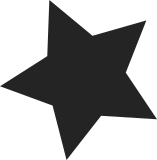
Formerly, we used to maintain a per-vcpu shadow TLB and on every entry to the guest would load this array into the hardware TLB. This consumed 1280 bytes of memory (64 entries of 16 bytes plus a struct page pointer each), and also required some assembly to loop over the array on every entry. Instead of saving a copy in memory, we can just store shadow mappings directly into the hardware TLB, accepting that the host kernel will clobber these as part of the normal 440 TLB round robin. When we do that we need less than half the memory, and we have decreased the exit handling time for all guest exits, at the cost of increased number of TLB misses because the host overwrites some guest entries. These savings will be increased on processors with larger TLBs or which implement intelligent flush instructions like tlbivax (which will avoid the need to walk arrays in software). In addition to that and to the code simplification, we have a greater chance of leaving other host userspace mappings in the TLB, instead of forcing all subsequent tasks to re-fault all their mappings. Signed-off-by: Hollis Blanchard <hollisb@us.ibm.com> Signed-off-by: Avi Kivity <avi@redhat.com>
95 lines
3.9 KiB
C
95 lines
3.9 KiB
C
/*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License, version 2, as
|
|
* published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
*
|
|
* Copyright IBM Corp. 2008
|
|
*
|
|
* Authors: Hollis Blanchard <hollisb@us.ibm.com>
|
|
*/
|
|
|
|
#ifndef __POWERPC_KVM_PPC_H__
|
|
#define __POWERPC_KVM_PPC_H__
|
|
|
|
/* This file exists just so we can dereference kvm_vcpu, avoiding nested header
|
|
* dependencies. */
|
|
|
|
#include <linux/mutex.h>
|
|
#include <linux/timer.h>
|
|
#include <linux/types.h>
|
|
#include <linux/kvm_types.h>
|
|
#include <linux/kvm_host.h>
|
|
|
|
enum emulation_result {
|
|
EMULATE_DONE, /* no further processing */
|
|
EMULATE_DO_MMIO, /* kvm_run filled with MMIO request */
|
|
EMULATE_DO_DCR, /* kvm_run filled with DCR request */
|
|
EMULATE_FAIL, /* can't emulate this instruction */
|
|
};
|
|
|
|
extern int __kvmppc_vcpu_run(struct kvm_run *kvm_run, struct kvm_vcpu *vcpu);
|
|
extern char kvmppc_handlers_start[];
|
|
extern unsigned long kvmppc_handler_len;
|
|
|
|
extern void kvmppc_dump_vcpu(struct kvm_vcpu *vcpu);
|
|
extern int kvmppc_handle_load(struct kvm_run *run, struct kvm_vcpu *vcpu,
|
|
unsigned int rt, unsigned int bytes,
|
|
int is_bigendian);
|
|
extern int kvmppc_handle_store(struct kvm_run *run, struct kvm_vcpu *vcpu,
|
|
u32 val, unsigned int bytes, int is_bigendian);
|
|
|
|
extern int kvmppc_emulate_instruction(struct kvm_run *run,
|
|
struct kvm_vcpu *vcpu);
|
|
extern int kvmppc_emulate_mmio(struct kvm_run *run, struct kvm_vcpu *vcpu);
|
|
extern void kvmppc_emulate_dec(struct kvm_vcpu *vcpu);
|
|
|
|
extern void kvmppc_mmu_map(struct kvm_vcpu *vcpu, u64 gvaddr, gpa_t gpaddr,
|
|
u64 asid, u32 flags, u32 max_bytes,
|
|
unsigned int gtlb_idx);
|
|
extern void kvmppc_mmu_priv_switch(struct kvm_vcpu *vcpu, int usermode);
|
|
extern void kvmppc_mmu_switch_pid(struct kvm_vcpu *vcpu, u32 pid);
|
|
|
|
/* Core-specific hooks */
|
|
|
|
extern struct kvm_vcpu *kvmppc_core_vcpu_create(struct kvm *kvm,
|
|
unsigned int id);
|
|
extern void kvmppc_core_vcpu_free(struct kvm_vcpu *vcpu);
|
|
extern int kvmppc_core_vcpu_setup(struct kvm_vcpu *vcpu);
|
|
extern int kvmppc_core_check_processor_compat(void);
|
|
extern int kvmppc_core_vcpu_translate(struct kvm_vcpu *vcpu,
|
|
struct kvm_translation *tr);
|
|
|
|
extern void kvmppc_core_vcpu_load(struct kvm_vcpu *vcpu, int cpu);
|
|
extern void kvmppc_core_vcpu_put(struct kvm_vcpu *vcpu);
|
|
|
|
extern void kvmppc_core_load_guest_debugstate(struct kvm_vcpu *vcpu);
|
|
extern void kvmppc_core_load_host_debugstate(struct kvm_vcpu *vcpu);
|
|
|
|
extern void kvmppc_core_deliver_interrupts(struct kvm_vcpu *vcpu);
|
|
extern int kvmppc_core_pending_dec(struct kvm_vcpu *vcpu);
|
|
extern void kvmppc_core_queue_program(struct kvm_vcpu *vcpu);
|
|
extern void kvmppc_core_queue_dec(struct kvm_vcpu *vcpu);
|
|
extern void kvmppc_core_queue_external(struct kvm_vcpu *vcpu,
|
|
struct kvm_interrupt *irq);
|
|
|
|
extern int kvmppc_core_emulate_op(struct kvm_run *run, struct kvm_vcpu *vcpu,
|
|
unsigned int op, int *advance);
|
|
extern int kvmppc_core_emulate_mtspr(struct kvm_vcpu *vcpu, int sprn, int rs);
|
|
extern int kvmppc_core_emulate_mfspr(struct kvm_vcpu *vcpu, int sprn, int rt);
|
|
|
|
extern int kvmppc_booke_init(void);
|
|
extern void kvmppc_booke_exit(void);
|
|
|
|
extern void kvmppc_core_destroy_mmu(struct kvm_vcpu *vcpu);
|
|
|
|
#endif /* __POWERPC_KVM_PPC_H__ */
|