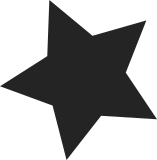
There are 3 big benefits to suballocating a single big DMA buffer for command submission: 1. Avoid hammering CMA. The old way of allocating and freeing a DMA buffer for each submission was hitting some of the real slow pathes in CMA, as this allocator was not designed for a concurrent small buffers load. 2. Less TLB flushes on IOMMUv2. If a new command buffer is mapped into the GPU address space the MMU TLBs need to be flushed. By having one big buffer statically mapped to the GPU, a lot of those flushes can be avoided. 3. No funky workarounds for GC3000. The FE TLB flush on GC3000 isn't reliable. To work around that we tried to lay out the cmdbufs in the GPU address space in a way to avoid this issue. This hasn't always worked if the address space is crowded. A single statically mapped buffer avoids the erratum completely. Signed-off-by: Lucas Stach <l.stach@pengutronix.de> Reviewed-by: Christian Gmeiner <christian.gmeiner@gmail.com>
79 lines
2.4 KiB
C
79 lines
2.4 KiB
C
/*
|
|
* Copyright (C) 2015 Etnaviv Project
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License version 2 as published by
|
|
* the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but WITHOUT
|
|
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
|
|
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for
|
|
* more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along with
|
|
* this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef __ETNAVIV_MMU_H__
|
|
#define __ETNAVIV_MMU_H__
|
|
|
|
#include <linux/iommu.h>
|
|
|
|
enum etnaviv_iommu_version {
|
|
ETNAVIV_IOMMU_V1 = 0,
|
|
ETNAVIV_IOMMU_V2,
|
|
};
|
|
|
|
struct etnaviv_gpu;
|
|
struct etnaviv_vram_mapping;
|
|
|
|
struct etnaviv_iommu_ops {
|
|
struct iommu_ops ops;
|
|
size_t (*dump_size)(struct iommu_domain *);
|
|
void (*dump)(struct iommu_domain *, void *);
|
|
};
|
|
|
|
struct etnaviv_iommu {
|
|
struct etnaviv_gpu *gpu;
|
|
struct iommu_domain *domain;
|
|
|
|
enum etnaviv_iommu_version version;
|
|
|
|
/* memory manager for GPU address area */
|
|
struct mutex lock;
|
|
struct list_head mappings;
|
|
struct drm_mm mm;
|
|
u32 last_iova;
|
|
bool need_flush;
|
|
};
|
|
|
|
struct etnaviv_gem_object;
|
|
|
|
int etnaviv_iommu_attach(struct etnaviv_iommu *iommu, const char **names,
|
|
int cnt);
|
|
int etnaviv_iommu_map(struct etnaviv_iommu *iommu, u32 iova,
|
|
struct sg_table *sgt, unsigned len, int prot);
|
|
int etnaviv_iommu_unmap(struct etnaviv_iommu *iommu, u32 iova,
|
|
struct sg_table *sgt, unsigned len);
|
|
int etnaviv_iommu_map_gem(struct etnaviv_iommu *mmu,
|
|
struct etnaviv_gem_object *etnaviv_obj, u32 memory_base,
|
|
struct etnaviv_vram_mapping *mapping);
|
|
void etnaviv_iommu_unmap_gem(struct etnaviv_iommu *mmu,
|
|
struct etnaviv_vram_mapping *mapping);
|
|
void etnaviv_iommu_destroy(struct etnaviv_iommu *iommu);
|
|
|
|
int etnaviv_iommu_get_suballoc_va(struct etnaviv_gpu *gpu, dma_addr_t paddr,
|
|
struct drm_mm_node *vram_node, size_t size,
|
|
u32 *iova);
|
|
void etnaviv_iommu_put_suballoc_va(struct etnaviv_gpu *gpu,
|
|
struct drm_mm_node *vram_node, size_t size,
|
|
u32 iova);
|
|
|
|
size_t etnaviv_iommu_dump_size(struct etnaviv_iommu *iommu);
|
|
void etnaviv_iommu_dump(struct etnaviv_iommu *iommu, void *buf);
|
|
|
|
struct etnaviv_iommu *etnaviv_iommu_new(struct etnaviv_gpu *gpu);
|
|
void etnaviv_iommu_restore(struct etnaviv_gpu *gpu);
|
|
|
|
#endif /* __ETNAVIV_MMU_H__ */
|