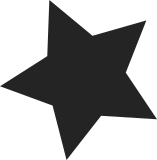
dev_queue_xmit() needs to dirty fields "state", "q", "bstats" and "qstats" On x86_64 arch, they currently span three cache lines, involving more cache line ping pongs than necessary, making longer holding of queue spinlock. We can reduce this to one cache line, by grouping all read-mostly fields at the beginning of structure. (Or should I say, all highly modified fields at the end :) ) Before patch : offsetof(struct Qdisc, state)=0x38 offsetof(struct Qdisc, q)=0x48 offsetof(struct Qdisc, bstats)=0x80 offsetof(struct Qdisc, qstats)=0x90 sizeof(struct Qdisc)=0xc8 After patch : offsetof(struct Qdisc, state)=0x80 offsetof(struct Qdisc, q)=0x88 offsetof(struct Qdisc, bstats)=0xa0 offsetof(struct Qdisc, qstats)=0xac sizeof(struct Qdisc)=0xc0 Signed-off-by: Eric Dumazet <dada1@cosmosbay.com> Signed-off-by: David S. Miller <davem@davemloft.net>
68 lines
1.2 KiB
C
68 lines
1.2 KiB
C
#ifndef __LINUX_GEN_STATS_H
|
|
#define __LINUX_GEN_STATS_H
|
|
|
|
#include <linux/types.h>
|
|
|
|
enum {
|
|
TCA_STATS_UNSPEC,
|
|
TCA_STATS_BASIC,
|
|
TCA_STATS_RATE_EST,
|
|
TCA_STATS_QUEUE,
|
|
TCA_STATS_APP,
|
|
__TCA_STATS_MAX,
|
|
};
|
|
#define TCA_STATS_MAX (__TCA_STATS_MAX - 1)
|
|
|
|
/**
|
|
* struct gnet_stats_basic - byte/packet throughput statistics
|
|
* @bytes: number of seen bytes
|
|
* @packets: number of seen packets
|
|
*/
|
|
struct gnet_stats_basic
|
|
{
|
|
__u64 bytes;
|
|
__u32 packets;
|
|
} __attribute__ ((packed));
|
|
|
|
/**
|
|
* struct gnet_stats_rate_est - rate estimator
|
|
* @bps: current byte rate
|
|
* @pps: current packet rate
|
|
*/
|
|
struct gnet_stats_rate_est
|
|
{
|
|
__u32 bps;
|
|
__u32 pps;
|
|
};
|
|
|
|
/**
|
|
* struct gnet_stats_queue - queuing statistics
|
|
* @qlen: queue length
|
|
* @backlog: backlog size of queue
|
|
* @drops: number of dropped packets
|
|
* @requeues: number of requeues
|
|
* @overlimits: number of enqueues over the limit
|
|
*/
|
|
struct gnet_stats_queue
|
|
{
|
|
__u32 qlen;
|
|
__u32 backlog;
|
|
__u32 drops;
|
|
__u32 requeues;
|
|
__u32 overlimits;
|
|
};
|
|
|
|
/**
|
|
* struct gnet_estimator - rate estimator configuration
|
|
* @interval: sampling period
|
|
* @ewma_log: the log of measurement window weight
|
|
*/
|
|
struct gnet_estimator
|
|
{
|
|
signed char interval;
|
|
unsigned char ewma_log;
|
|
};
|
|
|
|
|
|
#endif /* __LINUX_GEN_STATS_H */
|