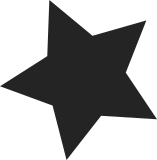
skb_gso_segment has three possible return values: 1. a pointer to the first segmented skb 2. an errno value (IS_ERR()) 3. NULL. This can happen when GSO is used for header verification. However, several callers currently test IS_ERR instead of IS_ERR_OR_NULL and would oops when NULL is returned. Note that these call sites should never actually see such a NULL return value; all callers mask out the GSO bits in the feature argument. However, there have been issues with some protocol handlers erronously not respecting the specified feature mask in some cases. It is preferable to get 'have to turn off hw offloading, else slow' reports rather than 'kernel crashes'. Signed-off-by: Florian Westphal <fw@strlen.de> Signed-off-by: David S. Miller <davem@davemloft.net>
237 lines
4.7 KiB
C
237 lines
4.7 KiB
C
/*
|
|
* xfrm_output.c - Common IPsec encapsulation code.
|
|
*
|
|
* Copyright (c) 2007 Herbert Xu <herbert@gondor.apana.org.au>
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version
|
|
* 2 of the License, or (at your option) any later version.
|
|
*/
|
|
|
|
#include <linux/errno.h>
|
|
#include <linux/module.h>
|
|
#include <linux/netdevice.h>
|
|
#include <linux/netfilter.h>
|
|
#include <linux/skbuff.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/spinlock.h>
|
|
#include <net/dst.h>
|
|
#include <net/xfrm.h>
|
|
|
|
static int xfrm_output2(struct sk_buff *skb);
|
|
|
|
static int xfrm_skb_check_space(struct sk_buff *skb)
|
|
{
|
|
struct dst_entry *dst = skb_dst(skb);
|
|
int nhead = dst->header_len + LL_RESERVED_SPACE(dst->dev)
|
|
- skb_headroom(skb);
|
|
int ntail = dst->dev->needed_tailroom - skb_tailroom(skb);
|
|
|
|
if (nhead <= 0) {
|
|
if (ntail <= 0)
|
|
return 0;
|
|
nhead = 0;
|
|
} else if (ntail < 0)
|
|
ntail = 0;
|
|
|
|
return pskb_expand_head(skb, nhead, ntail, GFP_ATOMIC);
|
|
}
|
|
|
|
static int xfrm_output_one(struct sk_buff *skb, int err)
|
|
{
|
|
struct dst_entry *dst = skb_dst(skb);
|
|
struct xfrm_state *x = dst->xfrm;
|
|
struct net *net = xs_net(x);
|
|
|
|
if (err <= 0)
|
|
goto resume;
|
|
|
|
do {
|
|
err = xfrm_skb_check_space(skb);
|
|
if (err) {
|
|
XFRM_INC_STATS(net, LINUX_MIB_XFRMOUTERROR);
|
|
goto error_nolock;
|
|
}
|
|
|
|
err = x->outer_mode->output(x, skb);
|
|
if (err) {
|
|
XFRM_INC_STATS(net, LINUX_MIB_XFRMOUTSTATEMODEERROR);
|
|
goto error_nolock;
|
|
}
|
|
|
|
spin_lock_bh(&x->lock);
|
|
|
|
if (unlikely(x->km.state != XFRM_STATE_VALID)) {
|
|
XFRM_INC_STATS(net, LINUX_MIB_XFRMOUTSTATEINVALID);
|
|
err = -EINVAL;
|
|
goto error;
|
|
}
|
|
|
|
err = xfrm_state_check_expire(x);
|
|
if (err) {
|
|
XFRM_INC_STATS(net, LINUX_MIB_XFRMOUTSTATEEXPIRED);
|
|
goto error;
|
|
}
|
|
|
|
err = x->repl->overflow(x, skb);
|
|
if (err) {
|
|
XFRM_INC_STATS(net, LINUX_MIB_XFRMOUTSTATESEQERROR);
|
|
goto error;
|
|
}
|
|
|
|
x->curlft.bytes += skb->len;
|
|
x->curlft.packets++;
|
|
|
|
spin_unlock_bh(&x->lock);
|
|
|
|
skb_dst_force(skb);
|
|
|
|
err = x->type->output(x, skb);
|
|
if (err == -EINPROGRESS)
|
|
goto out;
|
|
|
|
resume:
|
|
if (err) {
|
|
XFRM_INC_STATS(net, LINUX_MIB_XFRMOUTSTATEPROTOERROR);
|
|
goto error_nolock;
|
|
}
|
|
|
|
dst = skb_dst_pop(skb);
|
|
if (!dst) {
|
|
XFRM_INC_STATS(net, LINUX_MIB_XFRMOUTERROR);
|
|
err = -EHOSTUNREACH;
|
|
goto error_nolock;
|
|
}
|
|
skb_dst_set(skb, dst);
|
|
x = dst->xfrm;
|
|
} while (x && !(x->outer_mode->flags & XFRM_MODE_FLAG_TUNNEL));
|
|
|
|
return 0;
|
|
|
|
error:
|
|
spin_unlock_bh(&x->lock);
|
|
error_nolock:
|
|
kfree_skb(skb);
|
|
out:
|
|
return err;
|
|
}
|
|
|
|
int xfrm_output_resume(struct sk_buff *skb, int err)
|
|
{
|
|
while (likely((err = xfrm_output_one(skb, err)) == 0)) {
|
|
nf_reset(skb);
|
|
|
|
err = skb_dst(skb)->ops->local_out(skb);
|
|
if (unlikely(err != 1))
|
|
goto out;
|
|
|
|
if (!skb_dst(skb)->xfrm)
|
|
return dst_output(skb);
|
|
|
|
err = nf_hook(skb_dst(skb)->ops->family,
|
|
NF_INET_POST_ROUTING, skb,
|
|
NULL, skb_dst(skb)->dev, xfrm_output2);
|
|
if (unlikely(err != 1))
|
|
goto out;
|
|
}
|
|
|
|
if (err == -EINPROGRESS)
|
|
err = 0;
|
|
|
|
out:
|
|
return err;
|
|
}
|
|
EXPORT_SYMBOL_GPL(xfrm_output_resume);
|
|
|
|
static int xfrm_output2(struct sk_buff *skb)
|
|
{
|
|
return xfrm_output_resume(skb, 1);
|
|
}
|
|
|
|
static int xfrm_output_gso(struct sk_buff *skb)
|
|
{
|
|
struct sk_buff *segs;
|
|
|
|
segs = skb_gso_segment(skb, 0);
|
|
kfree_skb(skb);
|
|
if (IS_ERR(segs))
|
|
return PTR_ERR(segs);
|
|
if (segs == NULL)
|
|
return -EINVAL;
|
|
|
|
do {
|
|
struct sk_buff *nskb = segs->next;
|
|
int err;
|
|
|
|
segs->next = NULL;
|
|
err = xfrm_output2(segs);
|
|
|
|
if (unlikely(err)) {
|
|
kfree_skb_list(nskb);
|
|
return err;
|
|
}
|
|
|
|
segs = nskb;
|
|
} while (segs);
|
|
|
|
return 0;
|
|
}
|
|
|
|
int xfrm_output(struct sk_buff *skb)
|
|
{
|
|
struct net *net = dev_net(skb_dst(skb)->dev);
|
|
int err;
|
|
|
|
if (skb_is_gso(skb))
|
|
return xfrm_output_gso(skb);
|
|
|
|
if (skb->ip_summed == CHECKSUM_PARTIAL) {
|
|
err = skb_checksum_help(skb);
|
|
if (err) {
|
|
XFRM_INC_STATS(net, LINUX_MIB_XFRMOUTERROR);
|
|
kfree_skb(skb);
|
|
return err;
|
|
}
|
|
}
|
|
|
|
return xfrm_output2(skb);
|
|
}
|
|
EXPORT_SYMBOL_GPL(xfrm_output);
|
|
|
|
int xfrm_inner_extract_output(struct xfrm_state *x, struct sk_buff *skb)
|
|
{
|
|
struct xfrm_mode *inner_mode;
|
|
if (x->sel.family == AF_UNSPEC)
|
|
inner_mode = xfrm_ip2inner_mode(x,
|
|
xfrm_af2proto(skb_dst(skb)->ops->family));
|
|
else
|
|
inner_mode = x->inner_mode;
|
|
|
|
if (inner_mode == NULL)
|
|
return -EAFNOSUPPORT;
|
|
return inner_mode->afinfo->extract_output(x, skb);
|
|
}
|
|
EXPORT_SYMBOL_GPL(xfrm_inner_extract_output);
|
|
|
|
void xfrm_local_error(struct sk_buff *skb, int mtu)
|
|
{
|
|
unsigned int proto;
|
|
struct xfrm_state_afinfo *afinfo;
|
|
|
|
if (skb->protocol == htons(ETH_P_IP))
|
|
proto = AF_INET;
|
|
else if (skb->protocol == htons(ETH_P_IPV6))
|
|
proto = AF_INET6;
|
|
else
|
|
return;
|
|
|
|
afinfo = xfrm_state_get_afinfo(proto);
|
|
if (!afinfo)
|
|
return;
|
|
|
|
afinfo->local_error(skb, mtu);
|
|
xfrm_state_put_afinfo(afinfo);
|
|
}
|
|
EXPORT_SYMBOL_GPL(xfrm_local_error);
|