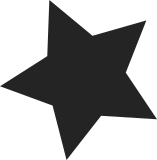
The FIFO_DEPTH hardware configuration parameter can be found from the power-on value of RX_WMark in the FIFOTH register. This is used to initialise the watermarks, but when calculating the number of free fifo spaces a preprocessor definition is used which is hard coded to 32. Fix reading the value out of FIFOTH (the default value in the RX_WMark field is FIFO_DEPTH-1 not FIFO_DEPTH). Allow the fifo depth to be overriden by platform data (since a bootloader may have changed FIFOTH making auto-detection unreliable). Store the fifo_depth for later use. Also fix the calculation to find the number of free bytes in the fifo to include the fifo depth in the left shift by the data shift, since the fifo depth is measured in fifo items not bytes. Signed-off-by: James Hogan <james.hogan@imgtec.com> Acked-by: Will Newton <will.newton@imgtec.com> Signed-off-by: Chris Ball <cjb@laptop.org>
168 lines
5.2 KiB
C
168 lines
5.2 KiB
C
/*
|
|
* Synopsys DesignWare Multimedia Card Interface driver
|
|
* (Based on NXP driver for lpc 31xx)
|
|
*
|
|
* Copyright (C) 2009 NXP Semiconductors
|
|
* Copyright (C) 2009, 2010 Imagination Technologies Ltd.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*/
|
|
|
|
#ifndef _DW_MMC_H_
|
|
#define _DW_MMC_H_
|
|
|
|
#define SDMMC_CTRL 0x000
|
|
#define SDMMC_PWREN 0x004
|
|
#define SDMMC_CLKDIV 0x008
|
|
#define SDMMC_CLKSRC 0x00c
|
|
#define SDMMC_CLKENA 0x010
|
|
#define SDMMC_TMOUT 0x014
|
|
#define SDMMC_CTYPE 0x018
|
|
#define SDMMC_BLKSIZ 0x01c
|
|
#define SDMMC_BYTCNT 0x020
|
|
#define SDMMC_INTMASK 0x024
|
|
#define SDMMC_CMDARG 0x028
|
|
#define SDMMC_CMD 0x02c
|
|
#define SDMMC_RESP0 0x030
|
|
#define SDMMC_RESP1 0x034
|
|
#define SDMMC_RESP2 0x038
|
|
#define SDMMC_RESP3 0x03c
|
|
#define SDMMC_MINTSTS 0x040
|
|
#define SDMMC_RINTSTS 0x044
|
|
#define SDMMC_STATUS 0x048
|
|
#define SDMMC_FIFOTH 0x04c
|
|
#define SDMMC_CDETECT 0x050
|
|
#define SDMMC_WRTPRT 0x054
|
|
#define SDMMC_GPIO 0x058
|
|
#define SDMMC_TCBCNT 0x05c
|
|
#define SDMMC_TBBCNT 0x060
|
|
#define SDMMC_DEBNCE 0x064
|
|
#define SDMMC_USRID 0x068
|
|
#define SDMMC_VERID 0x06c
|
|
#define SDMMC_HCON 0x070
|
|
#define SDMMC_UHS_REG 0x074
|
|
#define SDMMC_BMOD 0x080
|
|
#define SDMMC_PLDMND 0x084
|
|
#define SDMMC_DBADDR 0x088
|
|
#define SDMMC_IDSTS 0x08c
|
|
#define SDMMC_IDINTEN 0x090
|
|
#define SDMMC_DSCADDR 0x094
|
|
#define SDMMC_BUFADDR 0x098
|
|
#define SDMMC_DATA 0x100
|
|
|
|
/* shift bit field */
|
|
#define _SBF(f, v) ((v) << (f))
|
|
|
|
/* Control register defines */
|
|
#define SDMMC_CTRL_USE_IDMAC BIT(25)
|
|
#define SDMMC_CTRL_CEATA_INT_EN BIT(11)
|
|
#define SDMMC_CTRL_SEND_AS_CCSD BIT(10)
|
|
#define SDMMC_CTRL_SEND_CCSD BIT(9)
|
|
#define SDMMC_CTRL_ABRT_READ_DATA BIT(8)
|
|
#define SDMMC_CTRL_SEND_IRQ_RESP BIT(7)
|
|
#define SDMMC_CTRL_READ_WAIT BIT(6)
|
|
#define SDMMC_CTRL_DMA_ENABLE BIT(5)
|
|
#define SDMMC_CTRL_INT_ENABLE BIT(4)
|
|
#define SDMMC_CTRL_DMA_RESET BIT(2)
|
|
#define SDMMC_CTRL_FIFO_RESET BIT(1)
|
|
#define SDMMC_CTRL_RESET BIT(0)
|
|
/* Clock Enable register defines */
|
|
#define SDMMC_CLKEN_LOW_PWR BIT(16)
|
|
#define SDMMC_CLKEN_ENABLE BIT(0)
|
|
/* time-out register defines */
|
|
#define SDMMC_TMOUT_DATA(n) _SBF(8, (n))
|
|
#define SDMMC_TMOUT_DATA_MSK 0xFFFFFF00
|
|
#define SDMMC_TMOUT_RESP(n) ((n) & 0xFF)
|
|
#define SDMMC_TMOUT_RESP_MSK 0xFF
|
|
/* card-type register defines */
|
|
#define SDMMC_CTYPE_8BIT BIT(16)
|
|
#define SDMMC_CTYPE_4BIT BIT(0)
|
|
#define SDMMC_CTYPE_1BIT 0
|
|
/* Interrupt status & mask register defines */
|
|
#define SDMMC_INT_SDIO BIT(16)
|
|
#define SDMMC_INT_EBE BIT(15)
|
|
#define SDMMC_INT_ACD BIT(14)
|
|
#define SDMMC_INT_SBE BIT(13)
|
|
#define SDMMC_INT_HLE BIT(12)
|
|
#define SDMMC_INT_FRUN BIT(11)
|
|
#define SDMMC_INT_HTO BIT(10)
|
|
#define SDMMC_INT_DTO BIT(9)
|
|
#define SDMMC_INT_RTO BIT(8)
|
|
#define SDMMC_INT_DCRC BIT(7)
|
|
#define SDMMC_INT_RCRC BIT(6)
|
|
#define SDMMC_INT_RXDR BIT(5)
|
|
#define SDMMC_INT_TXDR BIT(4)
|
|
#define SDMMC_INT_DATA_OVER BIT(3)
|
|
#define SDMMC_INT_CMD_DONE BIT(2)
|
|
#define SDMMC_INT_RESP_ERR BIT(1)
|
|
#define SDMMC_INT_CD BIT(0)
|
|
#define SDMMC_INT_ERROR 0xbfc2
|
|
/* Command register defines */
|
|
#define SDMMC_CMD_START BIT(31)
|
|
#define SDMMC_CMD_CCS_EXP BIT(23)
|
|
#define SDMMC_CMD_CEATA_RD BIT(22)
|
|
#define SDMMC_CMD_UPD_CLK BIT(21)
|
|
#define SDMMC_CMD_INIT BIT(15)
|
|
#define SDMMC_CMD_STOP BIT(14)
|
|
#define SDMMC_CMD_PRV_DAT_WAIT BIT(13)
|
|
#define SDMMC_CMD_SEND_STOP BIT(12)
|
|
#define SDMMC_CMD_STRM_MODE BIT(11)
|
|
#define SDMMC_CMD_DAT_WR BIT(10)
|
|
#define SDMMC_CMD_DAT_EXP BIT(9)
|
|
#define SDMMC_CMD_RESP_CRC BIT(8)
|
|
#define SDMMC_CMD_RESP_LONG BIT(7)
|
|
#define SDMMC_CMD_RESP_EXP BIT(6)
|
|
#define SDMMC_CMD_INDX(n) ((n) & 0x1F)
|
|
/* Status register defines */
|
|
#define SDMMC_GET_FCNT(x) (((x)>>17) & 0x1FF)
|
|
/* Internal DMAC interrupt defines */
|
|
#define SDMMC_IDMAC_INT_AI BIT(9)
|
|
#define SDMMC_IDMAC_INT_NI BIT(8)
|
|
#define SDMMC_IDMAC_INT_CES BIT(5)
|
|
#define SDMMC_IDMAC_INT_DU BIT(4)
|
|
#define SDMMC_IDMAC_INT_FBE BIT(2)
|
|
#define SDMMC_IDMAC_INT_RI BIT(1)
|
|
#define SDMMC_IDMAC_INT_TI BIT(0)
|
|
/* Internal DMAC bus mode bits */
|
|
#define SDMMC_IDMAC_ENABLE BIT(7)
|
|
#define SDMMC_IDMAC_FB BIT(1)
|
|
#define SDMMC_IDMAC_SWRESET BIT(0)
|
|
|
|
/* Register access macros */
|
|
#define mci_readl(dev, reg) \
|
|
__raw_readl((dev)->regs + SDMMC_##reg)
|
|
#define mci_writel(dev, reg, value) \
|
|
__raw_writel((value), (dev)->regs + SDMMC_##reg)
|
|
|
|
/* 16-bit FIFO access macros */
|
|
#define mci_readw(dev, reg) \
|
|
__raw_readw((dev)->regs + SDMMC_##reg)
|
|
#define mci_writew(dev, reg, value) \
|
|
__raw_writew((value), (dev)->regs + SDMMC_##reg)
|
|
|
|
/* 64-bit FIFO access macros */
|
|
#ifdef readq
|
|
#define mci_readq(dev, reg) \
|
|
__raw_readq((dev)->regs + SDMMC_##reg)
|
|
#define mci_writeq(dev, reg, value) \
|
|
__raw_writeq((value), (dev)->regs + SDMMC_##reg)
|
|
#else
|
|
/*
|
|
* Dummy readq implementation for architectures that don't define it.
|
|
*
|
|
* We would assume that none of these architectures would configure
|
|
* the IP block with a 64bit FIFO width, so this code will never be
|
|
* executed on those machines. Defining these macros here keeps the
|
|
* rest of the code free from ifdefs.
|
|
*/
|
|
#define mci_readq(dev, reg) \
|
|
(*(volatile u64 __force *)((dev)->regs + SDMMC_##reg))
|
|
#define mci_writeq(dev, reg, value) \
|
|
(*(volatile u64 __force *)((dev)->regs + SDMMC_##reg) = (value))
|
|
#endif
|
|
|
|
#endif /* _DW_MMC_H_ */
|