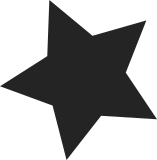
Arnd Bergmann reported a (false positive) objtool warning:
drivers/infiniband/sw/rxe/rxe_resp.o: warning: objtool: rxe_responder()+0xfe: sibling call from callable instruction with changed frame pointer
The issue is in find_switch_table(). It tries to find a switch
statement's jump table by walking backwards from an indirect jump
instruction, looking for a relocation to the .rodata section. In this
case it stopped walking prematurely: the first .rodata relocation it
encountered was for a variable (resp_state_name) instead of a jump
table, so it just assumed there wasn't a jump table.
The fix is to ignore any .rodata relocation which refers to an ELF
object symbol. This works because the jump tables are anonymous and
have no symbols associated with them.
Reported-by: Arnd Bergmann <arnd@arndb.de>
Tested-by: Arnd Bergmann <arnd@arndb.de>
Signed-off-by: Josh Poimboeuf <jpoimboe@redhat.com>
Cc: Denys Vlasenko <dvlasenk@redhat.com>
Cc: Linus Torvalds <torvalds@linux-foundation.org>
Cc: Peter Zijlstra <peterz@infradead.org>
Cc: Thomas Gleixner <tglx@linutronix.de>
Fixes: 3732710ff6
("objtool: Improve rare switch jump table pattern detection")
Link: http://lkml.kernel.org/r/20170302225723.3ndbsnl4hkqbne7a@treble
Signed-off-by: Ingo Molnar <mingo@kernel.org>
92 lines
2.3 KiB
C
92 lines
2.3 KiB
C
/*
|
|
* Copyright (C) 2015 Josh Poimboeuf <jpoimboe@redhat.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2
|
|
* of the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef _OBJTOOL_ELF_H
|
|
#define _OBJTOOL_ELF_H
|
|
|
|
#include <stdio.h>
|
|
#include <gelf.h>
|
|
#include <linux/list.h>
|
|
#include <linux/hashtable.h>
|
|
|
|
#ifdef LIBELF_USE_DEPRECATED
|
|
# define elf_getshdrnum elf_getshnum
|
|
# define elf_getshdrstrndx elf_getshstrndx
|
|
#endif
|
|
|
|
struct section {
|
|
struct list_head list;
|
|
GElf_Shdr sh;
|
|
struct list_head symbol_list;
|
|
DECLARE_HASHTABLE(symbol_hash, 8);
|
|
struct list_head rela_list;
|
|
DECLARE_HASHTABLE(rela_hash, 16);
|
|
struct section *base, *rela;
|
|
struct symbol *sym;
|
|
Elf_Data *elf_data;
|
|
char *name;
|
|
int idx;
|
|
unsigned long data;
|
|
unsigned int len;
|
|
};
|
|
|
|
struct symbol {
|
|
struct list_head list;
|
|
struct hlist_node hash;
|
|
GElf_Sym sym;
|
|
struct section *sec;
|
|
char *name;
|
|
unsigned int idx;
|
|
unsigned char bind, type;
|
|
unsigned long offset;
|
|
unsigned int len;
|
|
};
|
|
|
|
struct rela {
|
|
struct list_head list;
|
|
struct hlist_node hash;
|
|
GElf_Rela rela;
|
|
struct symbol *sym;
|
|
unsigned int type;
|
|
unsigned long offset;
|
|
int addend;
|
|
};
|
|
|
|
struct elf {
|
|
Elf *elf;
|
|
GElf_Ehdr ehdr;
|
|
int fd;
|
|
char *name;
|
|
struct list_head sections;
|
|
DECLARE_HASHTABLE(rela_hash, 16);
|
|
};
|
|
|
|
|
|
struct elf *elf_open(const char *name);
|
|
struct section *find_section_by_name(struct elf *elf, const char *name);
|
|
struct symbol *find_symbol_by_offset(struct section *sec, unsigned long offset);
|
|
struct symbol *find_symbol_containing(struct section *sec, unsigned long offset);
|
|
struct rela *find_rela_by_dest(struct section *sec, unsigned long offset);
|
|
struct rela *find_rela_by_dest_range(struct section *sec, unsigned long offset,
|
|
unsigned int len);
|
|
struct symbol *find_containing_func(struct section *sec, unsigned long offset);
|
|
void elf_close(struct elf *elf);
|
|
|
|
|
|
|
|
#endif /* _OBJTOOL_ELF_H */
|