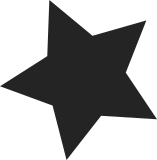
With radix, we can get page fault with DSISR_PROTFAULT value set in case of PROT_NONE or autonuma mapping. The PROT_NONE case in handled by the vma check where we consider the access bad. For autonuma we should fall through and fixup the access mask correctly. Without this patch we trigger the WARN_ON() on radix. This code moves that WARN_ON() within a radix_enabled() check. I also moved the WARN_ON() outside the if condition making it apply for all type of faults (exec/write/read). It is also conditionalized for book3s, because BOOK3E can also get a PROTFAULT to handle the D/I cache sync. Signed-off-by: Aneesh Kumar K.V <aneesh.kumar@linux.vnet.ibm.com> Signed-off-by: Michael Ellerman <mpe@ellerman.id.au>
163 lines
4 KiB
C
163 lines
4 KiB
C
/*
|
|
* CoProcessor (SPU/AFU) mm fault handler
|
|
*
|
|
* (C) Copyright IBM Deutschland Entwicklung GmbH 2007
|
|
*
|
|
* Author: Arnd Bergmann <arndb@de.ibm.com>
|
|
* Author: Jeremy Kerr <jk@ozlabs.org>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2, or (at your option)
|
|
* any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
|
|
*/
|
|
#include <linux/sched.h>
|
|
#include <linux/mm.h>
|
|
#include <linux/export.h>
|
|
#include <asm/reg.h>
|
|
#include <asm/copro.h>
|
|
#include <asm/spu.h>
|
|
#include <misc/cxl-base.h>
|
|
|
|
/*
|
|
* This ought to be kept in sync with the powerpc specific do_page_fault
|
|
* function. Currently, there are a few corner cases that we haven't had
|
|
* to handle fortunately.
|
|
*/
|
|
int copro_handle_mm_fault(struct mm_struct *mm, unsigned long ea,
|
|
unsigned long dsisr, unsigned *flt)
|
|
{
|
|
struct vm_area_struct *vma;
|
|
unsigned long is_write;
|
|
int ret;
|
|
|
|
if (mm == NULL)
|
|
return -EFAULT;
|
|
|
|
if (mm->pgd == NULL)
|
|
return -EFAULT;
|
|
|
|
down_read(&mm->mmap_sem);
|
|
ret = -EFAULT;
|
|
vma = find_vma(mm, ea);
|
|
if (!vma)
|
|
goto out_unlock;
|
|
|
|
if (ea < vma->vm_start) {
|
|
if (!(vma->vm_flags & VM_GROWSDOWN))
|
|
goto out_unlock;
|
|
if (expand_stack(vma, ea))
|
|
goto out_unlock;
|
|
}
|
|
|
|
is_write = dsisr & DSISR_ISSTORE;
|
|
if (is_write) {
|
|
if (!(vma->vm_flags & VM_WRITE))
|
|
goto out_unlock;
|
|
} else {
|
|
if (!(vma->vm_flags & (VM_READ | VM_EXEC)))
|
|
goto out_unlock;
|
|
/*
|
|
* PROT_NONE is covered by the VMA check above.
|
|
* and hash should get a NOHPTE fault instead of
|
|
* a PROTFAULT in case fixup is needed for things
|
|
* like autonuma.
|
|
*/
|
|
if (!radix_enabled())
|
|
WARN_ON_ONCE(dsisr & DSISR_PROTFAULT);
|
|
}
|
|
|
|
ret = 0;
|
|
*flt = handle_mm_fault(vma, ea, is_write ? FAULT_FLAG_WRITE : 0);
|
|
if (unlikely(*flt & VM_FAULT_ERROR)) {
|
|
if (*flt & VM_FAULT_OOM) {
|
|
ret = -ENOMEM;
|
|
goto out_unlock;
|
|
} else if (*flt & (VM_FAULT_SIGBUS | VM_FAULT_SIGSEGV)) {
|
|
ret = -EFAULT;
|
|
goto out_unlock;
|
|
}
|
|
BUG();
|
|
}
|
|
|
|
if (*flt & VM_FAULT_MAJOR)
|
|
current->maj_flt++;
|
|
else
|
|
current->min_flt++;
|
|
|
|
out_unlock:
|
|
up_read(&mm->mmap_sem);
|
|
return ret;
|
|
}
|
|
EXPORT_SYMBOL_GPL(copro_handle_mm_fault);
|
|
|
|
int copro_calculate_slb(struct mm_struct *mm, u64 ea, struct copro_slb *slb)
|
|
{
|
|
u64 vsid, vsidkey;
|
|
int psize, ssize;
|
|
|
|
switch (REGION_ID(ea)) {
|
|
case USER_REGION_ID:
|
|
pr_devel("%s: 0x%llx -- USER_REGION_ID\n", __func__, ea);
|
|
if (mm == NULL)
|
|
return 1;
|
|
psize = get_slice_psize(mm, ea);
|
|
ssize = user_segment_size(ea);
|
|
vsid = get_vsid(mm->context.id, ea, ssize);
|
|
vsidkey = SLB_VSID_USER;
|
|
break;
|
|
case VMALLOC_REGION_ID:
|
|
pr_devel("%s: 0x%llx -- VMALLOC_REGION_ID\n", __func__, ea);
|
|
if (ea < VMALLOC_END)
|
|
psize = mmu_vmalloc_psize;
|
|
else
|
|
psize = mmu_io_psize;
|
|
ssize = mmu_kernel_ssize;
|
|
vsid = get_kernel_vsid(ea, mmu_kernel_ssize);
|
|
vsidkey = SLB_VSID_KERNEL;
|
|
break;
|
|
case KERNEL_REGION_ID:
|
|
pr_devel("%s: 0x%llx -- KERNEL_REGION_ID\n", __func__, ea);
|
|
psize = mmu_linear_psize;
|
|
ssize = mmu_kernel_ssize;
|
|
vsid = get_kernel_vsid(ea, mmu_kernel_ssize);
|
|
vsidkey = SLB_VSID_KERNEL;
|
|
break;
|
|
default:
|
|
pr_debug("%s: invalid region access at %016llx\n", __func__, ea);
|
|
return 1;
|
|
}
|
|
/* Bad address */
|
|
if (!vsid)
|
|
return 1;
|
|
|
|
vsid = (vsid << slb_vsid_shift(ssize)) | vsidkey;
|
|
|
|
vsid |= mmu_psize_defs[psize].sllp |
|
|
((ssize == MMU_SEGSIZE_1T) ? SLB_VSID_B_1T : 0);
|
|
|
|
slb->esid = (ea & (ssize == MMU_SEGSIZE_1T ? ESID_MASK_1T : ESID_MASK)) | SLB_ESID_V;
|
|
slb->vsid = vsid;
|
|
|
|
return 0;
|
|
}
|
|
EXPORT_SYMBOL_GPL(copro_calculate_slb);
|
|
|
|
void copro_flush_all_slbs(struct mm_struct *mm)
|
|
{
|
|
#ifdef CONFIG_SPU_BASE
|
|
spu_flush_all_slbs(mm);
|
|
#endif
|
|
cxl_slbia(mm);
|
|
}
|
|
EXPORT_SYMBOL_GPL(copro_flush_all_slbs);
|