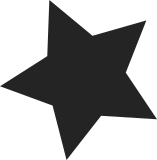
dirent modification events (create/delete/move) do not carry the child entry name/inode information. Instead, we report FAN_ONDIR for mkdir/rmdir so user can differentiate them from creat/unlink. This is consistent with inotify reporting IN_ISDIR with dirent events and is useful for implementing recursive directory tree watcher. We avoid merging dirent events referring to subdirs with dirent events referring to non subdirs, otherwise, user won't be able to tell from a mask FAN_CREATE|FAN_DELETE|FAN_ONDIR if it describes mkdir+unlink pair or rmdir+create pair of events. For backward compatibility and consistency, do not report FAN_ONDIR to user in legacy fanotify mode (reporting fd) and report FAN_ONDIR to user in FAN_REPORT_FID mode for all event types. Cc: <linux-api@vger.kernel.org> Signed-off-by: Amir Goldstein <amir73il@gmail.com> Signed-off-by: Jan Kara <jack@suse.cz>
84 lines
2.7 KiB
C
84 lines
2.7 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _LINUX_FANOTIFY_H
|
|
#define _LINUX_FANOTIFY_H
|
|
|
|
#include <uapi/linux/fanotify.h>
|
|
|
|
#define FAN_GROUP_FLAG(group, flag) \
|
|
((group)->fanotify_data.flags & (flag))
|
|
|
|
/*
|
|
* Flags allowed to be passed from/to userspace.
|
|
*
|
|
* We intentionally do not add new bits to the old FAN_ALL_* constants, because
|
|
* they are uapi exposed constants. If there are programs out there using
|
|
* these constant, the programs may break if re-compiled with new uapi headers
|
|
* and then run on an old kernel.
|
|
*/
|
|
#define FANOTIFY_CLASS_BITS (FAN_CLASS_NOTIF | FAN_CLASS_CONTENT | \
|
|
FAN_CLASS_PRE_CONTENT)
|
|
|
|
#define FANOTIFY_INIT_FLAGS (FANOTIFY_CLASS_BITS | \
|
|
FAN_REPORT_TID | FAN_REPORT_FID | \
|
|
FAN_CLOEXEC | FAN_NONBLOCK | \
|
|
FAN_UNLIMITED_QUEUE | FAN_UNLIMITED_MARKS)
|
|
|
|
#define FANOTIFY_MARK_TYPE_BITS (FAN_MARK_INODE | FAN_MARK_MOUNT | \
|
|
FAN_MARK_FILESYSTEM)
|
|
|
|
#define FANOTIFY_MARK_FLAGS (FANOTIFY_MARK_TYPE_BITS | \
|
|
FAN_MARK_ADD | \
|
|
FAN_MARK_REMOVE | \
|
|
FAN_MARK_DONT_FOLLOW | \
|
|
FAN_MARK_ONLYDIR | \
|
|
FAN_MARK_IGNORED_MASK | \
|
|
FAN_MARK_IGNORED_SURV_MODIFY | \
|
|
FAN_MARK_FLUSH)
|
|
|
|
/*
|
|
* Events that can be reported with data type FSNOTIFY_EVENT_PATH.
|
|
* Note that FAN_MODIFY can also be reported with data type
|
|
* FSNOTIFY_EVENT_INODE.
|
|
*/
|
|
#define FANOTIFY_PATH_EVENTS (FAN_ACCESS | FAN_MODIFY | \
|
|
FAN_CLOSE | FAN_OPEN | FAN_OPEN_EXEC)
|
|
|
|
/*
|
|
* Directory entry modification events - reported only to directory
|
|
* where entry is modified and not to a watching parent.
|
|
*/
|
|
#define FANOTIFY_DIRENT_EVENTS (FAN_MOVE | FAN_CREATE | FAN_DELETE)
|
|
|
|
/* Events that can only be reported with data type FSNOTIFY_EVENT_INODE */
|
|
#define FANOTIFY_INODE_EVENTS (FANOTIFY_DIRENT_EVENTS | \
|
|
FAN_ATTRIB | FAN_MOVE_SELF | FAN_DELETE_SELF)
|
|
|
|
/* Events that user can request to be notified on */
|
|
#define FANOTIFY_EVENTS (FANOTIFY_PATH_EVENTS | \
|
|
FANOTIFY_INODE_EVENTS)
|
|
|
|
/* Events that require a permission response from user */
|
|
#define FANOTIFY_PERM_EVENTS (FAN_OPEN_PERM | FAN_ACCESS_PERM | \
|
|
FAN_OPEN_EXEC_PERM)
|
|
|
|
/* Extra flags that may be reported with event or control handling of events */
|
|
#define FANOTIFY_EVENT_FLAGS (FAN_EVENT_ON_CHILD | FAN_ONDIR)
|
|
|
|
/* Events that may be reported to user */
|
|
#define FANOTIFY_OUTGOING_EVENTS (FANOTIFY_EVENTS | \
|
|
FANOTIFY_PERM_EVENTS | \
|
|
FAN_Q_OVERFLOW | FAN_ONDIR)
|
|
|
|
#define ALL_FANOTIFY_EVENT_BITS (FANOTIFY_OUTGOING_EVENTS | \
|
|
FANOTIFY_EVENT_FLAGS)
|
|
|
|
/* Do not use these old uapi constants internally */
|
|
#undef FAN_ALL_CLASS_BITS
|
|
#undef FAN_ALL_INIT_FLAGS
|
|
#undef FAN_ALL_MARK_FLAGS
|
|
#undef FAN_ALL_EVENTS
|
|
#undef FAN_ALL_PERM_EVENTS
|
|
#undef FAN_ALL_OUTGOING_EVENTS
|
|
|
|
#endif /* _LINUX_FANOTIFY_H */
|