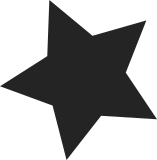
Add check for the return value of memblock_alloc*() functions and call panic() in case of error. The panic message repeats the one used by panicing memblock allocators with adjustment of parameters to include only relevant ones. The replacement was mostly automated with semantic patches like the one below with manual massaging of format strings. @@ expression ptr, size, align; @@ ptr = memblock_alloc(size, align); + if (!ptr) + panic("%s: Failed to allocate %lu bytes align=0x%lx\n", __func__, size, align); [anders.roxell@linaro.org: use '%pa' with 'phys_addr_t' type] Link: http://lkml.kernel.org/r/20190131161046.21886-1-anders.roxell@linaro.org [rppt@linux.ibm.com: fix format strings for panics after memblock_alloc] Link: http://lkml.kernel.org/r/1548950940-15145-1-git-send-email-rppt@linux.ibm.com [rppt@linux.ibm.com: don't panic if the allocation in sparse_buffer_init fails] Link: http://lkml.kernel.org/r/20190131074018.GD28876@rapoport-lnx [akpm@linux-foundation.org: fix xtensa printk warning] Link: http://lkml.kernel.org/r/1548057848-15136-20-git-send-email-rppt@linux.ibm.com Signed-off-by: Mike Rapoport <rppt@linux.ibm.com> Signed-off-by: Anders Roxell <anders.roxell@linaro.org> Reviewed-by: Guo Ren <ren_guo@c-sky.com> [c-sky] Acked-by: Paul Burton <paul.burton@mips.com> [MIPS] Acked-by: Heiko Carstens <heiko.carstens@de.ibm.com> [s390] Reviewed-by: Juergen Gross <jgross@suse.com> [Xen] Reviewed-by: Geert Uytterhoeven <geert@linux-m68k.org> [m68k] Acked-by: Max Filippov <jcmvbkbc@gmail.com> [xtensa] Cc: Catalin Marinas <catalin.marinas@arm.com> Cc: Christophe Leroy <christophe.leroy@c-s.fr> Cc: Christoph Hellwig <hch@lst.de> Cc: "David S. Miller" <davem@davemloft.net> Cc: Dennis Zhou <dennis@kernel.org> Cc: Greentime Hu <green.hu@gmail.com> Cc: Greg Kroah-Hartman <gregkh@linuxfoundation.org> Cc: Guan Xuetao <gxt@pku.edu.cn> Cc: Guo Ren <guoren@kernel.org> Cc: Mark Salter <msalter@redhat.com> Cc: Matt Turner <mattst88@gmail.com> Cc: Michael Ellerman <mpe@ellerman.id.au> Cc: Michal Simek <monstr@monstr.eu> Cc: Petr Mladek <pmladek@suse.com> Cc: Richard Weinberger <richard@nod.at> Cc: Rich Felker <dalias@libc.org> Cc: Rob Herring <robh+dt@kernel.org> Cc: Rob Herring <robh@kernel.org> Cc: Russell King <linux@armlinux.org.uk> Cc: Stafford Horne <shorne@gmail.com> Cc: Tony Luck <tony.luck@intel.com> Cc: Vineet Gupta <vgupta@synopsys.com> Cc: Yoshinori Sato <ysato@users.sourceforge.jp> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
157 lines
3.7 KiB
C
157 lines
3.7 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* linux/arch/m68k/mm/init.c
|
|
*
|
|
* Copyright (C) 1995 Hamish Macdonald
|
|
*
|
|
* Contains common initialization routines, specific init code moved
|
|
* to motorola.c and sun3mmu.c
|
|
*/
|
|
|
|
#include <linux/module.h>
|
|
#include <linux/signal.h>
|
|
#include <linux/sched.h>
|
|
#include <linux/mm.h>
|
|
#include <linux/swap.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/string.h>
|
|
#include <linux/types.h>
|
|
#include <linux/init.h>
|
|
#include <linux/memblock.h>
|
|
#include <linux/gfp.h>
|
|
|
|
#include <asm/setup.h>
|
|
#include <linux/uaccess.h>
|
|
#include <asm/page.h>
|
|
#include <asm/pgalloc.h>
|
|
#include <asm/traps.h>
|
|
#include <asm/machdep.h>
|
|
#include <asm/io.h>
|
|
#ifdef CONFIG_ATARI
|
|
#include <asm/atari_stram.h>
|
|
#endif
|
|
#include <asm/sections.h>
|
|
#include <asm/tlb.h>
|
|
|
|
/*
|
|
* ZERO_PAGE is a special page that is used for zero-initialized
|
|
* data and COW.
|
|
*/
|
|
void *empty_zero_page;
|
|
EXPORT_SYMBOL(empty_zero_page);
|
|
|
|
#if !defined(CONFIG_SUN3) && !defined(CONFIG_COLDFIRE)
|
|
extern void init_pointer_table(unsigned long ptable);
|
|
extern pmd_t *zero_pgtable;
|
|
#endif
|
|
|
|
#ifdef CONFIG_MMU
|
|
|
|
pg_data_t pg_data_map[MAX_NUMNODES];
|
|
EXPORT_SYMBOL(pg_data_map);
|
|
|
|
int m68k_virt_to_node_shift;
|
|
|
|
#ifndef CONFIG_SINGLE_MEMORY_CHUNK
|
|
pg_data_t *pg_data_table[65];
|
|
EXPORT_SYMBOL(pg_data_table);
|
|
#endif
|
|
|
|
void __init m68k_setup_node(int node)
|
|
{
|
|
#ifndef CONFIG_SINGLE_MEMORY_CHUNK
|
|
struct m68k_mem_info *info = m68k_memory + node;
|
|
int i, end;
|
|
|
|
i = (unsigned long)phys_to_virt(info->addr) >> __virt_to_node_shift();
|
|
end = (unsigned long)phys_to_virt(info->addr + info->size - 1) >> __virt_to_node_shift();
|
|
for (; i <= end; i++) {
|
|
if (pg_data_table[i])
|
|
pr_warn("overlap at %u for chunk %u\n", i, node);
|
|
pg_data_table[i] = pg_data_map + node;
|
|
}
|
|
#endif
|
|
node_set_online(node);
|
|
}
|
|
|
|
#else /* CONFIG_MMU */
|
|
|
|
/*
|
|
* paging_init() continues the virtual memory environment setup which
|
|
* was begun by the code in arch/head.S.
|
|
* The parameters are pointers to where to stick the starting and ending
|
|
* addresses of available kernel virtual memory.
|
|
*/
|
|
void __init paging_init(void)
|
|
{
|
|
/*
|
|
* Make sure start_mem is page aligned, otherwise bootmem and
|
|
* page_alloc get different views of the world.
|
|
*/
|
|
unsigned long end_mem = memory_end & PAGE_MASK;
|
|
unsigned long zones_size[MAX_NR_ZONES] = { 0, };
|
|
|
|
high_memory = (void *) end_mem;
|
|
|
|
empty_zero_page = memblock_alloc(PAGE_SIZE, PAGE_SIZE);
|
|
if (!empty_zero_page)
|
|
panic("%s: Failed to allocate %lu bytes align=0x%lx\n",
|
|
__func__, PAGE_SIZE, PAGE_SIZE);
|
|
|
|
/*
|
|
* Set up SFC/DFC registers (user data space).
|
|
*/
|
|
set_fs (USER_DS);
|
|
|
|
zones_size[ZONE_DMA] = (end_mem - PAGE_OFFSET) >> PAGE_SHIFT;
|
|
free_area_init(zones_size);
|
|
}
|
|
|
|
#endif /* CONFIG_MMU */
|
|
|
|
void free_initmem(void)
|
|
{
|
|
#ifndef CONFIG_MMU_SUN3
|
|
free_initmem_default(-1);
|
|
#endif /* CONFIG_MMU_SUN3 */
|
|
}
|
|
|
|
#if defined(CONFIG_MMU) && !defined(CONFIG_COLDFIRE)
|
|
#define VECTORS &vectors[0]
|
|
#else
|
|
#define VECTORS _ramvec
|
|
#endif
|
|
|
|
static inline void init_pointer_tables(void)
|
|
{
|
|
#if defined(CONFIG_MMU) && !defined(CONFIG_SUN3) && !defined(CONFIG_COLDFIRE)
|
|
int i;
|
|
|
|
/* insert pointer tables allocated so far into the tablelist */
|
|
init_pointer_table((unsigned long)kernel_pg_dir);
|
|
for (i = 0; i < PTRS_PER_PGD; i++) {
|
|
if (pgd_present(kernel_pg_dir[i]))
|
|
init_pointer_table(__pgd_page(kernel_pg_dir[i]));
|
|
}
|
|
|
|
/* insert also pointer table that we used to unmap the zero page */
|
|
if (zero_pgtable)
|
|
init_pointer_table((unsigned long)zero_pgtable);
|
|
#endif
|
|
}
|
|
|
|
void __init mem_init(void)
|
|
{
|
|
/* this will put all memory onto the freelists */
|
|
memblock_free_all();
|
|
init_pointer_tables();
|
|
mem_init_print_info(NULL);
|
|
}
|
|
|
|
#ifdef CONFIG_BLK_DEV_INITRD
|
|
void free_initrd_mem(unsigned long start, unsigned long end)
|
|
{
|
|
free_reserved_area((void *)start, (void *)end, -1, "initrd");
|
|
}
|
|
#endif
|