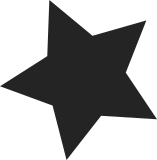
This patch fixes regressions in588bff95c9
. Due to that patch, function clean_journal was setting the value of sd_log_flush_head, but that's only valid if it is replaying the node's own journal. If it's replaying another node's journal, that's completely wrong and will lead to multiple problems. This patch tries to clean up the mess by passing the value of the logical journal block number into gfs2_write_log_header so the function can treat non-owned journals generically. For the local journal, the journal extent map is used for best performance. For other nodes from other journals, new function gfs2_lblk_to_dblk is called to figure it out using gfs2_iomap_get. This patch also tries to establish more consistency when passing journal block parameters by changing several unsigned int types to a consistent u32. Fixes:588bff95c9
("GFS2: Reduce code redundancy writing log headers") Signed-off-by: Bob Peterson <rpeterso@redhat.com> Signed-off-by: Andreas Gruenbacher <agruenba@redhat.com>
70 lines
2.3 KiB
C
70 lines
2.3 KiB
C
/*
|
|
* Copyright (C) Sistina Software, Inc. 1997-2003 All rights reserved.
|
|
* Copyright (C) 2004-2006 Red Hat, Inc. All rights reserved.
|
|
*
|
|
* This copyrighted material is made available to anyone wishing to use,
|
|
* modify, copy, or redistribute it subject to the terms and conditions
|
|
* of the GNU General Public License version 2.
|
|
*/
|
|
|
|
#ifndef __BMAP_DOT_H__
|
|
#define __BMAP_DOT_H__
|
|
|
|
#include <linux/iomap.h>
|
|
|
|
#include "inode.h"
|
|
|
|
struct inode;
|
|
struct gfs2_inode;
|
|
struct page;
|
|
|
|
|
|
/**
|
|
* gfs2_write_calc_reserv - calculate number of blocks needed to write to a file
|
|
* @ip: the file
|
|
* @len: the number of bytes to be written to the file
|
|
* @data_blocks: returns the number of data blocks required
|
|
* @ind_blocks: returns the number of indirect blocks required
|
|
*
|
|
*/
|
|
|
|
static inline void gfs2_write_calc_reserv(const struct gfs2_inode *ip,
|
|
unsigned int len,
|
|
unsigned int *data_blocks,
|
|
unsigned int *ind_blocks)
|
|
{
|
|
const struct gfs2_sbd *sdp = GFS2_SB(&ip->i_inode);
|
|
unsigned int tmp;
|
|
|
|
BUG_ON(gfs2_is_dir(ip));
|
|
*data_blocks = (len >> sdp->sd_sb.sb_bsize_shift) + 3;
|
|
*ind_blocks = 3 * (sdp->sd_max_height - 1);
|
|
|
|
for (tmp = *data_blocks; tmp > sdp->sd_diptrs;) {
|
|
tmp = DIV_ROUND_UP(tmp, sdp->sd_inptrs);
|
|
*ind_blocks += tmp;
|
|
}
|
|
}
|
|
|
|
extern const struct iomap_ops gfs2_iomap_ops;
|
|
|
|
extern int gfs2_unstuff_dinode(struct gfs2_inode *ip, struct page *page);
|
|
extern int gfs2_block_map(struct inode *inode, sector_t lblock,
|
|
struct buffer_head *bh, int create);
|
|
extern int gfs2_iomap_get_alloc(struct inode *inode, loff_t pos, loff_t length,
|
|
struct iomap *iomap);
|
|
extern int gfs2_extent_map(struct inode *inode, u64 lblock, int *new,
|
|
u64 *dblock, unsigned *extlen);
|
|
extern int gfs2_setattr_size(struct inode *inode, u64 size);
|
|
extern void gfs2_trim_blocks(struct inode *inode);
|
|
extern int gfs2_truncatei_resume(struct gfs2_inode *ip);
|
|
extern int gfs2_file_dealloc(struct gfs2_inode *ip);
|
|
extern int gfs2_write_alloc_required(struct gfs2_inode *ip, u64 offset,
|
|
unsigned int len);
|
|
extern int gfs2_map_journal_extents(struct gfs2_sbd *sdp, struct gfs2_jdesc *jd);
|
|
extern void gfs2_free_journal_extents(struct gfs2_jdesc *jd);
|
|
extern int __gfs2_punch_hole(struct file *file, loff_t offset, loff_t length);
|
|
extern int gfs2_lblk_to_dblk(struct inode *inode, u32 lblock, u64 *dblock);
|
|
|
|
#endif /* __BMAP_DOT_H__ */
|