|
u8 rev;
|
|
u32 temp;
|
|
int retval;
|
|
|
|
ehci->caps = hcd->regs;
|
|
|
|
/*
|
|
* ehci_init() causes memory for DMA transfers to be
|
|
* allocated. Thus, any vendor-specific workarounds based on
|
|
* limiting the type of memory used for DMA transfers must
|
|
* happen before ehci_setup() is called.
|
|
*
|
|
* Most other workarounds can be done either before or after
|
|
* init and reset; they are located here too.
|
|
*/
|
|
switch (pdev->vendor) {
|
|
case PCI_VENDOR_ID_TOSHIBA_2:
|
|
/* celleb's companion chip */
|
|
if (pdev->device == 0x01b5) {
|
|
#ifdef CONFIG_USB_EHCI_BIG_ENDIAN_MMIO
|
|
ehci->big_endian_mmio = 1;
|
|
#else
|
|
ehci_warn(ehci,
|
|
"unsupported big endian Toshiba quirk\n");
|
|
#endif
|
|
}
|
|
break;
|
|
case PCI_VENDOR_ID_NVIDIA:
|
|
/* NVidia reports that certain chips don't handle
|
|
* QH, ITD, or SITD addresses above 2GB. (But TD,
|
|
* data buffer, and periodic schedule are normal.)
|
|
*/
|
|
switch (pdev->device) {
|
|
case 0x003c: /* MCP04 */
|
|
case 0x005b: /* CK804 */
|
|
case 0x00d8: /* CK8 */
|
|
case 0x00e8: /* CK8S */
|
|
if (pci_set_consistent_dma_mask(pdev,
|
|
DMA_BIT_MASK(31)) < 0)
|
|
ehci_warn(ehci, "can't enable NVidia "
|
|
"workaround for >2GB RAM\n");
|
|
break;
|
|
|
|
/* Some NForce2 chips have problems with selective suspend;
|
|
* fixed in newer silicon.
|
|
*/
|
|
case 0x0068:
|
|
if (pdev->revision < 0xa4)
|
|
ehci->no_selective_suspend = 1;
|
|
break;
|
|
}
|
|
break;
|
|
case PCI_VENDOR_ID_INTEL:
|
|
ehci->fs_i_thresh = 1;
|
|
if (pdev->device == PCI_DEVICE_ID_INTEL_CE4100_USB)
|
|
hcd->has_tt = 1;
|
|
break;
|
|
case PCI_VENDOR_ID_TDI:
|
|
if (pdev->device == PCI_DEVICE_ID_TDI_EHCI)
|
|
hcd->has_tt = 1;
|
|
break;
|
|
case PCI_VENDOR_ID_AMD:
|
|
/* AMD PLL quirk */
|
|
if (usb_amd_find_chipset_info())
|
|
ehci->amd_pll_fix = 1;
|
|
/* AMD8111 EHCI doesn't work, according to AMD errata */
|
|
if (pdev->device == 0x7463) {
|
|
ehci_info(ehci, "ignoring AMD8111 (errata)\n");
|
|
retval = -EIO;
|
|
goto done;
|
|
}
|
|
|
|
/*
|
|
* EHCI controller on AMD SB700/SB800/Hudson-2/3 platforms may
|
|
* read/write memory space which does not belong to it when
|
|
* there is NULL pointer with T-bit set to 1 in the frame list
|
|
* table. To avoid the issue, the frame list link pointer
|
|
* should always contain a valid pointer to a inactive qh.
|
|
*/
|
|
if (pdev->device == 0x7808) {
|
|
ehci->use_dummy_qh = 1;
|
|
ehci_info(ehci, "applying AMD SB700/SB800/Hudson-2/3 EHCI dummy qh workaround\n");
|
|
}
|
|
break;
|
|
case PCI_VENDOR_ID_VIA:
|
|
if (pdev->device == 0x3104 && (pdev->revision & 0xf0) == 0x60) {
|
|
u8 tmp;
|
|
|
|
/* The VT6212 defaults to a 1 usec EHCI sleep time which
|
|
* hogs the PCI bus *badly*. Setting bit 5 of 0x4B makes
|
|
* that sleep time use the conventional 10 usec.
|
|
*/
|
|
pci_read_config_byte(pdev, 0x4b, &tmp);
|
|
if (tmp & 0x20)
|
|
break;
|
|
pci_write_config_byte(pdev, 0x4b, tmp | 0x20);
|
|
}
|
|
break;
|
|
case PCI_VENDOR_ID_ATI:
|
|
/* AMD PLL quirk */
|
|
if (usb_amd_find_chipset_info())
|
|
ehci->amd_pll_fix = 1;
|
|
|
|
/*
|
|
* EHCI controller on AMD SB700/SB800/Hudson-2/3 platforms may
|
|
* read/write memory space which does not belong to it when
|
|
* there is NULL pointer with T-bit set to 1 in the frame list
|
|
* table. To avoid the issue, the frame list link pointer
|
|
* should always contain a valid pointer to a inactive qh.
|
|
*/
|
|
if (pdev->device == 0x4396) {
|
|
ehci->use_dummy_qh = 1;
|
|
ehci_info(ehci, "applying AMD SB700/SB800/Hudson-2/3 EHCI dummy qh workaround\n");
|
|
}
|
|
/* SB600 and old version of SB700 have a bug in EHCI controller,
|
|
* which causes usb devices lose response in some cases.
|
|
*/
|
|
if ((pdev->device == 0x4386) || (pdev->device == 0x4396)) {
|
|
p_smbus = pci_get_device(PCI_VENDOR_ID_ATI,
|
|
PCI_DEVICE_ID_ATI_SBX00_SMBUS,
|
|
NULL);
|
|
if (!p_smbus)
|
|
break;
|
|
rev = p_smbus->revision;
|
|
if ((pdev->device == 0x4386) || (rev == 0x3a)
|
|
|| (rev == 0x3b)) {
|
|
u8 tmp;
|
|
ehci_info(ehci, "applying AMD SB600/SB700 USB "
|
|
"freeze workaround\n");
|
|
pci_read_config_byte(pdev, 0x53, &tmp);
|
|
pci_write_config_byte(pdev, 0x53, tmp | (1<<3));
|
|
}
|
|
pci_dev_put(p_smbus);
|
|
}
|
|
break;
|
|
case PCI_VENDOR_ID_NETMOS:
|
|
/* MosChip frame-index-register bug */
|
|
ehci_info(ehci, "applying MosChip frame-index workaround\n");
|
|
ehci->frame_index_bug = 1;
|
|
break;
|
|
}
|
|
|
|
retval = ehci_setup(hcd);
|
|
if (retval)
|
|
return retval;
|
|
|
|
/* These workarounds need to be applied after ehci_setup() */
|
|
switch (pdev->vendor) {
|
|
case PCI_VENDOR_ID_NEC:
|
|
ehci->need_io_watchdog = 0;
|
|
break;
|
|
case PCI_VENDOR_ID_INTEL:
|
|
ehci->need_io_watchdog = 0;
|
|
if (pdev->device == 0x0806 || pdev->device == 0x0811
|
|
|| pdev->device == 0x0829) {
|
|
ehci_info(ehci, "disable lpm for langwell/penwell\n");
|
|
ehci->has_lpm = 0;
|
|
}
|
|
break;
|
|
case PCI_VENDOR_ID_NVIDIA:
|
|
switch (pdev->device) {
|
|
/* MCP89 chips on the MacBookAir3,1 give EPROTO when
|
|
* fetching device descriptors unless LPM is disabled.
|
|
* There are also intermittent problems enumerating
|
|
* devices with PPCD enabled.
|
|
*/
|
|
case 0x0d9d:
|
|
ehci_info(ehci, "disable lpm/ppcd for nvidia mcp89");
|
|
ehci->has_lpm = 0;
|
|
ehci->has_ppcd = 0;
|
|
ehci->command &= ~CMD_PPCEE;
|
|
break;
|
|
}
|
|
break;
|
|
}
|
|
|
|
/* optional debug port, normally in the first BAR */
|
|
temp = pci_find_capability(pdev, 0x0a);
|
|
if (temp) {
|
|
pci_read_config_dword(pdev, temp, &temp);
|
|
temp >>= 16;
|
|
if ((temp & (3 << 13)) == (1 << 13)) {
|
|
temp &= 0x1fff;
|
|
ehci->debug = hcd->regs + temp;
|
|
temp = ehci_readl(ehci, &ehci->debug->control);
|
|
ehci_info(ehci, "debug port %d%s\n",
|
|
HCS_DEBUG_PORT(ehci->hcs_params),
|
|
(temp & DBGP_ENABLED)
|
|
? " IN USE"
|
|
: "");
|
|
if (!(temp & DBGP_ENABLED))
|
|
ehci->debug = NULL;
|
|
}
|
|
}
|
|
|
|
/* at least the Genesys GL880S needs fixup here */
|
|
temp = HCS_N_CC(ehci->hcs_params) * HCS_N_PCC(ehci->hcs_params);
|
|
temp &= 0x0f;
|
|
if (temp && HCS_N_PORTS(ehci->hcs_params) > temp) {
|
|
ehci_dbg(ehci, "bogus port configuration: "
|
|
"cc=%d x pcc=%d < ports=%d\n",
|
|
HCS_N_CC(ehci->hcs_params),
|
|
HCS_N_PCC(ehci->hcs_params),
|
|
HCS_N_PORTS(ehci->hcs_params));
|
|
|
|
switch (pdev->vendor) {
|
|
case 0x17a0: /* GENESYS */
|
|
/* GL880S: should be PORTS=2 */
|
|
temp |= (ehci->hcs_params & ~0xf);
|
|
ehci->hcs_params = temp;
|
|
break;
|
|
case PCI_VENDOR_ID_NVIDIA:
|
|
/* NF4: should be PCC=10 */
|
|
break;
|
|
}
|
|
}
|
|
|
|
/* Serial Bus Release Number is at PCI 0x60 offset */
|
|
if (pdev->vendor == PCI_VENDOR_ID_STMICRO
|
|
&& pdev->device == PCI_DEVICE_ID_STMICRO_USB_HOST)
|
|
; /* ConneXT has no sbrn register */
|
|
else
|
|
pci_read_config_byte(pdev, 0x60, &ehci->sbrn);
|
|
|
|
/* Keep this around for a while just in case some EHCI
|
|
* implementation uses legacy PCI PM support. This test
|
|
* can be removed on 17 Dec 2009 if the dev_warn() hasn't
|
|
* been triggered by then.
|
|
*/
|
|
if (!device_can_wakeup(&pdev->dev)) {
|
|
u16 port_wake;
|
|
|
|
pci_read_config_word(pdev, 0x62, &port_wake);
|
|
if (port_wake & 0x0001) {
|
|
dev_warn(&pdev->dev, "Enabling legacy PCI PM\n");
|
|
device_set_wakeup_capable(&pdev->dev, 1);
|
|
}
|
|
}
|
|
|
|
#ifdef CONFIG_USB_SUSPEND
|
|
/* REVISIT: the controller works fine for wakeup iff the root hub
|
|
* itself is "globally" suspended, but usbcore currently doesn't
|
|
* understand such things.
|
|
*
|
|
* System suspend currently expects to be able to suspend the entire
|
|
* device tree, device-at-a-time. If we failed selective suspend
|
|
* reports, system suspend would fail; so the root hub code must claim
|
|
* success. That's lying to usbcore, and it matters for runtime
|
|
* PM scenarios with selective suspend and remote wakeup...
|
|
*/
|
|
if (ehci->no_selective_suspend && device_can_wakeup(&pdev->dev))
|
|
ehci_warn(ehci, "selective suspend/wakeup unavailable\n");
|
|
#endif
|
|
|
|
ehci_port_power(ehci, 1);
|
|
retval = ehci_pci_reinit(ehci, pdev);
|
|
done:
|
|
return retval;
|
|
}
|
|
|
|
/*-------------------------------------------------------------------------*/
|
|
|
|
#ifdef CONFIG_PM
|
|
|
|
/* suspend/resume, section 4.3 */
|
|
|
|
/* These routines rely on the PCI bus glue
|
|
* to handle powerdown and wakeup, and currently also on
|
|
* transceivers that don't need any software attention to set up
|
|
* the right sort of wakeup.
|
|
* Also they depend on separate root hub suspend/resume.
|
|
*/
|
|
|
|
static int ehci_pci_suspend(struct usb_hcd *hcd, bool do_wakeup)
|
|
{
|
|
return ehci_suspend(hcd, do_wakeup);
|
|
}
|
|
|
|
static bool usb_is_intel_switchable_ehci(struct pci_dev *pdev)
|
|
{
|
|
return pdev->class == PCI_CLASS_SERIAL_USB_EHCI &&
|
|
pdev->vendor == PCI_VENDOR_ID_INTEL &&
|
|
(pdev->device == 0x1E26 ||
|
|
pdev->device == 0x8C2D ||
|
|
pdev->device == 0x8C26);
|
|
}
|
|
|
|
static void ehci_enable_xhci_companion(void)
|
|
{
|
|
struct pci_dev *companion = NULL;
|
|
|
|
/* The xHCI and EHCI controllers are not on the same PCI slot */
|
|
for_each_pci_dev(companion) {
|
|
if (!usb_is_intel_switchable_xhci(companion))
|
|
continue;
|
|
usb_enable_xhci_ports(companion);
|
|
return;
|
|
}
|
|
}
|
|
|
|
static int ehci_pci_resume(struct usb_hcd *hcd, bool hibernated)
|
|
{
|
|
struct ehci_hcd *ehci = hcd_to_ehci(hcd);
|
|
struct pci_dev *pdev = to_pci_dev(hcd->self.controller);
|
|
|
|
/* The BIOS on systems with the Intel Panther Point chipset may or may
|
|
* not support xHCI natively. That means that during system resume, it
|
|
* may switch the ports back to EHCI so that users can use their
|
|
* keyboard to select a kernel from GRUB after resume from hibernate.
|
|
*
|
|
* The BIOS is supposed to remember whether the OS had xHCI ports
|
|
* enabled before resume, and switch the ports back to xHCI when the
|
|
* BIOS/OS semaphore is written, but we all know we can't trust BIOS
|
|
* writers.
|
|
*
|
|
* Unconditionally switch the ports back to xHCI after a system resume.
|
|
* We can't tell whether the EHCI or xHCI controller will be resumed
|
|
* first, so we have to do the port switchover in both drivers. Writing
|
|
* a '1' to the port switchover registers should have no effect if the
|
|
* port was already switched over.
|
|
*/
|
|
if (usb_is_intel_switchable_ehci(pdev))
|
|
ehci_enable_xhci_companion();
|
|
|
|
if (ehci_resume(hcd, hibernated) != 0)
|
|
(void) ehci_pci_reinit(ehci, pdev);
|
|
return 0;
|
|
}
|
|
#endif
|
|
|
|
static int ehci_update_device(struct usb_hcd *hcd, struct usb_device *udev)
|
|
{
|
|
struct ehci_hcd *ehci = hcd_to_ehci(hcd);
|
|
int rc = 0;
|
|
|
|
if (!udev->parent) /* udev is root hub itself, impossible */
|
|
rc = -1;
|
|
/* we only support lpm device connected to root hub yet */
|
|
if (ehci->has_lpm && !udev->parent->parent) {
|
|
rc = ehci_lpm_set_da(ehci, udev->devnum, udev->portnum);
|
|
if (!rc)
|
|
rc = ehci_lpm_check(ehci, udev->portnum);
|
|
}
|
|
return rc;
|
|
}
|
|
|
|
static const struct hc_driver ehci_pci_hc_driver = {
|
|
.description = hcd_name,
|
|
.product_desc = "EHCI Host Controller",
|
|
.hcd_priv_size = sizeof(struct ehci_hcd),
|
|
|
|
/*
|
|
* generic hardware linkage
|
|
*/
|
|
.irq = ehci_irq,
|
|
.flags = HCD_MEMORY | HCD_USB2,
|
|
|
|
/*
|
|
* basic lifecycle operations
|
|
*/
|
|
.reset = ehci_pci_setup,
|
|
.start = ehci_run,
|
|
#ifdef CONFIG_PM
|
|
.pci_suspend = ehci_pci_suspend,
|
|
.pci_resume = ehci_pci_resume,
|
|
#endif
|
|
.stop = ehci_stop,
|
|
.shutdown = ehci_shutdown,
|
|
|
|
/*
|
|
* managing i/o requests and associated device resources
|
|
*/
|
|
.urb_enqueue = ehci_urb_enqueue,
|
|
.urb_dequeue = ehci_urb_dequeue,
|
|
.endpoint_disable = ehci_endpoint_disable,
|
|
.endpoint_reset = ehci_endpoint_reset,
|
|
|
|
/*
|
|
* scheduling support
|
|
*/
|
|
.get_frame_number = ehci_get_frame,
|
|
|
|
/*
|
|
* root hub support
|
|
*/
|
|
.hub_status_data = ehci_hub_status_data,
|
|
.hub_control = ehci_hub_control,
|
|
.bus_suspend = ehci_bus_suspend,
|
|
.bus_resume = ehci_bus_resume,
|
|
.relinquish_port = ehci_relinquish_port,
|
|
.port_handed_over = ehci_port_handed_over,
|
|
|
|
/*
|
|
* call back when device connected and addressed
|
|
*/
|
|
.update_device = ehci_update_device,
|
|
|
|
.clear_tt_buffer_complete = ehci_clear_tt_buffer_complete,
|
|
};
|
|
|
|
/*-------------------------------------------------------------------------*/
|
|
|
|
/* PCI driver selection metadata; PCI hotplugging uses this */
|
|
static const struct pci_device_id pci_ids [] = { {
|
|
/* handle any USB 2.0 EHCI controller */
|
|
PCI_DEVICE_CLASS(PCI_CLASS_SERIAL_USB_EHCI, ~0),
|
|
.driver_data = (unsigned long) &ehci_pci_hc_driver,
|
|
}, {
|
|
PCI_VDEVICE(STMICRO, PCI_DEVICE_ID_STMICRO_USB_HOST),
|
|
.driver_data = (unsigned long) &ehci_pci_hc_driver,
|
|
},
|
|
{ /* end: all zeroes */ }
|
|
};
|
|
MODULE_DEVICE_TABLE(pci, pci_ids);
|
|
|
|
/* pci driver glue; this is a "new style" PCI driver module */
|
|
static struct pci_driver ehci_pci_driver = {
|
|
.name = (char *) hcd_name,
|
|
.id_table = pci_ids,
|
|
|
|
.probe = usb_hcd_pci_probe,
|
|
.remove = usb_hcd_pci_remove,
|
|
.shutdown = usb_hcd_pci_shutdown,
|
|
|
|
#ifdef CONFIG_PM_SLEEP
|
|
.driver = {
|
|
.pm = &usb_hcd_pci_pm_ops
|
|
},
|
|
#endif
|
|
};
|