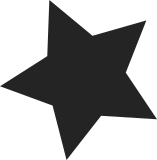
BFS is a very simple FS and its superblocks contains only static information and is never changed. However, the BFS code for some misterious reasons marked its buffer head as dirty from time to time, but nothing in that buffer was ever changed. This patch removes all the BFS superblock manipulation, simply because it is not needed. It removes: 1. The si_sbh filed from 'struct bfs_sb_info' because it is not needed. We only need to read the SB once on mount to get the start of data blocks and the FS size. After this, we can forget about the SB. 2. All instances of 'mark_buffer_dirty(sbh)' for BFS SB because it is never changed. 3. The '->sync_fs()' method because there is nothing to sync (inodes are synched by VFS). 4. The '->write_super()' method, again, because the SB is never changed. Tested-by: Artem Bityutskiy <Artem.Bityutskiy@nokia.com> Signed-off-by: Artem Bityutskiy <Artem.Bityutskiy@nokia.com> Signed-off-by: Al Viro <viro@zeniv.linux.org.uk>
60 lines
1.3 KiB
C
60 lines
1.3 KiB
C
/*
|
|
* fs/bfs/bfs.h
|
|
* Copyright (C) 1999 Tigran Aivazian <tigran@veritas.com>
|
|
*/
|
|
#ifndef _FS_BFS_BFS_H
|
|
#define _FS_BFS_BFS_H
|
|
|
|
#include <linux/bfs_fs.h>
|
|
|
|
/*
|
|
* BFS file system in-core superblock info
|
|
*/
|
|
struct bfs_sb_info {
|
|
unsigned long si_blocks;
|
|
unsigned long si_freeb;
|
|
unsigned long si_freei;
|
|
unsigned long si_lf_eblk;
|
|
unsigned long si_lasti;
|
|
unsigned long *si_imap;
|
|
struct mutex bfs_lock;
|
|
};
|
|
|
|
/*
|
|
* BFS file system in-core inode info
|
|
*/
|
|
struct bfs_inode_info {
|
|
unsigned long i_dsk_ino; /* inode number from the disk, can be 0 */
|
|
unsigned long i_sblock;
|
|
unsigned long i_eblock;
|
|
struct inode vfs_inode;
|
|
};
|
|
|
|
static inline struct bfs_sb_info *BFS_SB(struct super_block *sb)
|
|
{
|
|
return sb->s_fs_info;
|
|
}
|
|
|
|
static inline struct bfs_inode_info *BFS_I(struct inode *inode)
|
|
{
|
|
return container_of(inode, struct bfs_inode_info, vfs_inode);
|
|
}
|
|
|
|
|
|
#define printf(format, args...) \
|
|
printk(KERN_ERR "BFS-fs: %s(): " format, __func__, ## args)
|
|
|
|
/* inode.c */
|
|
extern struct inode *bfs_iget(struct super_block *sb, unsigned long ino);
|
|
|
|
/* file.c */
|
|
extern const struct inode_operations bfs_file_inops;
|
|
extern const struct file_operations bfs_file_operations;
|
|
extern const struct address_space_operations bfs_aops;
|
|
|
|
/* dir.c */
|
|
extern const struct inode_operations bfs_dir_inops;
|
|
extern const struct file_operations bfs_dir_operations;
|
|
|
|
#endif /* _FS_BFS_BFS_H */
|