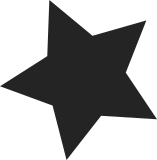
This avoids use of the kernel-internal "xtime" variable directly outside of the actual time-related functions. Instead, use the helper functions that we already have available to us. This doesn't actually change any behaviour, but this will allow us to fix the fact that "xtime" isn't updated very often with CONFIG_NO_HZ (because much of the realtime information is maintained as separate offsets to 'xtime'), which has caused interfaces that use xtime directly to get a time that is out of sync with the real-time clock by up to a third of a second or so. Signed-off-by: John Stultz <johnstul@us.ibm.com> Cc: Ingo Molnar <mingo@elte.hu> Cc: Thomas Gleixner <tglx@linutronix.de> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
240 lines
5.1 KiB
C
240 lines
5.1 KiB
C
/*
|
|
* RTC subsystem, base class
|
|
*
|
|
* Copyright (C) 2005 Tower Technologies
|
|
* Author: Alessandro Zummo <a.zummo@towertech.it>
|
|
*
|
|
* class skeleton from drivers/hwmon/hwmon.c
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*/
|
|
|
|
#include <linux/module.h>
|
|
#include <linux/rtc.h>
|
|
#include <linux/kdev_t.h>
|
|
#include <linux/idr.h>
|
|
|
|
#include "rtc-core.h"
|
|
|
|
|
|
static DEFINE_IDR(rtc_idr);
|
|
static DEFINE_MUTEX(idr_lock);
|
|
struct class *rtc_class;
|
|
|
|
static void rtc_device_release(struct device *dev)
|
|
{
|
|
struct rtc_device *rtc = to_rtc_device(dev);
|
|
mutex_lock(&idr_lock);
|
|
idr_remove(&rtc_idr, rtc->id);
|
|
mutex_unlock(&idr_lock);
|
|
kfree(rtc);
|
|
}
|
|
|
|
#if defined(CONFIG_PM) && defined(CONFIG_RTC_HCTOSYS_DEVICE)
|
|
|
|
/*
|
|
* On suspend(), measure the delta between one RTC and the
|
|
* system's wall clock; restore it on resume().
|
|
*/
|
|
|
|
static struct timespec delta;
|
|
static time_t oldtime;
|
|
|
|
static int rtc_suspend(struct device *dev, pm_message_t mesg)
|
|
{
|
|
struct rtc_device *rtc = to_rtc_device(dev);
|
|
struct rtc_time tm;
|
|
struct timespec ts = current_kernel_time();
|
|
|
|
if (strncmp(rtc->dev.bus_id,
|
|
CONFIG_RTC_HCTOSYS_DEVICE,
|
|
BUS_ID_SIZE) != 0)
|
|
return 0;
|
|
|
|
rtc_read_time(rtc, &tm);
|
|
rtc_tm_to_time(&tm, &oldtime);
|
|
|
|
/* RTC precision is 1 second; adjust delta for avg 1/2 sec err */
|
|
set_normalized_timespec(&delta,
|
|
ts.tv_sec - oldtime,
|
|
ts.tv_nsec - (NSEC_PER_SEC >> 1));
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int rtc_resume(struct device *dev)
|
|
{
|
|
struct rtc_device *rtc = to_rtc_device(dev);
|
|
struct rtc_time tm;
|
|
time_t newtime;
|
|
struct timespec time;
|
|
|
|
if (strncmp(rtc->dev.bus_id,
|
|
CONFIG_RTC_HCTOSYS_DEVICE,
|
|
BUS_ID_SIZE) != 0)
|
|
return 0;
|
|
|
|
rtc_read_time(rtc, &tm);
|
|
if (rtc_valid_tm(&tm) != 0) {
|
|
pr_debug("%s: bogus resume time\n", rtc->dev.bus_id);
|
|
return 0;
|
|
}
|
|
rtc_tm_to_time(&tm, &newtime);
|
|
if (newtime <= oldtime) {
|
|
if (newtime < oldtime)
|
|
pr_debug("%s: time travel!\n", rtc->dev.bus_id);
|
|
return 0;
|
|
}
|
|
|
|
/* restore wall clock using delta against this RTC;
|
|
* adjust again for avg 1/2 second RTC sampling error
|
|
*/
|
|
set_normalized_timespec(&time,
|
|
newtime + delta.tv_sec,
|
|
(NSEC_PER_SEC >> 1) + delta.tv_nsec);
|
|
do_settimeofday(&time);
|
|
|
|
return 0;
|
|
}
|
|
|
|
#else
|
|
#define rtc_suspend NULL
|
|
#define rtc_resume NULL
|
|
#endif
|
|
|
|
|
|
/**
|
|
* rtc_device_register - register w/ RTC class
|
|
* @dev: the device to register
|
|
*
|
|
* rtc_device_unregister() must be called when the class device is no
|
|
* longer needed.
|
|
*
|
|
* Returns the pointer to the new struct class device.
|
|
*/
|
|
struct rtc_device *rtc_device_register(const char *name, struct device *dev,
|
|
const struct rtc_class_ops *ops,
|
|
struct module *owner)
|
|
{
|
|
struct rtc_device *rtc;
|
|
int id, err;
|
|
|
|
if (idr_pre_get(&rtc_idr, GFP_KERNEL) == 0) {
|
|
err = -ENOMEM;
|
|
goto exit;
|
|
}
|
|
|
|
|
|
mutex_lock(&idr_lock);
|
|
err = idr_get_new(&rtc_idr, NULL, &id);
|
|
mutex_unlock(&idr_lock);
|
|
|
|
if (err < 0)
|
|
goto exit;
|
|
|
|
id = id & MAX_ID_MASK;
|
|
|
|
rtc = kzalloc(sizeof(struct rtc_device), GFP_KERNEL);
|
|
if (rtc == NULL) {
|
|
err = -ENOMEM;
|
|
goto exit_idr;
|
|
}
|
|
|
|
rtc->id = id;
|
|
rtc->ops = ops;
|
|
rtc->owner = owner;
|
|
rtc->max_user_freq = 64;
|
|
rtc->dev.parent = dev;
|
|
rtc->dev.class = rtc_class;
|
|
rtc->dev.release = rtc_device_release;
|
|
|
|
mutex_init(&rtc->ops_lock);
|
|
spin_lock_init(&rtc->irq_lock);
|
|
spin_lock_init(&rtc->irq_task_lock);
|
|
|
|
strlcpy(rtc->name, name, RTC_DEVICE_NAME_SIZE);
|
|
snprintf(rtc->dev.bus_id, BUS_ID_SIZE, "rtc%d", id);
|
|
|
|
rtc_dev_prepare(rtc);
|
|
|
|
err = device_register(&rtc->dev);
|
|
if (err)
|
|
goto exit_kfree;
|
|
|
|
rtc_dev_add_device(rtc);
|
|
rtc_sysfs_add_device(rtc);
|
|
rtc_proc_add_device(rtc);
|
|
|
|
dev_info(dev, "rtc core: registered %s as %s\n",
|
|
rtc->name, rtc->dev.bus_id);
|
|
|
|
return rtc;
|
|
|
|
exit_kfree:
|
|
kfree(rtc);
|
|
|
|
exit_idr:
|
|
mutex_lock(&idr_lock);
|
|
idr_remove(&rtc_idr, id);
|
|
mutex_unlock(&idr_lock);
|
|
|
|
exit:
|
|
dev_err(dev, "rtc core: unable to register %s, err = %d\n",
|
|
name, err);
|
|
return ERR_PTR(err);
|
|
}
|
|
EXPORT_SYMBOL_GPL(rtc_device_register);
|
|
|
|
|
|
/**
|
|
* rtc_device_unregister - removes the previously registered RTC class device
|
|
*
|
|
* @rtc: the RTC class device to destroy
|
|
*/
|
|
void rtc_device_unregister(struct rtc_device *rtc)
|
|
{
|
|
if (get_device(&rtc->dev) != NULL) {
|
|
mutex_lock(&rtc->ops_lock);
|
|
/* remove innards of this RTC, then disable it, before
|
|
* letting any rtc_class_open() users access it again
|
|
*/
|
|
rtc_sysfs_del_device(rtc);
|
|
rtc_dev_del_device(rtc);
|
|
rtc_proc_del_device(rtc);
|
|
device_unregister(&rtc->dev);
|
|
rtc->ops = NULL;
|
|
mutex_unlock(&rtc->ops_lock);
|
|
put_device(&rtc->dev);
|
|
}
|
|
}
|
|
EXPORT_SYMBOL_GPL(rtc_device_unregister);
|
|
|
|
static int __init rtc_init(void)
|
|
{
|
|
rtc_class = class_create(THIS_MODULE, "rtc");
|
|
if (IS_ERR(rtc_class)) {
|
|
printk(KERN_ERR "%s: couldn't create class\n", __FILE__);
|
|
return PTR_ERR(rtc_class);
|
|
}
|
|
rtc_class->suspend = rtc_suspend;
|
|
rtc_class->resume = rtc_resume;
|
|
rtc_dev_init();
|
|
rtc_sysfs_init(rtc_class);
|
|
return 0;
|
|
}
|
|
|
|
static void __exit rtc_exit(void)
|
|
{
|
|
rtc_dev_exit();
|
|
class_destroy(rtc_class);
|
|
}
|
|
|
|
subsys_initcall(rtc_init);
|
|
module_exit(rtc_exit);
|
|
|
|
MODULE_AUTHOR("Alessandro Zummo <a.zummo@towertech.it>");
|
|
MODULE_DESCRIPTION("RTC class support");
|
|
MODULE_LICENSE("GPL");
|