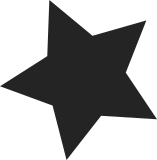
Certain system process significant unconnected UDP workload. It would be preferrable to disable UDP early demux for those systems and enable it for TCP only. By disabling UDP demux, we see these slight gains on an ARM64 system- 782 -> 788Mbps unconnected single stream UDPv4 633 -> 654Mbps unconnected UDPv4 different sources The performance impact can change based on CPU architecure and cache sizes. There will not much difference seen if entire UDP hash table is in cache. Both sysctls are enabled by default to preserve existing behavior. v1->v2: Change function pointer instead of adding conditional as suggested by Stephen. v2->v3: Read once in callers to avoid issues due to compiler optimizations. Also update commit message with the tests. v3->v4: Store and use read once result instead of querying pointer again incorrectly. v4->v5: Refactor to avoid errors due to compilation with IPV6={m,n} Signed-off-by: Subash Abhinov Kasiviswanathan <subashab@codeaurora.org> Suggested-by: Eric Dumazet <edumazet@google.com> Cc: Stephen Hemminger <stephen@networkplumber.org> Cc: Tom Herbert <tom@herbertland.com> Cc: David Miller <davem@davemloft.net> Signed-off-by: David S. Miller <davem@davemloft.net>
75 lines
2.1 KiB
C
75 lines
2.1 KiB
C
/*
|
|
* INET An implementation of the TCP/IP protocol suite for the LINUX
|
|
* operating system. INET is implemented using the BSD Socket
|
|
* interface as the means of communication with the user level.
|
|
*
|
|
* PF_INET6 protocol dispatch tables.
|
|
*
|
|
* Authors: Pedro Roque <roque@di.fc.ul.pt>
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version
|
|
* 2 of the License, or (at your option) any later version.
|
|
*/
|
|
|
|
/*
|
|
* Changes:
|
|
*
|
|
* Vince Laviano (vince@cs.stanford.edu) 16 May 2001
|
|
* - Removed unused variable 'inet6_protocol_base'
|
|
* - Modified inet6_del_protocol() to correctly maintain copy bit.
|
|
*/
|
|
#include <linux/module.h>
|
|
#include <linux/netdevice.h>
|
|
#include <linux/spinlock.h>
|
|
#include <net/protocol.h>
|
|
|
|
#if IS_ENABLED(CONFIG_IPV6)
|
|
struct inet6_protocol __rcu *inet6_protos[MAX_INET_PROTOS] __read_mostly;
|
|
EXPORT_SYMBOL(inet6_protos);
|
|
|
|
int inet6_add_protocol(const struct inet6_protocol *prot, unsigned char protocol)
|
|
{
|
|
return !cmpxchg((const struct inet6_protocol **)&inet6_protos[protocol],
|
|
NULL, prot) ? 0 : -1;
|
|
}
|
|
EXPORT_SYMBOL(inet6_add_protocol);
|
|
|
|
int inet6_del_protocol(const struct inet6_protocol *prot, unsigned char protocol)
|
|
{
|
|
int ret;
|
|
|
|
ret = (cmpxchg((const struct inet6_protocol **)&inet6_protos[protocol],
|
|
prot, NULL) == prot) ? 0 : -1;
|
|
|
|
synchronize_net();
|
|
|
|
return ret;
|
|
}
|
|
EXPORT_SYMBOL(inet6_del_protocol);
|
|
#endif
|
|
|
|
const struct net_offload __rcu *inet6_offloads[MAX_INET_PROTOS] __read_mostly;
|
|
EXPORT_SYMBOL(inet6_offloads);
|
|
|
|
int inet6_add_offload(const struct net_offload *prot, unsigned char protocol)
|
|
{
|
|
return !cmpxchg((const struct net_offload **)&inet6_offloads[protocol],
|
|
NULL, prot) ? 0 : -1;
|
|
}
|
|
EXPORT_SYMBOL(inet6_add_offload);
|
|
|
|
int inet6_del_offload(const struct net_offload *prot, unsigned char protocol)
|
|
{
|
|
int ret;
|
|
|
|
ret = (cmpxchg((const struct net_offload **)&inet6_offloads[protocol],
|
|
prot, NULL) == prot) ? 0 : -1;
|
|
|
|
synchronize_net();
|
|
|
|
return ret;
|
|
}
|
|
EXPORT_SYMBOL(inet6_del_offload);
|