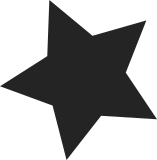
If the bearer carrying multicast messages supports broadcast, those messages will be sent to all cluster nodes, irrespective of whether these nodes host any actual destinations socket or not. This is clearly wasteful if the cluster is large and there are only a few real destinations for the message being sent. In this commit we extend the eligibility of the newly introduced "replicast" transmit option. We now make it possible for a user to select which method he wants to be used, either as a mandatory setting via setsockopt(), or as a relative setting where we let the broadcast layer decide which method to use based on the ratio between cluster size and the message's actual number of destination nodes. In the latter case, a sending socket must stick to a previously selected method until it enters an idle period of at least 5 seconds. This eliminates the risk of message reordering caused by method change, i.e., when changes to cluster size or number of destinations would otherwise mandate a new method to be used. Reviewed-by: Parthasarathy Bhuvaragan <parthasarathy.bhuvaragan@ericsson.com> Acked-by: Ying Xue <ying.xue@windriver.com> Signed-off-by: Jon Maloy <jon.maloy@ericsson.com> Signed-off-by: David S. Miller <davem@davemloft.net>
111 lines
4 KiB
C
111 lines
4 KiB
C
/*
|
|
* net/tipc/bcast.h: Include file for TIPC broadcast code
|
|
*
|
|
* Copyright (c) 2003-2006, 2014-2015, Ericsson AB
|
|
* Copyright (c) 2005, 2010-2011, Wind River Systems
|
|
* All rights reserved.
|
|
*
|
|
* Redistribution and use in source and binary forms, with or without
|
|
* modification, are permitted provided that the following conditions are met:
|
|
*
|
|
* 1. Redistributions of source code must retain the above copyright
|
|
* notice, this list of conditions and the following disclaimer.
|
|
* 2. Redistributions in binary form must reproduce the above copyright
|
|
* notice, this list of conditions and the following disclaimer in the
|
|
* documentation and/or other materials provided with the distribution.
|
|
* 3. Neither the names of the copyright holders nor the names of its
|
|
* contributors may be used to endorse or promote products derived from
|
|
* this software without specific prior written permission.
|
|
*
|
|
* Alternatively, this software may be distributed under the terms of the
|
|
* GNU General Public License ("GPL") version 2 as published by the Free
|
|
* Software Foundation.
|
|
*
|
|
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
|
|
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
|
|
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
|
|
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
|
|
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
|
|
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
|
|
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
|
|
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
|
|
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
|
|
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
|
|
* POSSIBILITY OF SUCH DAMAGE.
|
|
*/
|
|
|
|
#ifndef _TIPC_BCAST_H
|
|
#define _TIPC_BCAST_H
|
|
|
|
#include "core.h"
|
|
|
|
struct tipc_node;
|
|
struct tipc_msg;
|
|
struct tipc_nl_msg;
|
|
struct tipc_nlist;
|
|
struct tipc_nitem;
|
|
extern const char tipc_bclink_name[];
|
|
|
|
#define TIPC_METHOD_EXPIRE msecs_to_jiffies(5000)
|
|
|
|
struct tipc_nlist {
|
|
struct list_head list;
|
|
u32 self;
|
|
u16 remote;
|
|
bool local;
|
|
};
|
|
|
|
void tipc_nlist_init(struct tipc_nlist *nl, u32 self);
|
|
void tipc_nlist_purge(struct tipc_nlist *nl);
|
|
void tipc_nlist_add(struct tipc_nlist *nl, u32 node);
|
|
void tipc_nlist_del(struct tipc_nlist *nl, u32 node);
|
|
|
|
/* Cookie to be used between socket and broadcast layer
|
|
* @rcast: replicast (instead of broadcast) was used at previous xmit
|
|
* @mandatory: broadcast/replicast indication was set by user
|
|
* @expires: re-evaluate non-mandatory transmit method if we are past this
|
|
*/
|
|
struct tipc_mc_method {
|
|
bool rcast;
|
|
bool mandatory;
|
|
unsigned long expires;
|
|
};
|
|
|
|
int tipc_bcast_init(struct net *net);
|
|
void tipc_bcast_stop(struct net *net);
|
|
void tipc_bcast_add_peer(struct net *net, struct tipc_link *l,
|
|
struct sk_buff_head *xmitq);
|
|
void tipc_bcast_remove_peer(struct net *net, struct tipc_link *rcv_bcl);
|
|
void tipc_bcast_inc_bearer_dst_cnt(struct net *net, int bearer_id);
|
|
void tipc_bcast_dec_bearer_dst_cnt(struct net *net, int bearer_id);
|
|
int tipc_bcast_get_mtu(struct net *net);
|
|
void tipc_bcast_disable_rcast(struct net *net);
|
|
int tipc_mcast_xmit(struct net *net, struct sk_buff_head *pkts,
|
|
struct tipc_mc_method *method, struct tipc_nlist *dests,
|
|
u16 *cong_link_cnt);
|
|
int tipc_bcast_rcv(struct net *net, struct tipc_link *l, struct sk_buff *skb);
|
|
void tipc_bcast_ack_rcv(struct net *net, struct tipc_link *l,
|
|
struct tipc_msg *hdr);
|
|
int tipc_bcast_sync_rcv(struct net *net, struct tipc_link *l,
|
|
struct tipc_msg *hdr);
|
|
int tipc_nl_add_bc_link(struct net *net, struct tipc_nl_msg *msg);
|
|
int tipc_nl_bc_link_set(struct net *net, struct nlattr *attrs[]);
|
|
int tipc_bclink_reset_stats(struct net *net);
|
|
|
|
static inline void tipc_bcast_lock(struct net *net)
|
|
{
|
|
spin_lock_bh(&tipc_net(net)->bclock);
|
|
}
|
|
|
|
static inline void tipc_bcast_unlock(struct net *net)
|
|
{
|
|
spin_unlock_bh(&tipc_net(net)->bclock);
|
|
}
|
|
|
|
static inline struct tipc_link *tipc_bc_sndlink(struct net *net)
|
|
{
|
|
return tipc_net(net)->bcl;
|
|
}
|
|
|
|
#endif
|