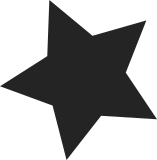
The code that initializes cpus on arm64 is currently split in two different code paths that carry out DT and ACPI cpus initialization. Most of the code executing SMP initialization is common and should be merged to reduce discrepancies between ACPI and DT initialization and to have code initializing cpus in a single common place in the kernel. This patch refactors arm64 SMP cpus initialization code to merge ACPI and DT boot paths in a common file and to create sanity checks that can be reused by both boot methods. Current code assumes PSCI is the only available boot method when arm64 boots with ACPI; this can be easily extended if/when the ACPI parking protocol is merged into the kernel. Signed-off-by: Sudeep Holla <sudeep.holla@arm.com> Signed-off-by: Lorenzo Pieralisi <lorenzo.pieralisi@arm.com> Acked-by: Hanjun Guo <hanjun.guo@linaro.org> Acked-by: Mark Rutland <mark.rutland@arm.com> Tested-by: Hanjun Guo <hanjun.guo@linaro.org> Tested-by: Mark Rutland <mark.rutland@arm.com> [DT] Cc: Will Deacon <will.deacon@arm.com> Signed-off-by: Catalin Marinas <catalin.marinas@arm.com>
75 lines
2.7 KiB
C
75 lines
2.7 KiB
C
/*
|
|
* Copyright (C) 2013 ARM Ltd.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
#ifndef __ASM_CPU_OPS_H
|
|
#define __ASM_CPU_OPS_H
|
|
|
|
#include <linux/init.h>
|
|
#include <linux/threads.h>
|
|
|
|
/**
|
|
* struct cpu_operations - Callback operations for hotplugging CPUs.
|
|
*
|
|
* @name: Name of the property as appears in a devicetree cpu node's
|
|
* enable-method property. On systems booting with ACPI, @name
|
|
* identifies the struct cpu_operations entry corresponding to
|
|
* the boot protocol specified in the ACPI MADT table.
|
|
* @cpu_init: Reads any data necessary for a specific enable-method for a
|
|
* proposed logical id.
|
|
* @cpu_prepare: Early one-time preparation step for a cpu. If there is a
|
|
* mechanism for doing so, tests whether it is possible to boot
|
|
* the given CPU.
|
|
* @cpu_boot: Boots a cpu into the kernel.
|
|
* @cpu_postboot: Optionally, perform any post-boot cleanup or necesary
|
|
* synchronisation. Called from the cpu being booted.
|
|
* @cpu_disable: Prepares a cpu to die. May fail for some mechanism-specific
|
|
* reason, which will cause the hot unplug to be aborted. Called
|
|
* from the cpu to be killed.
|
|
* @cpu_die: Makes a cpu leave the kernel. Must not fail. Called from the
|
|
* cpu being killed.
|
|
* @cpu_kill: Ensures a cpu has left the kernel. Called from another cpu.
|
|
* @cpu_init_idle: Reads any data necessary to initialize CPU idle states for
|
|
* a proposed logical id.
|
|
* @cpu_suspend: Suspends a cpu and saves the required context. May fail owing
|
|
* to wrong parameters or error conditions. Called from the
|
|
* CPU being suspended. Must be called with IRQs disabled.
|
|
*/
|
|
struct cpu_operations {
|
|
const char *name;
|
|
int (*cpu_init)(unsigned int);
|
|
int (*cpu_prepare)(unsigned int);
|
|
int (*cpu_boot)(unsigned int);
|
|
void (*cpu_postboot)(void);
|
|
#ifdef CONFIG_HOTPLUG_CPU
|
|
int (*cpu_disable)(unsigned int cpu);
|
|
void (*cpu_die)(unsigned int cpu);
|
|
int (*cpu_kill)(unsigned int cpu);
|
|
#endif
|
|
#ifdef CONFIG_CPU_IDLE
|
|
int (*cpu_init_idle)(unsigned int);
|
|
int (*cpu_suspend)(unsigned long);
|
|
#endif
|
|
};
|
|
|
|
extern const struct cpu_operations *cpu_ops[NR_CPUS];
|
|
int __init cpu_read_ops(int cpu);
|
|
|
|
static inline void __init cpu_read_bootcpu_ops(void)
|
|
{
|
|
cpu_read_ops(0);
|
|
}
|
|
|
|
#endif /* ifndef __ASM_CPU_OPS_H */
|