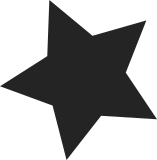
On 32bit platforms, time_t is still a signed 32bit long. If it is overflowed, userspace and the kernel cant agree on the current system time. This causes multiple issues, in particular with systemd: https://github.com/systemd/systemd/issues/1143 A good workaround is to simply avoid using hctosys which is something I greatly encourage as the time is better set by userspace. However, many distribution enable it and use systemd which is rendering the system unusable in case the RTC holds a date after 2038 (and more so after 2106). Many drivers have workaround for this case and they should be eliminated so there is only one place left to fix when userspace is able to cope with dates after the 31bit overflow. Acked-by: Arnd Bergmann <arnd@arndb.de> Signed-off-by: Alexandre Belloni <alexandre.belloni@bootlin.com>
76 lines
1.9 KiB
C
76 lines
1.9 KiB
C
/*
|
|
* RTC subsystem, initialize system time on startup
|
|
*
|
|
* Copyright (C) 2005 Tower Technologies
|
|
* Author: Alessandro Zummo <a.zummo@towertech.it>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*/
|
|
|
|
#define pr_fmt(fmt) KBUILD_MODNAME ": " fmt
|
|
|
|
#include <linux/rtc.h>
|
|
|
|
/* IMPORTANT: the RTC only stores whole seconds. It is arbitrary
|
|
* whether it stores the most close value or the value with partial
|
|
* seconds truncated. However, it is important that we use it to store
|
|
* the truncated value. This is because otherwise it is necessary,
|
|
* in an rtc sync function, to read both xtime.tv_sec and
|
|
* xtime.tv_nsec. On some processors (i.e. ARM), an atomic read
|
|
* of >32bits is not possible. So storing the most close value would
|
|
* slow down the sync API. So here we have the truncated value and
|
|
* the best guess is to add 0.5s.
|
|
*/
|
|
|
|
static int __init rtc_hctosys(void)
|
|
{
|
|
int err = -ENODEV;
|
|
struct rtc_time tm;
|
|
struct timespec64 tv64 = {
|
|
.tv_nsec = NSEC_PER_SEC >> 1,
|
|
};
|
|
struct rtc_device *rtc = rtc_class_open(CONFIG_RTC_HCTOSYS_DEVICE);
|
|
|
|
if (rtc == NULL) {
|
|
pr_info("unable to open rtc device (%s)\n",
|
|
CONFIG_RTC_HCTOSYS_DEVICE);
|
|
goto err_open;
|
|
}
|
|
|
|
err = rtc_read_time(rtc, &tm);
|
|
if (err) {
|
|
dev_err(rtc->dev.parent,
|
|
"hctosys: unable to read the hardware clock\n");
|
|
goto err_read;
|
|
|
|
}
|
|
|
|
tv64.tv_sec = rtc_tm_to_time64(&tm);
|
|
|
|
#if BITS_PER_LONG == 32
|
|
if (tv64.tv_sec > INT_MAX)
|
|
goto err_read;
|
|
#endif
|
|
|
|
err = do_settimeofday64(&tv64);
|
|
|
|
dev_info(rtc->dev.parent,
|
|
"setting system clock to "
|
|
"%d-%02d-%02d %02d:%02d:%02d UTC (%lld)\n",
|
|
tm.tm_year + 1900, tm.tm_mon + 1, tm.tm_mday,
|
|
tm.tm_hour, tm.tm_min, tm.tm_sec,
|
|
(long long) tv64.tv_sec);
|
|
|
|
err_read:
|
|
rtc_class_close(rtc);
|
|
|
|
err_open:
|
|
rtc_hctosys_ret = err;
|
|
|
|
return err;
|
|
}
|
|
|
|
late_initcall(rtc_hctosys);
|