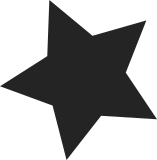
The introduction of these dummy BUILD_BUG_ON stubs dates back to commmit
903c0c7cdc
("sparse: define dummy BUILD_BUG_ON definition for
sparse").
At that time, BUILD_BUG_ON() was implemented with the negative array
trick *and* the link-time trick, like this:
extern int __build_bug_on_failed;
#define BUILD_BUG_ON(condition) \
do { \
((void)sizeof(char[1 - 2*!!(condition)])); \
if (condition) __build_bug_on_failed = 1; \
} while(0)
Sparse is more strict about the negative array trick than GCC because
Sparse requires the array length to be really constant.
Here is the simple test code for the macro above:
static const int x = 0;
BUILD_BUG_ON(x);
GCC is absolutely fine with it (-Wvla was enabled only very recently),
but Sparse warns like this:
error: bad constant expression
error: cannot size expression
(If you are using a newer version of Sparse, you will see a different
warning message, "warning: Variable length array is used".)
Anyway, Sparse was producing many false positives, and noisier than it
should be at that time.
With the previous commit, the leftover negative array trick is gone.
Sparse is fine with the current BUILD_BUG_ON(), which is implemented by
using the 'error' attribute.
I am keeping the stub for BUILD_BUG_ON_ZERO(). Otherwise, Sparse would
complain about the following code, which GCC is fine with:
static const int x = 0;
int y = BUILD_BUG_ON_ZERO(x);
Link: http://lkml.kernel.org/r/1542856462-18836-3-git-send-email-yamada.masahiro@socionext.com
Signed-off-by: Masahiro Yamada <yamada.masahiro@socionext.com>
Acked-by: Kees Cook <keescook@chromium.org>
Reviewed-by: Luc Van Oostenryck <luc.vanoostenryck@gmail.com>
Reviewed-by: Nick Desaulniers <ndesaulniers@google.com>
Tested-by: Nick Desaulniers <ndesaulniers@google.com>
Signed-off-by: Andrew Morton <akpm@linux-foundation.org>
Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
62 lines
2 KiB
C
62 lines
2 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _LINUX_BUILD_BUG_H
|
|
#define _LINUX_BUILD_BUG_H
|
|
|
|
#include <linux/compiler.h>
|
|
|
|
#ifdef __CHECKER__
|
|
#define BUILD_BUG_ON_ZERO(e) (0)
|
|
#else /* __CHECKER__ */
|
|
/*
|
|
* Force a compilation error if condition is true, but also produce a
|
|
* result (of value 0 and type size_t), so the expression can be used
|
|
* e.g. in a structure initializer (or where-ever else comma expressions
|
|
* aren't permitted).
|
|
*/
|
|
#define BUILD_BUG_ON_ZERO(e) (sizeof(struct { int:(-!!(e)); }))
|
|
#endif /* __CHECKER__ */
|
|
|
|
/* Force a compilation error if a constant expression is not a power of 2 */
|
|
#define __BUILD_BUG_ON_NOT_POWER_OF_2(n) \
|
|
BUILD_BUG_ON(((n) & ((n) - 1)) != 0)
|
|
#define BUILD_BUG_ON_NOT_POWER_OF_2(n) \
|
|
BUILD_BUG_ON((n) == 0 || (((n) & ((n) - 1)) != 0))
|
|
|
|
/*
|
|
* BUILD_BUG_ON_INVALID() permits the compiler to check the validity of the
|
|
* expression but avoids the generation of any code, even if that expression
|
|
* has side-effects.
|
|
*/
|
|
#define BUILD_BUG_ON_INVALID(e) ((void)(sizeof((__force long)(e))))
|
|
|
|
/**
|
|
* BUILD_BUG_ON_MSG - break compile if a condition is true & emit supplied
|
|
* error message.
|
|
* @condition: the condition which the compiler should know is false.
|
|
*
|
|
* See BUILD_BUG_ON for description.
|
|
*/
|
|
#define BUILD_BUG_ON_MSG(cond, msg) compiletime_assert(!(cond), msg)
|
|
|
|
/**
|
|
* BUILD_BUG_ON - break compile if a condition is true.
|
|
* @condition: the condition which the compiler should know is false.
|
|
*
|
|
* If you have some code which relies on certain constants being equal, or
|
|
* some other compile-time-evaluated condition, you should use BUILD_BUG_ON to
|
|
* detect if someone changes it.
|
|
*/
|
|
#define BUILD_BUG_ON(condition) \
|
|
BUILD_BUG_ON_MSG(condition, "BUILD_BUG_ON failed: " #condition)
|
|
|
|
/**
|
|
* BUILD_BUG - break compile if used.
|
|
*
|
|
* If you have some code that you expect the compiler to eliminate at
|
|
* build time, you should use BUILD_BUG to detect if it is
|
|
* unexpectedly used.
|
|
*/
|
|
#define BUILD_BUG() BUILD_BUG_ON_MSG(1, "BUILD_BUG failed")
|
|
|
|
#endif /* _LINUX_BUILD_BUG_H */
|