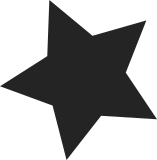
The replacement of <asm/pgrable.h> with <linux/pgtable.h> made the include of the latter in the middle of asm includes. Fix this up with the aid of the below script and manual adjustments here and there. import sys import re if len(sys.argv) is not 3: print "USAGE: %s <file> <header>" % (sys.argv[0]) sys.exit(1) hdr_to_move="#include <linux/%s>" % sys.argv[2] moved = False in_hdrs = False with open(sys.argv[1], "r") as f: lines = f.readlines() for _line in lines: line = _line.rstrip(' ') if line == hdr_to_move: continue if line.startswith("#include <linux/"): in_hdrs = True elif not moved and in_hdrs: moved = True print hdr_to_move print line Signed-off-by: Mike Rapoport <rppt@linux.ibm.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Cc: Arnd Bergmann <arnd@arndb.de> Cc: Borislav Petkov <bp@alien8.de> Cc: Brian Cain <bcain@codeaurora.org> Cc: Catalin Marinas <catalin.marinas@arm.com> Cc: Chris Zankel <chris@zankel.net> Cc: "David S. Miller" <davem@davemloft.net> Cc: Geert Uytterhoeven <geert@linux-m68k.org> Cc: Greentime Hu <green.hu@gmail.com> Cc: Greg Ungerer <gerg@linux-m68k.org> Cc: Guan Xuetao <gxt@pku.edu.cn> Cc: Guo Ren <guoren@kernel.org> Cc: Heiko Carstens <heiko.carstens@de.ibm.com> Cc: Helge Deller <deller@gmx.de> Cc: Ingo Molnar <mingo@redhat.com> Cc: Ley Foon Tan <ley.foon.tan@intel.com> Cc: Mark Salter <msalter@redhat.com> Cc: Matthew Wilcox <willy@infradead.org> Cc: Matt Turner <mattst88@gmail.com> Cc: Max Filippov <jcmvbkbc@gmail.com> Cc: Michael Ellerman <mpe@ellerman.id.au> Cc: Michal Simek <monstr@monstr.eu> Cc: Nick Hu <nickhu@andestech.com> Cc: Paul Walmsley <paul.walmsley@sifive.com> Cc: Richard Weinberger <richard@nod.at> Cc: Rich Felker <dalias@libc.org> Cc: Russell King <linux@armlinux.org.uk> Cc: Stafford Horne <shorne@gmail.com> Cc: Thomas Bogendoerfer <tsbogend@alpha.franken.de> Cc: Thomas Gleixner <tglx@linutronix.de> Cc: Tony Luck <tony.luck@intel.com> Cc: Vincent Chen <deanbo422@gmail.com> Cc: Vineet Gupta <vgupta@synopsys.com> Cc: Will Deacon <will@kernel.org> Cc: Yoshinori Sato <ysato@users.sourceforge.jp> Link: http://lkml.kernel.org/r/20200514170327.31389-4-rppt@kernel.org Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
190 lines
3.7 KiB
C
190 lines
3.7 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/*
|
|
* Copyright © 2008 Keith Packard <keithp@keithp.com>
|
|
*/
|
|
|
|
#ifndef _LINUX_IO_MAPPING_H
|
|
#define _LINUX_IO_MAPPING_H
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/bug.h>
|
|
#include <linux/io.h>
|
|
#include <linux/pgtable.h>
|
|
#include <asm/page.h>
|
|
|
|
/*
|
|
* The io_mapping mechanism provides an abstraction for mapping
|
|
* individual pages from an io device to the CPU in an efficient fashion.
|
|
*
|
|
* See Documentation/driver-api/io-mapping.rst
|
|
*/
|
|
|
|
struct io_mapping {
|
|
resource_size_t base;
|
|
unsigned long size;
|
|
pgprot_t prot;
|
|
void __iomem *iomem;
|
|
};
|
|
|
|
#ifdef CONFIG_HAVE_ATOMIC_IOMAP
|
|
|
|
#include <linux/pfn.h>
|
|
#include <asm/iomap.h>
|
|
/*
|
|
* For small address space machines, mapping large objects
|
|
* into the kernel virtual space isn't practical. Where
|
|
* available, use fixmap support to dynamically map pages
|
|
* of the object at run time.
|
|
*/
|
|
|
|
static inline struct io_mapping *
|
|
io_mapping_init_wc(struct io_mapping *iomap,
|
|
resource_size_t base,
|
|
unsigned long size)
|
|
{
|
|
pgprot_t prot;
|
|
|
|
if (iomap_create_wc(base, size, &prot))
|
|
return NULL;
|
|
|
|
iomap->base = base;
|
|
iomap->size = size;
|
|
iomap->prot = prot;
|
|
return iomap;
|
|
}
|
|
|
|
static inline void
|
|
io_mapping_fini(struct io_mapping *mapping)
|
|
{
|
|
iomap_free(mapping->base, mapping->size);
|
|
}
|
|
|
|
/* Atomic map/unmap */
|
|
static inline void __iomem *
|
|
io_mapping_map_atomic_wc(struct io_mapping *mapping,
|
|
unsigned long offset)
|
|
{
|
|
resource_size_t phys_addr;
|
|
|
|
BUG_ON(offset >= mapping->size);
|
|
phys_addr = mapping->base + offset;
|
|
return iomap_atomic_prot_pfn(PHYS_PFN(phys_addr), mapping->prot);
|
|
}
|
|
|
|
static inline void
|
|
io_mapping_unmap_atomic(void __iomem *vaddr)
|
|
{
|
|
iounmap_atomic(vaddr);
|
|
}
|
|
|
|
static inline void __iomem *
|
|
io_mapping_map_wc(struct io_mapping *mapping,
|
|
unsigned long offset,
|
|
unsigned long size)
|
|
{
|
|
resource_size_t phys_addr;
|
|
|
|
BUG_ON(offset >= mapping->size);
|
|
phys_addr = mapping->base + offset;
|
|
|
|
return ioremap_wc(phys_addr, size);
|
|
}
|
|
|
|
static inline void
|
|
io_mapping_unmap(void __iomem *vaddr)
|
|
{
|
|
iounmap(vaddr);
|
|
}
|
|
|
|
#else
|
|
|
|
#include <linux/uaccess.h>
|
|
|
|
/* Create the io_mapping object*/
|
|
static inline struct io_mapping *
|
|
io_mapping_init_wc(struct io_mapping *iomap,
|
|
resource_size_t base,
|
|
unsigned long size)
|
|
{
|
|
iomap->base = base;
|
|
iomap->size = size;
|
|
iomap->iomem = ioremap_wc(base, size);
|
|
#if defined(pgprot_noncached_wc) /* archs can't agree on a name ... */
|
|
iomap->prot = pgprot_noncached_wc(PAGE_KERNEL);
|
|
#elif defined(pgprot_writecombine)
|
|
iomap->prot = pgprot_writecombine(PAGE_KERNEL);
|
|
#else
|
|
iomap->prot = pgprot_noncached(PAGE_KERNEL);
|
|
#endif
|
|
|
|
return iomap;
|
|
}
|
|
|
|
static inline void
|
|
io_mapping_fini(struct io_mapping *mapping)
|
|
{
|
|
iounmap(mapping->iomem);
|
|
}
|
|
|
|
/* Non-atomic map/unmap */
|
|
static inline void __iomem *
|
|
io_mapping_map_wc(struct io_mapping *mapping,
|
|
unsigned long offset,
|
|
unsigned long size)
|
|
{
|
|
return mapping->iomem + offset;
|
|
}
|
|
|
|
static inline void
|
|
io_mapping_unmap(void __iomem *vaddr)
|
|
{
|
|
}
|
|
|
|
/* Atomic map/unmap */
|
|
static inline void __iomem *
|
|
io_mapping_map_atomic_wc(struct io_mapping *mapping,
|
|
unsigned long offset)
|
|
{
|
|
preempt_disable();
|
|
pagefault_disable();
|
|
return io_mapping_map_wc(mapping, offset, PAGE_SIZE);
|
|
}
|
|
|
|
static inline void
|
|
io_mapping_unmap_atomic(void __iomem *vaddr)
|
|
{
|
|
io_mapping_unmap(vaddr);
|
|
pagefault_enable();
|
|
preempt_enable();
|
|
}
|
|
|
|
#endif /* HAVE_ATOMIC_IOMAP */
|
|
|
|
static inline struct io_mapping *
|
|
io_mapping_create_wc(resource_size_t base,
|
|
unsigned long size)
|
|
{
|
|
struct io_mapping *iomap;
|
|
|
|
iomap = kmalloc(sizeof(*iomap), GFP_KERNEL);
|
|
if (!iomap)
|
|
return NULL;
|
|
|
|
if (!io_mapping_init_wc(iomap, base, size)) {
|
|
kfree(iomap);
|
|
return NULL;
|
|
}
|
|
|
|
return iomap;
|
|
}
|
|
|
|
static inline void
|
|
io_mapping_free(struct io_mapping *iomap)
|
|
{
|
|
io_mapping_fini(iomap);
|
|
kfree(iomap);
|
|
}
|
|
|
|
#endif /* _LINUX_IO_MAPPING_H */
|