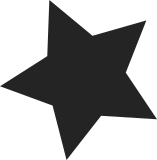
In commit 15ef17f622
(tty: ar933x_uart: use the clk API to get the uart
clock), the AR933x UART driver for has been converted
to get the uart clock rate via the clock API and it
does not use the platform data anymore.
Remove the ar933x_uart_platform.h header file and get
rid of the superfluous variable and initialization code
in platform setup.
Signed-off-by: Gabor Juhos <juhosg@openwrt.org>
Cc: linux-mips@linux-mips.org
Patchwork: https://patchwork.linux-mips.org/patch/5832/
Signed-off-by: Ralf Baechle <ralf@linux-mips.org>
109 lines
2.5 KiB
C
109 lines
2.5 KiB
C
/*
|
|
* Atheros AR71XX/AR724X/AR913X common devices
|
|
*
|
|
* Copyright (C) 2008-2011 Gabor Juhos <juhosg@openwrt.org>
|
|
* Copyright (C) 2008 Imre Kaloz <kaloz@openwrt.org>
|
|
*
|
|
* Parts of this file are based on Atheros' 2.6.15 BSP
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License version 2 as published
|
|
* by the Free Software Foundation.
|
|
*/
|
|
|
|
#include <linux/kernel.h>
|
|
#include <linux/init.h>
|
|
#include <linux/platform_device.h>
|
|
#include <linux/serial_8250.h>
|
|
#include <linux/clk.h>
|
|
#include <linux/err.h>
|
|
|
|
#include <asm/mach-ath79/ath79.h>
|
|
#include <asm/mach-ath79/ar71xx_regs.h>
|
|
#include "common.h"
|
|
#include "dev-common.h"
|
|
|
|
static struct resource ath79_uart_resources[] = {
|
|
{
|
|
.start = AR71XX_UART_BASE,
|
|
.end = AR71XX_UART_BASE + AR71XX_UART_SIZE - 1,
|
|
.flags = IORESOURCE_MEM,
|
|
},
|
|
};
|
|
|
|
#define AR71XX_UART_FLAGS (UPF_BOOT_AUTOCONF | UPF_SKIP_TEST | UPF_IOREMAP)
|
|
static struct plat_serial8250_port ath79_uart_data[] = {
|
|
{
|
|
.mapbase = AR71XX_UART_BASE,
|
|
.irq = ATH79_MISC_IRQ(3),
|
|
.flags = AR71XX_UART_FLAGS,
|
|
.iotype = UPIO_MEM32,
|
|
.regshift = 2,
|
|
}, {
|
|
/* terminating entry */
|
|
}
|
|
};
|
|
|
|
static struct platform_device ath79_uart_device = {
|
|
.name = "serial8250",
|
|
.id = PLAT8250_DEV_PLATFORM,
|
|
.resource = ath79_uart_resources,
|
|
.num_resources = ARRAY_SIZE(ath79_uart_resources),
|
|
.dev = {
|
|
.platform_data = ath79_uart_data
|
|
},
|
|
};
|
|
|
|
static struct resource ar933x_uart_resources[] = {
|
|
{
|
|
.start = AR933X_UART_BASE,
|
|
.end = AR933X_UART_BASE + AR71XX_UART_SIZE - 1,
|
|
.flags = IORESOURCE_MEM,
|
|
},
|
|
{
|
|
.start = ATH79_MISC_IRQ(3),
|
|
.end = ATH79_MISC_IRQ(3),
|
|
.flags = IORESOURCE_IRQ,
|
|
},
|
|
};
|
|
|
|
static struct platform_device ar933x_uart_device = {
|
|
.name = "ar933x-uart",
|
|
.id = -1,
|
|
.resource = ar933x_uart_resources,
|
|
.num_resources = ARRAY_SIZE(ar933x_uart_resources),
|
|
};
|
|
|
|
void __init ath79_register_uart(void)
|
|
{
|
|
unsigned long uart_clk_rate;
|
|
|
|
uart_clk_rate = ath79_get_sys_clk_rate("uart");
|
|
|
|
if (soc_is_ar71xx() ||
|
|
soc_is_ar724x() ||
|
|
soc_is_ar913x() ||
|
|
soc_is_ar934x() ||
|
|
soc_is_qca955x()) {
|
|
ath79_uart_data[0].uartclk = uart_clk_rate;
|
|
platform_device_register(&ath79_uart_device);
|
|
} else if (soc_is_ar933x()) {
|
|
platform_device_register(&ar933x_uart_device);
|
|
} else {
|
|
BUG();
|
|
}
|
|
}
|
|
|
|
void __init ath79_register_wdt(void)
|
|
{
|
|
struct resource res;
|
|
|
|
memset(&res, 0, sizeof(res));
|
|
|
|
res.flags = IORESOURCE_MEM;
|
|
res.start = AR71XX_RESET_BASE + AR71XX_RESET_REG_WDOG_CTRL;
|
|
res.end = res.start + 0x8 - 1;
|
|
|
|
platform_device_register_simple("ath79-wdt", -1, &res, 1);
|
|
}
|