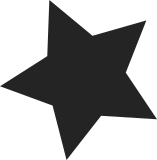
These header files are never installed to user consumption, so any __KERNEL__ cpp checks are superfluous. Projects should also not copy these files into their userland utility sources and try to use them there. If they insist on doing so, the onus is on them to sanitize the headers as needed. Signed-off-by: David S. Miller <davem@davemloft.net>
74 lines
1.8 KiB
C
74 lines
1.8 KiB
C
#ifndef __LINUX_NET_AFUNIX_H
|
|
#define __LINUX_NET_AFUNIX_H
|
|
|
|
#include <linux/socket.h>
|
|
#include <linux/un.h>
|
|
#include <linux/mutex.h>
|
|
#include <net/sock.h>
|
|
|
|
extern void unix_inflight(struct file *fp);
|
|
extern void unix_notinflight(struct file *fp);
|
|
extern void unix_gc(void);
|
|
extern void wait_for_unix_gc(void);
|
|
extern struct sock *unix_get_socket(struct file *filp);
|
|
|
|
#define UNIX_HASH_SIZE 256
|
|
|
|
extern unsigned int unix_tot_inflight;
|
|
|
|
struct unix_address {
|
|
atomic_t refcnt;
|
|
int len;
|
|
unsigned hash;
|
|
struct sockaddr_un name[0];
|
|
};
|
|
|
|
struct unix_skb_parms {
|
|
struct pid *pid; /* Skb credentials */
|
|
const struct cred *cred;
|
|
struct scm_fp_list *fp; /* Passed files */
|
|
#ifdef CONFIG_SECURITY_NETWORK
|
|
u32 secid; /* Security ID */
|
|
#endif
|
|
};
|
|
|
|
#define UNIXCB(skb) (*(struct unix_skb_parms *)&((skb)->cb))
|
|
#define UNIXSID(skb) (&UNIXCB((skb)).secid)
|
|
|
|
#define unix_state_lock(s) spin_lock(&unix_sk(s)->lock)
|
|
#define unix_state_unlock(s) spin_unlock(&unix_sk(s)->lock)
|
|
#define unix_state_lock_nested(s) \
|
|
spin_lock_nested(&unix_sk(s)->lock, \
|
|
SINGLE_DEPTH_NESTING)
|
|
|
|
/* The AF_UNIX socket */
|
|
struct unix_sock {
|
|
/* WARNING: sk has to be the first member */
|
|
struct sock sk;
|
|
struct unix_address *addr;
|
|
struct dentry *dentry;
|
|
struct vfsmount *mnt;
|
|
struct mutex readlock;
|
|
struct sock *peer;
|
|
struct sock *other;
|
|
struct list_head link;
|
|
atomic_long_t inflight;
|
|
spinlock_t lock;
|
|
unsigned int gc_candidate : 1;
|
|
unsigned int gc_maybe_cycle : 1;
|
|
unsigned char recursion_level;
|
|
struct socket_wq peer_wq;
|
|
};
|
|
#define unix_sk(__sk) ((struct unix_sock *)__sk)
|
|
|
|
#define peer_wait peer_wq.wait
|
|
|
|
#ifdef CONFIG_SYSCTL
|
|
extern int unix_sysctl_register(struct net *net);
|
|
extern void unix_sysctl_unregister(struct net *net);
|
|
#else
|
|
static inline int unix_sysctl_register(struct net *net) { return 0; }
|
|
static inline void unix_sysctl_unregister(struct net *net) {}
|
|
#endif
|
|
#endif
|