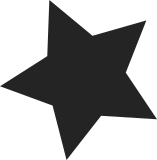
Now that the governor table is in place and the macro allows to browse the table, declare the governor so the entry is added in the governor table in the init section. The [un]register_thermal_governors function does no longer need to use the exported [un]register thermal governor's specific function which in turn call the [un]register_thermal_governor. The governors are fully self-encapsulated. The cyclic dependency is no longer needed, remove it. Reviewed-by: Amit Kucheria <amit.kucheria@linaro.org> Signed-off-by: Daniel Lezcano <daniel.lezcano@linaro.org> Signed-off-by: Zhang Rui <rui.zhang@intel.com>
48 lines
1.4 KiB
C
48 lines
1.4 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* user_space.c - A simple user space Thermal events notifier
|
|
*
|
|
* Copyright (C) 2012 Intel Corp
|
|
* Copyright (C) 2012 Durgadoss R <durgadoss.r@intel.com>
|
|
*
|
|
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
|
|
*
|
|
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
|
|
*/
|
|
|
|
#include <linux/thermal.h>
|
|
#include <linux/slab.h>
|
|
|
|
#include "thermal_core.h"
|
|
|
|
/**
|
|
* notify_user_space - Notifies user space about thermal events
|
|
* @tz - thermal_zone_device
|
|
* @trip - trip point index
|
|
*
|
|
* This function notifies the user space through UEvents.
|
|
*/
|
|
static int notify_user_space(struct thermal_zone_device *tz, int trip)
|
|
{
|
|
char *thermal_prop[5];
|
|
int i;
|
|
|
|
mutex_lock(&tz->lock);
|
|
thermal_prop[0] = kasprintf(GFP_KERNEL, "NAME=%s", tz->type);
|
|
thermal_prop[1] = kasprintf(GFP_KERNEL, "TEMP=%d", tz->temperature);
|
|
thermal_prop[2] = kasprintf(GFP_KERNEL, "TRIP=%d", trip);
|
|
thermal_prop[3] = kasprintf(GFP_KERNEL, "EVENT=%d", tz->notify_event);
|
|
thermal_prop[4] = NULL;
|
|
kobject_uevent_env(&tz->device.kobj, KOBJ_CHANGE, thermal_prop);
|
|
for (i = 0; i < 4; ++i)
|
|
kfree(thermal_prop[i]);
|
|
mutex_unlock(&tz->lock);
|
|
return 0;
|
|
}
|
|
|
|
static struct thermal_governor thermal_gov_user_space = {
|
|
.name = "user_space",
|
|
.throttle = notify_user_space,
|
|
};
|
|
THERMAL_GOVERNOR_DECLARE(thermal_gov_user_space);
|