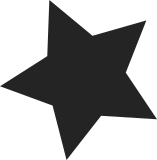
DesignWare v3.65 hardware implements MSI controller registers in application space. This requires updates to the DesignWare core to support controllers based on this older hardware. Add msi_irq_set()/clear() interfaces to allow Set/Clear MSI IRQ enable bit in the application register. Also, v3.65 hardware uses the MSI_IRQ register in application register space to raise MSI IRQ to the RC from EP. Current code uses the standard mechanism as per PCI spec. So add get_msi_data() to get the address of this register so common code can work on both v3.65 and newer hardware. [bhelgaas: changelog] Signed-off-by: Murali Karicheri <m-karicheri2@ti.com> Signed-off-by: Bjorn Helgaas <bhelgaas@google.com> Reviewed-by: Pratyush Anand <pratyush.anand@st.com> Acked-by: Mohit Kumar <mohit.kumar@st.com> Acked-by: Jingoo Han <jg1.han@samsung.com> Acked-by: Santosh Shilimkar <santosh.shilimkar@ti.com> CC: Russell King <linux@arm.linux.org.uk> CC: Grant Likely <grant.likely@linaro.org> CC: Rob Herring <robh+dt@kernel.org> CC: Richard Zhu <r65037@freescale.com> CC: Kishon Vijay Abraham I <kishon@ti.com> CC: Marek Vasut <marex@denx.de> CC: Arnd Bergmann <arnd@arndb.de> CC: Pawel Moll <pawel.moll@arm.com> CC: Mark Rutland <mark.rutland@arm.com> CC: Ian Campbell <ijc+devicetree@hellion.org.uk> CC: Kumar Gala <galak@codeaurora.org> CC: Randy Dunlap <rdunlap@infradead.org> CC: Grant Likely <grant.likely@linaro.org>
88 lines
2.6 KiB
C
88 lines
2.6 KiB
C
/*
|
|
* Synopsys Designware PCIe host controller driver
|
|
*
|
|
* Copyright (C) 2013 Samsung Electronics Co., Ltd.
|
|
* http://www.samsung.com
|
|
*
|
|
* Author: Jingoo Han <jg1.han@samsung.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*/
|
|
|
|
#ifndef _PCIE_DESIGNWARE_H
|
|
#define _PCIE_DESIGNWARE_H
|
|
|
|
struct pcie_port_info {
|
|
u32 cfg0_size;
|
|
u32 cfg1_size;
|
|
u32 io_size;
|
|
u32 mem_size;
|
|
phys_addr_t io_bus_addr;
|
|
phys_addr_t mem_bus_addr;
|
|
};
|
|
|
|
/*
|
|
* Maximum number of MSI IRQs can be 256 per controller. But keep
|
|
* it 32 as of now. Probably we will never need more than 32. If needed,
|
|
* then increment it in multiple of 32.
|
|
*/
|
|
#define MAX_MSI_IRQS 32
|
|
#define MAX_MSI_CTRLS (MAX_MSI_IRQS / 32)
|
|
|
|
struct pcie_port {
|
|
struct device *dev;
|
|
u8 root_bus_nr;
|
|
void __iomem *dbi_base;
|
|
u64 cfg0_base;
|
|
u64 cfg0_mod_base;
|
|
void __iomem *va_cfg0_base;
|
|
u64 cfg1_base;
|
|
u64 cfg1_mod_base;
|
|
void __iomem *va_cfg1_base;
|
|
u64 io_base;
|
|
u64 io_mod_base;
|
|
u64 mem_base;
|
|
u64 mem_mod_base;
|
|
struct resource cfg;
|
|
struct resource io;
|
|
struct resource mem;
|
|
struct pcie_port_info config;
|
|
int irq;
|
|
u32 lanes;
|
|
struct pcie_host_ops *ops;
|
|
int msi_irq;
|
|
struct irq_domain *irq_domain;
|
|
unsigned long msi_data;
|
|
DECLARE_BITMAP(msi_irq_in_use, MAX_MSI_IRQS);
|
|
};
|
|
|
|
struct pcie_host_ops {
|
|
void (*readl_rc)(struct pcie_port *pp,
|
|
void __iomem *dbi_base, u32 *val);
|
|
void (*writel_rc)(struct pcie_port *pp,
|
|
u32 val, void __iomem *dbi_base);
|
|
int (*rd_own_conf)(struct pcie_port *pp, int where, int size, u32 *val);
|
|
int (*wr_own_conf)(struct pcie_port *pp, int where, int size, u32 val);
|
|
int (*rd_other_conf)(struct pcie_port *pp, struct pci_bus *bus,
|
|
unsigned int devfn, int where, int size, u32 *val);
|
|
int (*wr_other_conf)(struct pcie_port *pp, struct pci_bus *bus,
|
|
unsigned int devfn, int where, int size, u32 val);
|
|
int (*link_up)(struct pcie_port *pp);
|
|
void (*host_init)(struct pcie_port *pp);
|
|
void (*msi_set_irq)(struct pcie_port *pp, int irq);
|
|
void (*msi_clear_irq)(struct pcie_port *pp, int irq);
|
|
u32 (*get_msi_data)(struct pcie_port *pp);
|
|
};
|
|
|
|
int dw_pcie_cfg_read(void __iomem *addr, int where, int size, u32 *val);
|
|
int dw_pcie_cfg_write(void __iomem *addr, int where, int size, u32 val);
|
|
irqreturn_t dw_handle_msi_irq(struct pcie_port *pp);
|
|
void dw_pcie_msi_init(struct pcie_port *pp);
|
|
int dw_pcie_link_up(struct pcie_port *pp);
|
|
void dw_pcie_setup_rc(struct pcie_port *pp);
|
|
int dw_pcie_host_init(struct pcie_port *pp);
|
|
|
|
#endif /* _PCIE_DESIGNWARE_H */
|