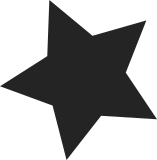
Especially for SDIO drivers which may have special conditions/errors to report, it is a good thing to relay the returned error code back to upper layers. This also allows for the rationalization of the resume path where code to "remove" a no-longer-existing or replaced card was duplicated into the MMC, SD and SDIO bus drivers. In the SDIO case, if a function suspend method returns an error, then all previously suspended functions are resumed and the error returned. An exception is made for -ENOSYS which the core interprets as "we don't support suspend so just kick the card out for suspend and return success". When resuming SDIO cards, the core code only validates the manufacturer and product IDs to make sure the same kind of card is still present before invoking functions resume methods. It's the function driver's responsibility to perform further tests to confirm that the actual same card is present (same MAC address, etc.) and return an error otherwise. Signed-off-by: Nicolas Pitre <nico@marvell.com> Cc: <linux-mmc@vger.kernel.org> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
68 lines
1.9 KiB
C
68 lines
1.9 KiB
C
/*
|
|
* linux/drivers/mmc/core/core.h
|
|
*
|
|
* Copyright (C) 2003 Russell King, All Rights Reserved.
|
|
* Copyright 2007 Pierre Ossman
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*/
|
|
#ifndef _MMC_CORE_CORE_H
|
|
#define _MMC_CORE_CORE_H
|
|
|
|
#include <linux/delay.h>
|
|
|
|
#define MMC_CMD_RETRIES 3
|
|
|
|
struct mmc_bus_ops {
|
|
int (*awake)(struct mmc_host *);
|
|
int (*sleep)(struct mmc_host *);
|
|
void (*remove)(struct mmc_host *);
|
|
void (*detect)(struct mmc_host *);
|
|
int (*suspend)(struct mmc_host *);
|
|
int (*resume)(struct mmc_host *);
|
|
void (*power_save)(struct mmc_host *);
|
|
void (*power_restore)(struct mmc_host *);
|
|
};
|
|
|
|
void mmc_attach_bus(struct mmc_host *host, const struct mmc_bus_ops *ops);
|
|
void mmc_detach_bus(struct mmc_host *host);
|
|
|
|
void mmc_set_chip_select(struct mmc_host *host, int mode);
|
|
void mmc_set_clock(struct mmc_host *host, unsigned int hz);
|
|
void mmc_set_bus_mode(struct mmc_host *host, unsigned int mode);
|
|
void mmc_set_bus_width(struct mmc_host *host, unsigned int width);
|
|
u32 mmc_select_voltage(struct mmc_host *host, u32 ocr);
|
|
void mmc_set_timing(struct mmc_host *host, unsigned int timing);
|
|
|
|
static inline void mmc_delay(unsigned int ms)
|
|
{
|
|
if (ms < 1000 / HZ) {
|
|
cond_resched();
|
|
mdelay(ms);
|
|
} else {
|
|
msleep(ms);
|
|
}
|
|
}
|
|
|
|
void mmc_rescan(struct work_struct *work);
|
|
void mmc_start_host(struct mmc_host *host);
|
|
void mmc_stop_host(struct mmc_host *host);
|
|
|
|
int mmc_attach_mmc(struct mmc_host *host, u32 ocr);
|
|
int mmc_attach_sd(struct mmc_host *host, u32 ocr);
|
|
int mmc_attach_sdio(struct mmc_host *host, u32 ocr);
|
|
|
|
extern int use_spi_crc;
|
|
|
|
/* Debugfs information for hosts and cards */
|
|
void mmc_add_host_debugfs(struct mmc_host *host);
|
|
void mmc_remove_host_debugfs(struct mmc_host *host);
|
|
|
|
void mmc_add_card_debugfs(struct mmc_card *card);
|
|
void mmc_remove_card_debugfs(struct mmc_card *card);
|
|
|
|
#endif
|
|
|