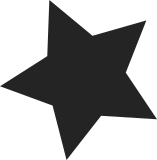
Redefine GZIP, BZIP2, LZOP variables as KGZIP, KBZIP2, KLZOP resp.
GZIP, BZIP2, LZOP env variables are reserved by the tools. The original
attempt to redefine them internally doesn't work in makefiles/scripts
intercall scenarios, e.g., "make GZIP=gzip bindeb-pkg" and results in
broken builds. There can be other broken build commands because of this,
so the universal solution is to use non-reserved env variables for the
compression tools.
Fixes: 8dfb61dcba
("kbuild: add variables for compression tools")
Signed-off-by: Denis Efremov <efremov@linux.com>
Tested-by: Guenter Roeck <linux@roeck-us.net>
Signed-off-by: Masahiro Yamada <masahiroy@kernel.org>
145 lines
4.2 KiB
Bash
Executable file
145 lines
4.2 KiB
Bash
Executable file
#!/bin/sh
|
|
# SPDX-License-Identifier: GPL-2.0
|
|
|
|
#
|
|
# buildtar 0.0.5
|
|
#
|
|
# (C) 2004-2006 by Jan-Benedict Glaw <jbglaw@lug-owl.de>
|
|
#
|
|
# This script is used to compile a tarball from the currently
|
|
# prepared kernel. Based upon the builddeb script from
|
|
# Wichert Akkerman <wichert@wiggy.net>.
|
|
#
|
|
|
|
set -e
|
|
|
|
#
|
|
# Some variables and settings used throughout the script
|
|
#
|
|
tmpdir="${objtree}/tar-install"
|
|
tarball="${objtree}/linux-${KERNELRELEASE}-${ARCH}.tar"
|
|
|
|
|
|
#
|
|
# Figure out how to compress, if requested at all
|
|
#
|
|
case "${1}" in
|
|
dir-pkg|tar-pkg)
|
|
opts=
|
|
;;
|
|
targz-pkg)
|
|
opts="-I ${KGZIP}"
|
|
tarball=${tarball}.gz
|
|
;;
|
|
tarbz2-pkg)
|
|
opts="-I ${KBZIP2}"
|
|
tarball=${tarball}.bz2
|
|
;;
|
|
tarxz-pkg)
|
|
opts="-I ${XZ}"
|
|
tarball=${tarball}.xz
|
|
;;
|
|
*)
|
|
echo "Unknown tarball target \"${1}\" requested, please add it to ${0}." >&2
|
|
exit 1
|
|
;;
|
|
esac
|
|
|
|
|
|
#
|
|
# Clean-up and re-create the temporary directory
|
|
#
|
|
rm -rf -- "${tmpdir}"
|
|
mkdir -p -- "${tmpdir}/boot"
|
|
dirs=boot
|
|
|
|
#
|
|
# Try to install modules
|
|
#
|
|
if grep -q '^CONFIG_MODULES=y' include/config/auto.conf; then
|
|
make ARCH="${ARCH}" -f ${srctree}/Makefile INSTALL_MOD_PATH="${tmpdir}" modules_install
|
|
dirs="$dirs lib"
|
|
fi
|
|
|
|
|
|
#
|
|
# Install basic kernel files
|
|
#
|
|
cp -v -- "${objtree}/System.map" "${tmpdir}/boot/System.map-${KERNELRELEASE}"
|
|
cp -v -- "${KCONFIG_CONFIG}" "${tmpdir}/boot/config-${KERNELRELEASE}"
|
|
cp -v -- "${objtree}/vmlinux" "${tmpdir}/boot/vmlinux-${KERNELRELEASE}"
|
|
|
|
|
|
#
|
|
# Install arch-specific kernel image(s)
|
|
#
|
|
case "${ARCH}" in
|
|
x86|i386|x86_64)
|
|
[ -f "${objtree}/arch/x86/boot/bzImage" ] && cp -v -- "${objtree}/arch/x86/boot/bzImage" "${tmpdir}/boot/vmlinuz-${KERNELRELEASE}"
|
|
;;
|
|
alpha)
|
|
[ -f "${objtree}/arch/alpha/boot/vmlinux.gz" ] && cp -v -- "${objtree}/arch/alpha/boot/vmlinux.gz" "${tmpdir}/boot/vmlinuz-${KERNELRELEASE}"
|
|
;;
|
|
parisc*)
|
|
[ -f "${KBUILD_IMAGE}" ] && cp -v -- "${KBUILD_IMAGE}" "${tmpdir}/boot/vmlinux-${KERNELRELEASE}"
|
|
[ -f "${objtree}/lifimage" ] && cp -v -- "${objtree}/lifimage" "${tmpdir}/boot/lifimage-${KERNELRELEASE}"
|
|
;;
|
|
mips)
|
|
if [ -f "${objtree}/arch/mips/boot/compressed/vmlinux.bin" ]; then
|
|
cp -v -- "${objtree}/arch/mips/boot/compressed/vmlinux.bin" "${tmpdir}/boot/vmlinuz-${KERNELRELEASE}"
|
|
elif [ -f "${objtree}/arch/mips/boot/compressed/vmlinux.ecoff" ]; then
|
|
cp -v -- "${objtree}/arch/mips/boot/compressed/vmlinux.ecoff" "${tmpdir}/boot/vmlinuz-${KERNELRELEASE}"
|
|
elif [ -f "${objtree}/arch/mips/boot/compressed/vmlinux.srec" ]; then
|
|
cp -v -- "${objtree}/arch/mips/boot/compressed/vmlinux.srec" "${tmpdir}/boot/vmlinuz-${KERNELRELEASE}"
|
|
elif [ -f "${objtree}/vmlinux.32" ]; then
|
|
cp -v -- "${objtree}/vmlinux.32" "${tmpdir}/boot/vmlinux-${KERNELRELEASE}"
|
|
elif [ -f "${objtree}/vmlinux.64" ]; then
|
|
cp -v -- "${objtree}/vmlinux.64" "${tmpdir}/boot/vmlinux-${KERNELRELEASE}"
|
|
elif [ -f "${objtree}/arch/mips/boot/vmlinux.bin" ]; then
|
|
cp -v -- "${objtree}/arch/mips/boot/vmlinux.bin" "${tmpdir}/boot/vmlinux-${KERNELRELEASE}"
|
|
elif [ -f "${objtree}/arch/mips/boot/vmlinux.ecoff" ]; then
|
|
cp -v -- "${objtree}/arch/mips/boot/vmlinux.ecoff" "${tmpdir}/boot/vmlinux-${KERNELRELEASE}"
|
|
elif [ -f "${objtree}/arch/mips/boot/vmlinux.srec" ]; then
|
|
cp -v -- "${objtree}/arch/mips/boot/vmlinux.srec" "${tmpdir}/boot/vmlinux-${KERNELRELEASE}"
|
|
elif [ -f "${objtree}/vmlinux" ]; then
|
|
cp -v -- "${objtree}/vmlinux" "${tmpdir}/boot/vmlinux-${KERNELRELEASE}"
|
|
fi
|
|
;;
|
|
arm64)
|
|
for i in Image.bz2 Image.gz Image.lz4 Image.lzma Image.lzo ; do
|
|
if [ -f "${objtree}/arch/arm64/boot/${i}" ] ; then
|
|
cp -v -- "${objtree}/arch/arm64/boot/${i}" "${tmpdir}/boot/vmlinuz-${KERNELRELEASE}"
|
|
break
|
|
fi
|
|
done
|
|
;;
|
|
*)
|
|
[ -f "${KBUILD_IMAGE}" ] && cp -v -- "${KBUILD_IMAGE}" "${tmpdir}/boot/vmlinux-kbuild-${KERNELRELEASE}"
|
|
echo "" >&2
|
|
echo '** ** ** WARNING ** ** **' >&2
|
|
echo "" >&2
|
|
echo "Your architecture did not define any architecture-dependent files" >&2
|
|
echo "to be placed into the tarball. Please add those to ${0} ..." >&2
|
|
echo "" >&2
|
|
sleep 5
|
|
;;
|
|
esac
|
|
|
|
if [ "${1}" = dir-pkg ]; then
|
|
echo "Kernel tree successfully created in $tmpdir"
|
|
exit 0
|
|
fi
|
|
|
|
#
|
|
# Create the tarball
|
|
#
|
|
if tar --owner=root --group=root --help >/dev/null 2>&1; then
|
|
opts="$opts --owner=root --group=root"
|
|
fi
|
|
|
|
tar cf $tarball -C $tmpdir $opts $dirs
|
|
|
|
echo "Tarball successfully created in $tarball"
|
|
|
|
exit 0
|