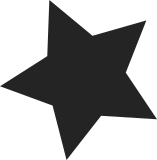
platform_cpu_die() is entered from the CPU's own idle thread, which can not be migrated to other CPUs. Moreover, the 'cpu' argument comes from the thread info, which will always be the 'current' CPU. So remove this useless bug check. Signed-off-by: Russell King <rmk+kernel@arm.linux.org.uk>
60 lines
1.1 KiB
C
60 lines
1.1 KiB
C
/*
|
|
* Copyright (C) STMicroelectronics 2009
|
|
* Copyright (C) ST-Ericsson SA 2010
|
|
*
|
|
* License Terms: GNU General Public License v2
|
|
* Based on ARM realview platform
|
|
*
|
|
* Author: Sundar Iyer <sundar.iyer@stericsson.com>
|
|
*
|
|
*/
|
|
#include <linux/kernel.h>
|
|
#include <linux/errno.h>
|
|
#include <linux/smp.h>
|
|
|
|
#include <asm/cacheflush.h>
|
|
|
|
extern volatile int pen_release;
|
|
|
|
static inline void platform_do_lowpower(unsigned int cpu)
|
|
{
|
|
flush_cache_all();
|
|
|
|
/* we put the platform to just WFI */
|
|
for (;;) {
|
|
__asm__ __volatile__("dsb\n\t" "wfi\n\t"
|
|
: : : "memory");
|
|
if (pen_release == cpu) {
|
|
/*
|
|
* OK, proper wakeup, we're done
|
|
*/
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
|
|
int platform_cpu_kill(unsigned int cpu)
|
|
{
|
|
return 1;
|
|
}
|
|
|
|
/*
|
|
* platform-specific code to shutdown a CPU
|
|
*
|
|
* Called with IRQs disabled
|
|
*/
|
|
void platform_cpu_die(unsigned int cpu)
|
|
{
|
|
/* directly enter low power state, skipping secure registers */
|
|
platform_do_lowpower(cpu);
|
|
}
|
|
|
|
int platform_cpu_disable(unsigned int cpu)
|
|
{
|
|
/*
|
|
* we don't allow CPU 0 to be shutdown (it is still too special
|
|
* e.g. clock tick interrupts)
|
|
*/
|
|
return cpu == 0 ? -EPERM : 0;
|
|
}
|