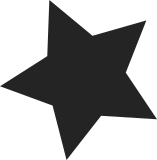
Following changes have been made: 1. Scan outstanding commands only in the queue where it is submitted 2. Update queue registers directly in the fast path 3. Queue specific BAR is remapped only for multiq capable adapters Signed-off-by: Anirban Chakraborty <anirban.chakraborty@qlogic.com> Signed-off-by: James Bottomley <James.Bottomley@HansenPartnership.com>
67 lines
1.4 KiB
C
67 lines
1.4 KiB
C
/*
|
|
* QLogic Fibre Channel HBA Driver
|
|
* Copyright (c) 2003-2008 QLogic Corporation
|
|
*
|
|
* See LICENSE.qla2xxx for copyright and licensing details.
|
|
*/
|
|
|
|
/*
|
|
* qla2x00_debounce_register
|
|
* Debounce register.
|
|
*
|
|
* Input:
|
|
* port = register address.
|
|
*
|
|
* Returns:
|
|
* register value.
|
|
*/
|
|
static __inline__ uint16_t
|
|
qla2x00_debounce_register(volatile uint16_t __iomem *addr)
|
|
{
|
|
volatile uint16_t first;
|
|
volatile uint16_t second;
|
|
|
|
do {
|
|
first = RD_REG_WORD(addr);
|
|
barrier();
|
|
cpu_relax();
|
|
second = RD_REG_WORD(addr);
|
|
} while (first != second);
|
|
|
|
return (first);
|
|
}
|
|
|
|
static inline void
|
|
qla2x00_poll(struct rsp_que *rsp)
|
|
{
|
|
unsigned long flags;
|
|
struct qla_hw_data *ha = rsp->hw;
|
|
local_irq_save(flags);
|
|
ha->isp_ops->intr_handler(0, rsp);
|
|
local_irq_restore(flags);
|
|
}
|
|
|
|
static inline uint8_t *
|
|
host_to_fcp_swap(uint8_t *fcp, uint32_t bsize)
|
|
{
|
|
uint32_t *ifcp = (uint32_t *) fcp;
|
|
uint32_t *ofcp = (uint32_t *) fcp;
|
|
uint32_t iter = bsize >> 2;
|
|
|
|
for (; iter ; iter--)
|
|
*ofcp++ = swab32(*ifcp++);
|
|
|
|
return fcp;
|
|
}
|
|
|
|
static inline int
|
|
qla2x00_is_reserved_id(scsi_qla_host_t *vha, uint16_t loop_id)
|
|
{
|
|
struct qla_hw_data *ha = vha->hw;
|
|
if (IS_FWI2_CAPABLE(ha))
|
|
return (loop_id > NPH_LAST_HANDLE);
|
|
|
|
return ((loop_id > ha->max_loop_id && loop_id < SNS_FIRST_LOOP_ID) ||
|
|
loop_id == MANAGEMENT_SERVER || loop_id == BROADCAST);
|
|
}
|