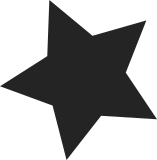
All the current architecture specific defines for these are the same. Refactor these common defines to a common header file. The new common linux/compat_time.h is also useful as it will eventually be used to hold all the defines that are needed for compat time types that support non y2038 safe types. New architectures need not have to define these new types as they will only use new y2038 safe syscalls. This file can be deleted after y2038 when we stop supporting non y2038 safe syscalls. The patch also requires an operation similar to: git grep "asm/compat\.h" | cut -d ":" -f 1 | xargs -n 1 sed -i -e "s%asm/compat.h%linux/compat.h%g" Cc: acme@kernel.org Cc: benh@kernel.crashing.org Cc: borntraeger@de.ibm.com Cc: catalin.marinas@arm.com Cc: cmetcalf@mellanox.com Cc: cohuck@redhat.com Cc: davem@davemloft.net Cc: deller@gmx.de Cc: devel@driverdev.osuosl.org Cc: gerald.schaefer@de.ibm.com Cc: gregkh@linuxfoundation.org Cc: heiko.carstens@de.ibm.com Cc: hoeppner@linux.vnet.ibm.com Cc: hpa@zytor.com Cc: jejb@parisc-linux.org Cc: jwi@linux.vnet.ibm.com Cc: linux-kernel@vger.kernel.org Cc: linux-mips@linux-mips.org Cc: linux-parisc@vger.kernel.org Cc: linuxppc-dev@lists.ozlabs.org Cc: linux-s390@vger.kernel.org Cc: mark.rutland@arm.com Cc: mingo@redhat.com Cc: mpe@ellerman.id.au Cc: oberpar@linux.vnet.ibm.com Cc: oprofile-list@lists.sf.net Cc: paulus@samba.org Cc: peterz@infradead.org Cc: ralf@linux-mips.org Cc: rostedt@goodmis.org Cc: rric@kernel.org Cc: schwidefsky@de.ibm.com Cc: sebott@linux.vnet.ibm.com Cc: sparclinux@vger.kernel.org Cc: sth@linux.vnet.ibm.com Cc: ubraun@linux.vnet.ibm.com Cc: will.deacon@arm.com Cc: x86@kernel.org Signed-off-by: Arnd Bergmann <arnd@arndb.de> Signed-off-by: Deepa Dinamani <deepa.kernel@gmail.com> Acked-by: Steven Rostedt (VMware) <rostedt@goodmis.org> Acked-by: Catalin Marinas <catalin.marinas@arm.com> Acked-by: James Hogan <jhogan@kernel.org> Acked-by: Helge Deller <deller@gmx.de> Signed-off-by: Arnd Bergmann <arnd@arndb.de>
63 lines
1.3 KiB
C
63 lines
1.3 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
#include <linux/compat.h>
|
|
#include <linux/errno.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/perf_event.h>
|
|
#include <linux/bug.h>
|
|
#include <linux/sched/task_stack.h>
|
|
|
|
#include <asm/perf_regs.h>
|
|
#include <asm/ptrace.h>
|
|
|
|
u64 perf_reg_value(struct pt_regs *regs, int idx)
|
|
{
|
|
if (WARN_ON_ONCE((u32)idx >= PERF_REG_ARM64_MAX))
|
|
return 0;
|
|
|
|
/*
|
|
* Compat (i.e. 32 bit) mode:
|
|
* - PC has been set in the pt_regs struct in kernel_entry,
|
|
* - Handle SP and LR here.
|
|
*/
|
|
if (compat_user_mode(regs)) {
|
|
if ((u32)idx == PERF_REG_ARM64_SP)
|
|
return regs->compat_sp;
|
|
if ((u32)idx == PERF_REG_ARM64_LR)
|
|
return regs->compat_lr;
|
|
}
|
|
|
|
if ((u32)idx == PERF_REG_ARM64_SP)
|
|
return regs->sp;
|
|
|
|
if ((u32)idx == PERF_REG_ARM64_PC)
|
|
return regs->pc;
|
|
|
|
return regs->regs[idx];
|
|
}
|
|
|
|
#define REG_RESERVED (~((1ULL << PERF_REG_ARM64_MAX) - 1))
|
|
|
|
int perf_reg_validate(u64 mask)
|
|
{
|
|
if (!mask || mask & REG_RESERVED)
|
|
return -EINVAL;
|
|
|
|
return 0;
|
|
}
|
|
|
|
u64 perf_reg_abi(struct task_struct *task)
|
|
{
|
|
if (is_compat_thread(task_thread_info(task)))
|
|
return PERF_SAMPLE_REGS_ABI_32;
|
|
else
|
|
return PERF_SAMPLE_REGS_ABI_64;
|
|
}
|
|
|
|
void perf_get_regs_user(struct perf_regs *regs_user,
|
|
struct pt_regs *regs,
|
|
struct pt_regs *regs_user_copy)
|
|
{
|
|
regs_user->regs = task_pt_regs(current);
|
|
regs_user->abi = perf_reg_abi(current);
|
|
}
|