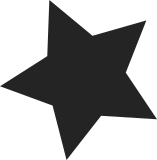
Make do_execve() take a const filename pointer so that kernel_execve() compiles correctly on ARM: arch/arm/kernel/sys_arm.c:88: warning: passing argument 1 of 'do_execve' discards qualifiers from pointer target type This also requires the argv and envp arguments to be consted twice, once for the pointer array and once for the strings the array points to. This is because do_execve() passes a pointer to the filename (now const) to copy_strings_kernel(). A simpler alternative would be to cast the filename pointer in do_execve() when it's passed to copy_strings_kernel(). do_execve() may not change any of the strings it is passed as part of the argv or envp lists as they are some of them in .rodata, so marking these strings as const should be fine. Further kernel_execve() and sys_execve() need to be changed to match. This has been test built on x86_64, frv, arm and mips. Signed-off-by: David Howells <dhowells@redhat.com> Tested-by: Ralf Baechle <ralf@linux-mips.org> Acked-by: Russell King <rmk+kernel@arm.linux.org.uk> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
70 lines
1.9 KiB
C
70 lines
1.9 KiB
C
/*
|
|
* syscalls.h - Linux syscall interfaces (arch-specific)
|
|
*
|
|
* Copyright (c) 2008 Jaswinder Singh Rajput
|
|
*
|
|
* This file is released under the GPLv2.
|
|
* See the file COPYING for more details.
|
|
*/
|
|
|
|
#ifndef _ASM_X86_SYSCALLS_H
|
|
#define _ASM_X86_SYSCALLS_H
|
|
|
|
#include <linux/compiler.h>
|
|
#include <linux/linkage.h>
|
|
#include <linux/signal.h>
|
|
#include <linux/types.h>
|
|
|
|
/* Common in X86_32 and X86_64 */
|
|
/* kernel/ioport.c */
|
|
asmlinkage long sys_ioperm(unsigned long, unsigned long, int);
|
|
long sys_iopl(unsigned int, struct pt_regs *);
|
|
|
|
/* kernel/process.c */
|
|
int sys_fork(struct pt_regs *);
|
|
int sys_vfork(struct pt_regs *);
|
|
long sys_execve(const char __user *,
|
|
const char __user *const __user *,
|
|
const char __user *const __user *, struct pt_regs *);
|
|
long sys_clone(unsigned long, unsigned long, void __user *,
|
|
void __user *, struct pt_regs *);
|
|
|
|
/* kernel/ldt.c */
|
|
asmlinkage int sys_modify_ldt(int, void __user *, unsigned long);
|
|
|
|
/* kernel/signal.c */
|
|
long sys_rt_sigreturn(struct pt_regs *);
|
|
long sys_sigaltstack(const stack_t __user *, stack_t __user *,
|
|
struct pt_regs *);
|
|
|
|
|
|
/* kernel/tls.c */
|
|
asmlinkage int sys_set_thread_area(struct user_desc __user *);
|
|
asmlinkage int sys_get_thread_area(struct user_desc __user *);
|
|
|
|
/* X86_32 only */
|
|
#ifdef CONFIG_X86_32
|
|
|
|
/* kernel/signal.c */
|
|
asmlinkage int sys_sigsuspend(int, int, old_sigset_t);
|
|
asmlinkage int sys_sigaction(int, const struct old_sigaction __user *,
|
|
struct old_sigaction __user *);
|
|
unsigned long sys_sigreturn(struct pt_regs *);
|
|
|
|
/* kernel/vm86_32.c */
|
|
int sys_vm86old(struct vm86_struct __user *, struct pt_regs *);
|
|
int sys_vm86(unsigned long, unsigned long, struct pt_regs *);
|
|
|
|
#else /* CONFIG_X86_32 */
|
|
|
|
/* X86_64 only */
|
|
/* kernel/process_64.c */
|
|
long sys_arch_prctl(int, unsigned long);
|
|
|
|
/* kernel/sys_x86_64.c */
|
|
asmlinkage long sys_mmap(unsigned long, unsigned long, unsigned long,
|
|
unsigned long, unsigned long, unsigned long);
|
|
|
|
#endif /* CONFIG_X86_32 */
|
|
#endif /* _ASM_X86_SYSCALLS_H */
|